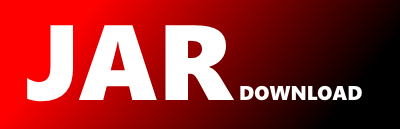
org.freedesktop.gstreamer.gst.AtomicQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gst Show documentation
Show all versions of gst Show documentation
Java language bindings for Gst, generated with Java-GI
/* Java-GI - Java language bindings for GObject-Introspection-based libraries
* Copyright (C) 2022-2023 Jan-Willem Harmannij
*
* SPDX-License-Identifier: LGPL-2.1-or-later
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, see .
*/
/* This file has been generated with Java-GI.
* Do not edit this file directly!
* Visit https://jwharm.github.io/java-gi for more information.
*/
package org.freedesktop.gstreamer.gst;
import io.github.jwharm.javagi.gobject.*;
import io.github.jwharm.javagi.gobject.types.*;
import io.github.jwharm.javagi.base.*;
import io.github.jwharm.javagi.interop.*;
import java.lang.foreign.*;
import java.lang.invoke.*;
import org.jetbrains.annotations.*;
/**
* The {@link AtomicQueue} object implements a queue that can be used from multiple
* threads without performing any blocking operations.
*/
public class AtomicQueue extends ManagedInstance {
static {
Gst.javagi$ensureInitialized();
}
/**
* Get the GType of the GstAtomicQueue class.
* @return the GType
*/
public static org.gnome.glib.Type getType() {
return Interop.getType("gst_atomic_queue_get_type");
}
/**
* Create a AtomicQueue proxy instance for the provided memory address.
* @param address the memory address of the native object
*/
public AtomicQueue(MemorySegment address) {
super(address);
MemoryCleaner.setFreeFunc(this.handle(), "gst_atomic_queue_unref");
}
/**
* Create a new atomic queue instance. {@code initial_size} will be rounded up to the
* nearest power of 2 and used as the initial size of the queue.
* @param initialSize initial queue size
*/
public AtomicQueue(int initialSize) {
super(constructNew(initialSize));
}
/**
* Helper function for the {@code gst_atomic_queue_new} constructor
*/
private static MemorySegment constructNew(int initialSize) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.JAVA_INT);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gst_atomic_queue_new", _fdesc, false).invokeExact(initialSize);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
return _result;
}
/**
* Get the amount of items in the queue.
* @return the number of elements in the queue.
*/
public int length() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gst_atomic_queue_length", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
return _result;
}
/**
* Peek the head element of the queue without removing it from the queue.
* @return the head element of {@code queue} or
* {@code null} when the queue is empty.
*/
public @Nullable java.lang.foreign.MemorySegment peek() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gst_atomic_queue_peek", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
return _result;
}
/**
* Get the head element of the queue.
* @return the head element of {@code queue} or {@code null} when
* the queue is empty.
*/
public @Nullable java.lang.foreign.MemorySegment pop() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gst_atomic_queue_pop", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
return _result;
}
/**
* Append {@code data} to the tail of the queue.
* @param data the data
*/
public void push(@Nullable java.lang.foreign.MemorySegment data) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gst_atomic_queue_push", _fdesc, false).invokeExact(handle(),
(MemorySegment) (data == null ? MemorySegment.NULL : data));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
}
/**
* Increase the refcount of {@code queue}.
*/
public void ref() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gst_atomic_queue_ref", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
}
/**
* Unref {@code queue} and free the memory when the refcount reaches 0.
*/
public void unref() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gst_atomic_queue_unref", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occurred: ", _err);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy