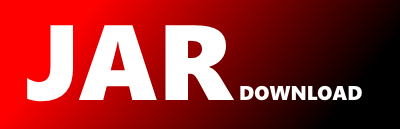
org.gnome.gtk.Accessible Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.Constants;
import io.github.jwharm.javagi.base.Out;
import io.github.jwharm.javagi.base.Proxy;
import io.github.jwharm.javagi.gobject.BuilderInterface;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Overrides;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.Integer;
import java.lang.NullPointerException;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import javax.annotation.processing.Generated;
import org.gnome.glib.GLib;
import org.gnome.glib.LogLevelFlags;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.TypeInstance;
import org.gnome.gobject.TypeInterface;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkAccessible} is an interface for describing UI elements for
* Assistive Technologies.
*
* Every accessible implementation has:
*
* - a “role”, represented by a value of the {@code Gtk.AccessibleRole} enumeration
*
- an “attribute”, represented by a set of {@code Gtk.AccessibleState},
* {@code Gtk.AccessibleProperty} and {@code Gtk.AccessibleRelation} values
*
*
* The role cannot be changed after instantiating a {@code GtkAccessible}
* implementation.
*
* The attributes are updated every time a UI element's state changes in
* a way that should be reflected by assistive technologies. For instance,
* if a {@code GtkWidget} visibility changes, the {@link org.gnome.gtk.AccessibleState#HIDDEN}
* state will also change to reflect the {@code Gtk.Widget:visible} property.
*
* Every accessible implementation is part of a tree of accessible objects.
* Normally, this tree corresponds to the widget tree, but can be customized
* by reimplementing the {@link Accessible#getAccessibleParent},
* {@link Accessible#getFirstAccessibleChild} and
* {@link Accessible#getNextAccessibleSibling} virtual functions.
* Note that you can not create a top-level accessible object as of now,
* which means that you must always have a parent accessible object.
* Also note that when an accessible object does not correspond to a widget,
* and it has children, whose implementation you don't control,
* it is necessary to ensure the correct shape of the a11y tree
* by calling {@link Accessible#setAccessibleParent} and
* updating the sibling by {@link Accessible#updateNextAccessibleSibling}.
*/
@Generated("io.github.jwharm.JavaGI")
public interface Accessible extends Proxy {
/**
* Get the GType of the Accessible class
*
* @return the GType
*/
static Type getType() {
return Interop.getType("gtk_accessible_get_type");
}
/**
* Requests the user's screen reader to announce the given message.
*
* This kind of notification is useful for messages that
* either have only a visual representation or that are not
* exposed visually at all, e.g. a notification about a
* successful operation.
*
* Also, by using this API, you can ensure that the message
* does not interrupts the user's current screen reader output.
*
* @param message the string to announce
* @param priority the priority of the announcement
*/
default void announce(String message, AccessibleAnnouncementPriority priority) {
try (var _arena = Arena.ofConfined()) {
try {
AccessibleMethodHandles.gtk_accessible_announce.invokeExact(handle(),
(MemorySegment) (message == null ? MemorySegment.NULL : Interop.allocateNativeString(message, _arena)),
priority.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Retrieves the accessible parent for an accessible object.
*
* This function returns {@code NULL} for top level widgets.
*
* @return the accessible parent
*/
default Accessible getAccessibleParent() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_accessible_parent",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) AccessibleMethodHandles.gtk_accessible_get_accessible_parent.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (Accessible) InstanceCache.getForType(_result, AccessibleImpl::new, true);
}
/**
* Retrieves the accessible role of an accessible object.
*
* @return the accessible role
*/
default AccessibleRole getAccessibleRole() {
int _result;
try {
_result = (int) AccessibleMethodHandles.gtk_accessible_get_accessible_role.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return AccessibleRole.of(_result);
}
/**
* Retrieves the accessible implementation for the given {@code GtkAccessible}.
*
* @return the accessible implementation object
*/
default ATContext getAtContext() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_at_context",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) AccessibleMethodHandles.gtk_accessible_get_at_context.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (ATContext) InstanceCache.getForType(_result, ATContext.ATContextImpl::new, true);
}
/**
* Queries the coordinates and dimensions of this accessible
*
* This functionality can be overridden by {@code GtkAccessible}
* implementations, e.g. to get the bounds from an ignored
* child widget.
*
* @param x the x coordinate of the top left corner of the accessible
* @param y the y coordinate of the top left corner of the widget
* @param width the width of the accessible object
* @param height the height of the accessible object
* @return true if the bounds are valid, and false otherwise
*/
default boolean getBounds(Out x, Out y, Out width,
Out height) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _xPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _yPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _widthPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _heightPointer = _arena.allocate(ValueLayout.JAVA_INT);
int _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_bounds",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (int) Interop.downcallHandle(_func, _fdesc).invokeExact(handle(),
(MemorySegment) (x == null ? MemorySegment.NULL : _xPointer),
(MemorySegment) (y == null ? MemorySegment.NULL : _yPointer),
(MemorySegment) (width == null ? MemorySegment.NULL : _widthPointer),
(MemorySegment) (height == null ? MemorySegment.NULL : _heightPointer));
} else {
_result = (int) AccessibleMethodHandles.gtk_accessible_get_bounds.invokeExact(
handle(), (MemorySegment) (x == null ? MemorySegment.NULL : _xPointer),
(MemorySegment) (y == null ? MemorySegment.NULL : _yPointer),
(MemorySegment) (width == null ? MemorySegment.NULL : _widthPointer),
(MemorySegment) (height == null ? MemorySegment.NULL : _heightPointer));
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (x != null) {
x.set(_xPointer.get(ValueLayout.JAVA_INT, 0));
}
if (y != null) {
y.set(_yPointer.get(ValueLayout.JAVA_INT, 0));
}
if (width != null) {
width.set(_widthPointer.get(ValueLayout.JAVA_INT, 0));
}
if (height != null) {
height.set(_heightPointer.get(ValueLayout.JAVA_INT, 0));
}
return _result != 0;
}
}
/**
* Retrieves the first accessible child of an accessible object.
*
* @return the first accessible child
*/
default Accessible getFirstAccessibleChild() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_first_accessible_child",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) AccessibleMethodHandles.gtk_accessible_get_first_accessible_child.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (Accessible) InstanceCache.getForType(_result, AccessibleImpl::new, true);
}
/**
* Retrieves the next accessible sibling of an accessible object
*
* @return the next accessible sibling
*/
default Accessible getNextAccessibleSibling() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_next_accessible_sibling",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) AccessibleMethodHandles.gtk_accessible_get_next_accessible_sibling.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (Accessible) InstanceCache.getForType(_result, AccessibleImpl::new, true);
}
/**
* Query a platform state, such as focus.
*
* See gtk_accessible_platform_changed().
*
* This functionality can be overridden by {@code GtkAccessible}
* implementations, e.g. to get platform state from an ignored
* child widget, as is the case for {@code GtkText} wrappers.
*
* @param state platform state to query
* @return the value of {@code state} for the accessible
*/
default boolean getPlatformState(AccessiblePlatformState state) {
int _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
AccessibleInterface.getMemoryLayout(), "get_platform_state",
Accessible.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (int) Interop.downcallHandle(_func, _fdesc).invokeExact(handle(),
state.getValue());
} else {
_result = (int) AccessibleMethodHandles.gtk_accessible_get_platform_state.invokeExact(
handle(), state.getValue());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Resets the accessible {@code property} to its default value.
*
* @param property a {@code GtkAccessibleProperty}
*/
default void resetProperty(AccessibleProperty property) {
try {
AccessibleMethodHandles.gtk_accessible_reset_property.invokeExact(handle(),
property.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Resets the accessible {@code relation} to its default value.
*
* @param relation a {@code GtkAccessibleRelation}
*/
default void resetRelation(AccessibleRelation relation) {
try {
AccessibleMethodHandles.gtk_accessible_reset_relation.invokeExact(handle(),
relation.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Resets the accessible {@code state} to its default value.
*
* @param state a {@code GtkAccessibleState}
*/
default void resetState(AccessibleState state) {
try {
AccessibleMethodHandles.gtk_accessible_reset_state.invokeExact(handle(),
state.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the parent and sibling of an accessible object.
*
* This function is meant to be used by accessible implementations that are
* not part of the widget hierarchy, and but act as a logical bridge between
* widgets. For instance, if a widget creates an object that holds metadata
* for each child, and you want that object to implement the {@code GtkAccessible}
* interface, you will use this function to ensure that the parent of each
* child widget is the metadata object, and the parent of each metadata
* object is the container widget.
*
* @param parent the parent accessible object
* @param nextSibling the sibling accessible object
*/
default void setAccessibleParent(@Nullable Accessible parent,
@Nullable Accessible nextSibling) {
try {
AccessibleMethodHandles.gtk_accessible_set_accessible_parent.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (nextSibling == null ? MemorySegment.NULL : nextSibling.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Updates the next accessible sibling of this Accessible.
*
* That might be useful when a new child of a custom {@code GtkAccessible}
* is created, and it needs to be linked to a previous child.
*
* @param newSibling the new next accessible sibling to set
*/
default void updateNextAccessibleSibling(@Nullable Accessible newSibling) {
try {
AccessibleMethodHandles.gtk_accessible_update_next_accessible_sibling.invokeExact(
handle(),
(MemorySegment) (newSibling == null ? MemorySegment.NULL : newSibling.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Updates an array of accessible properties.
*
* This function should be called by {@code GtkWidget} types whenever an accessible
* property change must be communicated to assistive technologies.
*
* This function is meant to be used by language bindings.
*
* @param properties an array of {@code GtkAccessibleProperty}
* @param values an array of {@code GValues}, one for each property
*/
default void updateProperty(AccessibleProperty[] properties, Value[] values) {
try (var _arena = Arena.ofConfined()) {
int nProperties = properties == null ? 0 : properties.length;
try {
AccessibleMethodHandles.gtk_accessible_update_property_value.invokeExact(handle(),
nProperties,
(MemorySegment) (properties == null ? MemorySegment.NULL : Interop.allocateNativeArray(Interop.getValues(properties), false, _arena)),
(MemorySegment) (values == null ? MemorySegment.NULL : Interop.allocateNativeArray(values, Value.getMemoryLayout(), false, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Updates an array of accessible relations.
*
* This function should be called by {@code GtkWidget} types whenever an accessible
* relation change must be communicated to assistive technologies.
*
* This function is meant to be used by language bindings.
*
* @param relations an array of {@code GtkAccessibleRelation}
* @param values an array of {@code GValues}, one for each relation
*/
default void updateRelation(AccessibleRelation[] relations, Value[] values) {
try (var _arena = Arena.ofConfined()) {
int nRelations = relations == null ? 0 : relations.length;
try {
AccessibleMethodHandles.gtk_accessible_update_relation_value.invokeExact(handle(),
nRelations,
(MemorySegment) (relations == null ? MemorySegment.NULL : Interop.allocateNativeArray(Interop.getValues(relations), false, _arena)),
(MemorySegment) (values == null ? MemorySegment.NULL : Interop.allocateNativeArray(values, Value.getMemoryLayout(), false, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Updates an array of accessible states.
*
* This function should be called by {@code GtkWidget} types whenever an accessible
* state change must be communicated to assistive technologies.
*
* This function is meant to be used by language bindings.
*
* @param states an array of {@code GtkAccessibleState}
* @param values an array of {@code GValues}, one for each state
*/
default void updateState(AccessibleState[] states, Value[] values) {
try (var _arena = Arena.ofConfined()) {
int nStates = states == null ? 0 : states.length;
try {
AccessibleMethodHandles.gtk_accessible_update_state_value.invokeExact(handle(),
nStates,
(MemorySegment) (states == null ? MemorySegment.NULL : Interop.allocateNativeArray(Interop.getValues(states), false, _arena)),
(MemorySegment) (values == null ? MemorySegment.NULL : Interop.allocateNativeArray(values, Value.getMemoryLayout(), false, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* The AccessibleImpl type represents a native instance of the Accessible interface.
*/
class AccessibleImpl extends GObject implements Accessible {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Creates a new instance of Accessible for the provided memory address.
*
* @param address the memory address of the instance
*/
public AccessibleImpl(MemorySegment address) {
super(address);
}
}
/**
* The common interface for accessible objects.
*
* @version 4.10
*/
class AccessibleInterface extends TypeInterface {
private Method _getAtContextMethod;
private Method _getPlatformStateMethod;
private Method _getAccessibleParentMethod;
private Method _getFirstAccessibleChildMethod;
private Method _getNextAccessibleSiblingMethod;
private Method _getBoundsMethod;
/**
* Create a AccessibleInterface proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public AccessibleInterface(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new AccessibleInterface.
*
* @param arena to control the memory allocation scope
*/
public AccessibleInterface(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new AccessibleInterface.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public AccessibleInterface() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
TypeInterface.getMemoryLayout().withName("g_iface"),
ValueLayout.ADDRESS.withName("get_at_context"),
ValueLayout.ADDRESS.withName("get_platform_state"),
ValueLayout.ADDRESS.withName("get_accessible_parent"),
ValueLayout.ADDRESS.withName("get_first_accessible_child"),
ValueLayout.ADDRESS.withName("get_next_accessible_sibling"),
ValueLayout.ADDRESS.withName("get_bounds")
).withName("GtkAccessibleInterface");
}
/**
* Override virtual method {@code get_at_context}.
*
* @param method the method to invoke
*/
public void overrideGetAtContext(Arena arena, Method method) {
this._getAtContextMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getAtContextUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_at_context"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getAtContextUpcall(MemorySegment self) {
try {
Arena _arena = Arena.ofAuto();
var _result = (ATContext) this._getAtContextMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false));
if (_result == null) return MemorySegment.NULL;
if (_result instanceof GObject _gobject) _gobject.ref();
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getAtContextMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_platform_state}.
*
* @param method the method to invoke
*/
public void overrideGetPlatformState(Arena arena, Method method) {
this._getPlatformStateMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getPlatformStateUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_platform_state"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private int getPlatformStateUpcall(MemorySegment self, int state) {
try {
Arena _arena = Arena.ofAuto();
var _result = (boolean) this._getPlatformStateMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false), AccessiblePlatformState.of(state));
return _result ? 1 : 0;
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getPlatformStateMethod);
return 0;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_accessible_parent}.
*
* @param method the method to invoke
*/
public void overrideGetAccessibleParent(Arena arena, Method method) {
this._getAccessibleParentMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getAccessibleParentUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_accessible_parent"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getAccessibleParentUpcall(MemorySegment self) {
try {
Arena _arena = Arena.ofAuto();
var _result = (Accessible) this._getAccessibleParentMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false));
if (_result == null) return MemorySegment.NULL;
if (_result instanceof GObject _gobject) _gobject.ref();
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getAccessibleParentMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_first_accessible_child}.
*
* @param method the method to invoke
*/
public void overrideGetFirstAccessibleChild(Arena arena, Method method) {
this._getFirstAccessibleChildMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getFirstAccessibleChildUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_first_accessible_child"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getFirstAccessibleChildUpcall(MemorySegment self) {
try {
Arena _arena = Arena.ofAuto();
var _result = (Accessible) this._getFirstAccessibleChildMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false));
if (_result == null) return MemorySegment.NULL;
if (_result instanceof GObject _gobject) _gobject.ref();
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getFirstAccessibleChildMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_next_accessible_sibling}.
*
* @param method the method to invoke
*/
public void overrideGetNextAccessibleSibling(Arena arena, Method method) {
this._getNextAccessibleSiblingMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getNextAccessibleSiblingUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_next_accessible_sibling"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getNextAccessibleSiblingUpcall(MemorySegment self) {
try {
Arena _arena = Arena.ofAuto();
var _result = (Accessible) this._getNextAccessibleSiblingMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false));
if (_result == null) return MemorySegment.NULL;
if (_result instanceof GObject _gobject) _gobject.ref();
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getNextAccessibleSiblingMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_bounds}.
*
* @param method the method to invoke
*/
public void overrideGetBounds(Arena arena, Method method) {
this._getBoundsMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), AccessibleInterface.class, "getBoundsUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_bounds"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private int getBoundsUpcall(MemorySegment self, MemorySegment x, MemorySegment y,
MemorySegment width, MemorySegment height) {
try {
Arena _arena = Arena.ofAuto();
MemorySegment xParam = x.reinterpret(ValueLayout.JAVA_INT.byteSize(), _arena, null);
Out _xOut = new Out<>(xParam.get(ValueLayout.JAVA_INT, 0));
MemorySegment yParam = y.reinterpret(ValueLayout.JAVA_INT.byteSize(), _arena, null);
Out _yOut = new Out<>(yParam.get(ValueLayout.JAVA_INT, 0));
MemorySegment widthParam = width.reinterpret(ValueLayout.JAVA_INT.byteSize(), _arena, null);
Out _widthOut = new Out<>(widthParam.get(ValueLayout.JAVA_INT, 0));
MemorySegment heightParam = height.reinterpret(ValueLayout.JAVA_INT.byteSize(), _arena, null);
Out _heightOut = new Out<>(heightParam.get(ValueLayout.JAVA_INT, 0));
var _result = (boolean) this._getBoundsMethod.invoke((Accessible) InstanceCache.getForType(self, AccessibleImpl::new, false), _xOut, _yOut, _widthOut, _heightOut);
xParam.set(ValueLayout.JAVA_INT, 0, _xOut.get());
yParam.set(ValueLayout.JAVA_INT, 0, _yOut.get());
widthParam.set(ValueLayout.JAVA_INT, 0, _widthOut.get());
heightParam.set(ValueLayout.JAVA_INT, 0, _heightOut.get());
return _result ? 1 : 0;
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getBoundsMethod);
return 0;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
interface Builder> extends BuilderInterface {
/**
* The accessible role of the given {@code GtkAccessible} implementation.
*
* The accessible role cannot be changed once set.
*
* @param accessibleRole the value for the {@code accessible-role} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
default B setAccessibleRole(AccessibleRole accessibleRole) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(AccessibleRole.getType());
_value.setEnum(accessibleRole.getValue());
addBuilderProperty("accessible-role", _value);
return (B) this;
}
}
}