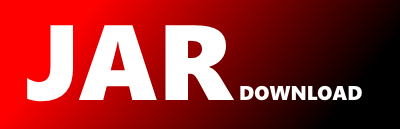
org.gnome.gtk.AspectFrame Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkAspectFrame} preserves the aspect ratio of its child.
*
* The frame can respect the aspect ratio of the child widget,
* or use its own aspect ratio.
*
* CSS nodes
* {@code GtkAspectFrame} uses a CSS node with name {@code aspectframe}.
*
* Accessibility
* Until GTK 4.10, {@code GtkAspectFrame} used the {@code GTK_ACCESSIBLE_ROLE_GROUP} role.
*
* Starting from GTK 4.12, {@code GtkAspectFrame} uses the {@code GTK_ACCESSIBLE_ROLE_GENERIC} role.
*/
@Generated("io.github.jwharm.JavaGI")
public class AspectFrame extends Widget implements Accessible, Buildable, ConstraintTarget {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a AspectFrame proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public AspectFrame(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Create a new {@code GtkAspectFrame}.
*
* @param xalign Horizontal alignment of the child within the parent.
* Ranges from 0.0 (left aligned) to 1.0 (right aligned)
* @param yalign Vertical alignment of the child within the parent.
* Ranges from 0.0 (top aligned) to 1.0 (bottom aligned)
* @param ratio The desired aspect ratio.
* @param obeyChild If {@code true}, {@code ratio} is ignored, and the aspect
* ratio is taken from the requistion of the child.
*/
public AspectFrame(float xalign, float yalign, float ratio, boolean obeyChild) {
this(constructNew(xalign, yalign, ratio, obeyChild));
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the AspectFrame class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_aspect_frame_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected AspectFrame asParent() {
AspectFrame _parent = new AspectFrame(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew(float xalign, float yalign, float ratio,
boolean obeyChild) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_aspect_frame_new.invokeExact(xalign,
yalign, ratio, obeyChild ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the child widget of this AspectFrame.
*
* @return the child widget of this AspectFrame
*/
public Widget getChild() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_aspect_frame_get_child.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Widget %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Returns whether the child's size request should override
* the set aspect ratio of the {@code GtkAspectFrame}.
*
* @return whether to obey the child's size request
*/
public boolean getObeyChild() {
int _result;
try {
_result = (int) MethodHandles.gtk_aspect_frame_get_obey_child.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Returns the desired aspect ratio of the child.
*
* @return the desired aspect ratio
*/
public float getRatio() {
float _result;
try {
_result = (float) MethodHandles.gtk_aspect_frame_get_ratio.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Returns the horizontal alignment of the child within the
* allocation of the {@code GtkAspectFrame}.
*
* @return the horizontal alignment
*/
public float getXalign() {
float _result;
try {
_result = (float) MethodHandles.gtk_aspect_frame_get_xalign.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Returns the vertical alignment of the child within the
* allocation of the {@code GtkAspectFrame}.
*
* @return the vertical alignment
*/
public float getYalign() {
float _result;
try {
_result = (float) MethodHandles.gtk_aspect_frame_get_yalign.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Sets the child widget of this AspectFrame.
*
* @param child the child widget
*/
public void setChild(@Nullable Widget child) {
try {
MethodHandles.gtk_aspect_frame_set_child.invokeExact(handle(),
(MemorySegment) (child == null ? MemorySegment.NULL : child.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets whether the aspect ratio of the child's size
* request should override the set aspect ratio of
* the {@code GtkAspectFrame}.
*
* @param obeyChild If {@code true}, {@code ratio} is ignored, and the aspect
* ratio is taken from the requisition of the child.
*/
public void setObeyChild(boolean obeyChild) {
try {
MethodHandles.gtk_aspect_frame_set_obey_child.invokeExact(handle(), obeyChild ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the desired aspect ratio of the child.
*
* @param ratio aspect ratio of the child
*/
public void setRatio(float ratio) {
try {
MethodHandles.gtk_aspect_frame_set_ratio.invokeExact(handle(), ratio);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the horizontal alignment of the child within the allocation
* of the {@code GtkAspectFrame}.
*
* @param xalign horizontal alignment, from 0.0 (left aligned) to 1.0 (right aligned)
*/
public void setXalign(float xalign) {
try {
MethodHandles.gtk_aspect_frame_set_xalign.invokeExact(handle(), xalign);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the vertical alignment of the child within the allocation
* of the {@code GtkAspectFrame}.
*
* @param yalign horizontal alignment, from 0.0 (top aligned) to 1.0 (bottom aligned)
*/
public void setYalign(float yalign) {
try {
MethodHandles.gtk_aspect_frame_set_yalign.invokeExact(handle(), yalign);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code AspectFrame}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends Widget.Builder implements Accessible.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code AspectFrame} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code AspectFrame}.
*
* @return a new instance of {@code AspectFrame} with the properties
* that were set in the Builder object.
*/
public AspectFrame build() {
try {
var _instance = (AspectFrame) GObject.withProperties(AspectFrame.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The child widget.
*
* @param child the value for the {@code child} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setChild(Widget child) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Widget.getType());
_value.setObject((GObject) child);
addBuilderProperty("child", _value);
return (B) this;
}
/**
* Whether the {@code GtkAspectFrame} should use the aspect ratio of its child.
*
* @param obeyChild the value for the {@code obey-child} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setObeyChild(boolean obeyChild) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(obeyChild);
addBuilderProperty("obey-child", _value);
return (B) this;
}
/**
* The aspect ratio to be used by the {@code GtkAspectFrame}.
*
* This property is only used if
* {@code Gtk.AspectFrame:obey-child} is set to {@code false}.
*
* @param ratio the value for the {@code ratio} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setRatio(float ratio) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.FLOAT);
_value.setFloat(ratio);
addBuilderProperty("ratio", _value);
return (B) this;
}
/**
* The horizontal alignment of the child.
*
* @param xalign the value for the {@code xalign} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setXalign(float xalign) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.FLOAT);
_value.setFloat(xalign);
addBuilderProperty("xalign", _value);
return (B) this;
}
/**
* The vertical alignment of the child.
*
* @param yalign the value for the {@code yalign} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setYalign(float yalign) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.FLOAT);
_value.setFloat(yalign);
addBuilderProperty("yalign", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_aspect_frame_new = Interop.downcallHandle(
"gtk_aspect_frame_new", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.JAVA_FLOAT, ValueLayout.JAVA_FLOAT, ValueLayout.JAVA_FLOAT,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_aspect_frame_get_child = Interop.downcallHandle(
"gtk_aspect_frame_get_child", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_get_obey_child = Interop.downcallHandle(
"gtk_aspect_frame_get_obey_child", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_get_ratio = Interop.downcallHandle(
"gtk_aspect_frame_get_ratio", FunctionDescriptor.of(ValueLayout.JAVA_FLOAT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_get_xalign = Interop.downcallHandle(
"gtk_aspect_frame_get_xalign", FunctionDescriptor.of(ValueLayout.JAVA_FLOAT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_get_yalign = Interop.downcallHandle(
"gtk_aspect_frame_get_yalign", FunctionDescriptor.of(ValueLayout.JAVA_FLOAT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_set_child = Interop.downcallHandle(
"gtk_aspect_frame_set_child", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_aspect_frame_set_obey_child = Interop.downcallHandle(
"gtk_aspect_frame_set_obey_child", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_aspect_frame_set_ratio = Interop.downcallHandle(
"gtk_aspect_frame_set_ratio", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_FLOAT), false);
static final MethodHandle gtk_aspect_frame_set_xalign = Interop.downcallHandle(
"gtk_aspect_frame_set_xalign", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_FLOAT), false);
static final MethodHandle gtk_aspect_frame_set_yalign = Interop.downcallHandle(
"gtk_aspect_frame_set_yalign", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_FLOAT), false);
}
}