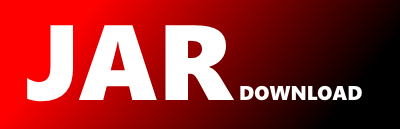
org.gnome.gtk.BookmarkList Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.gio.ListModel;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkBookmarkList} is a list model that wraps {@code GBookmarkFile}.
*
* It presents a {@code GListModel} and fills it asynchronously with the
* {@code GFileInfo}s returned from that function.
*
* The {@code GFileInfo}s in the list have some attributes in the recent
* namespace added: {@code recent::private} (boolean) and {@code recent:applications}
* (stringv).
*/
@Generated("io.github.jwharm.JavaGI")
public class BookmarkList extends GObject implements ListModel {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a BookmarkList proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public BookmarkList(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkBookmarkList} with the given {@code attributes}.
*
* @param filename The bookmark file to load
* @param attributes The attributes to query
*/
public BookmarkList(@Nullable String filename, @Nullable String attributes) {
this(constructNew(filename, attributes));
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the BookmarkList class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_bookmark_list_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected BookmarkList asParent() {
BookmarkList _parent = new BookmarkList(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew(@Nullable String filename,
@Nullable String attributes) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_bookmark_list_new.invokeExact(
(MemorySegment) (filename == null ? MemorySegment.NULL : Interop.allocateNativeString(filename, _arena)),
(MemorySegment) (attributes == null ? MemorySegment.NULL : Interop.allocateNativeString(attributes, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Gets the attributes queried on the children.
*
* @return The queried attributes
*/
public String getAttributes() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_bookmark_list_get_attributes.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Returns the filename of the bookmark file that
* this list is loading.
*
* @return the filename of the .xbel file
*/
public String getFilename() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_bookmark_list_get_filename.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the IO priority to use while loading file.
*
* @return The IO priority.
*/
public int getIoPriority() {
int _result;
try {
_result = (int) MethodHandles.gtk_bookmark_list_get_io_priority.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Returns {@code true} if the files are currently being loaded.
*
* Files will be added to this BookmarkList from time to time while loading is
* going on. The order in which are added is undefined and may change
* in between runs.
*
* @return {@code true} if this BookmarkList is loading
*/
public boolean isLoading() {
int _result;
try {
_result = (int) MethodHandles.gtk_bookmark_list_is_loading.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Sets the {@code attributes} to be enumerated and starts the enumeration.
*
* If {@code attributes} is {@code null}, no attributes will be queried, but a list
* of {@code GFileInfo}s will still be created.
*
* @param attributes the attributes to enumerate
*/
public void setAttributes(@Nullable String attributes) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_bookmark_list_set_attributes.invokeExact(handle(),
(MemorySegment) (attributes == null ? MemorySegment.NULL : Interop.allocateNativeString(attributes, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the IO priority to use while loading files.
*
* The default IO priority is {@code G_PRIORITY_DEFAULT}.
*
* @param ioPriority IO priority to use
*/
public void setIoPriority(int ioPriority) {
try {
MethodHandles.gtk_bookmark_list_set_io_priority.invokeExact(handle(), ioPriority);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code BookmarkList}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class BookmarkListClass extends GObject.ObjectClass {
/**
* Create a BookmarkListClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public BookmarkListClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new BookmarkListClass.
*
* @param arena to control the memory allocation scope
*/
public BookmarkListClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new BookmarkListClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public BookmarkListClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
GObject.ObjectClass.getMemoryLayout().withName("parent_class")
).withName("GtkBookmarkListClass");
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code BookmarkList} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code BookmarkList}.
*
* @return a new instance of {@code BookmarkList} with the properties
* that were set in the Builder object.
*/
public BookmarkList build() {
try {
var _instance = (BookmarkList) GObject.withProperties(BookmarkList.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The attributes to query.
*
* @param attributes the value for the {@code attributes} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAttributes(String attributes) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(attributes);
addBuilderProperty("attributes", _value);
return (B) this;
}
/**
* The bookmark file to load.
*
* @param filename the value for the {@code filename} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFilename(String filename) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(filename);
addBuilderProperty("filename", _value);
return (B) this;
}
/**
* Priority used when loading.
*
* @param ioPriority the value for the {@code io-priority} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setIoPriority(int ioPriority) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.INT);
_value.setInt(ioPriority);
addBuilderProperty("io-priority", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_bookmark_list_new = Interop.downcallHandle(
"gtk_bookmark_list_new", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_get_attributes = Interop.downcallHandle(
"gtk_bookmark_list_get_attributes", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_get_filename = Interop.downcallHandle(
"gtk_bookmark_list_get_filename", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_get_io_priority = Interop.downcallHandle(
"gtk_bookmark_list_get_io_priority", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_is_loading = Interop.downcallHandle(
"gtk_bookmark_list_is_loading", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_set_attributes = Interop.downcallHandle(
"gtk_bookmark_list_set_attributes", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_bookmark_list_set_io_priority = Interop.downcallHandle(
"gtk_bookmark_list_set_io_priority", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
}
}