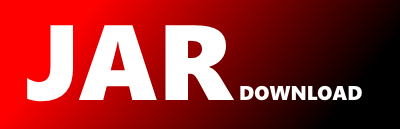
org.gnome.gtk.BuildableParseContext Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.Out;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.Integer;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.PtrArray;
import org.jetbrains.annotations.Nullable;
/**
* An opaque context struct for {@code GtkBuildableParser}.
*/
@Generated("io.github.jwharm.JavaGI")
public class BuildableParseContext extends ProxyInstance {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a BuildableParseContext proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public BuildableParseContext(MemorySegment address) {
super(address);
}
/**
* Retrieves the name of the currently open element.
*
* If called from the start_element or end_element handlers this will
* give the element_name as passed to those functions. For the parent
* elements, see gtk_buildable_parse_context_get_element_stack().
*
* @return the name of the currently open element
*/
public String getElement() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_buildable_parse_context_get_element.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Retrieves the element stack from the internal state of the parser.
*
* The returned {@code GPtrArray} is an array of strings where the last item is
* the currently open tag (as would be returned by
* gtk_buildable_parse_context_get_element()) and the previous item is its
* immediate parent.
*
* This function is intended to be used in the start_element and
* end_element handlers where gtk_buildable_parse_context_get_element()
* would merely return the name of the element that is being
* processed.
*
* @return the element stack, which must not be modified
*/
public String[] getElementStack() {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_buildable_parse_context_get_element_stack.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return io.github.jwharm.javagi.interop.Interop.getStringArrayFrom(Interop.dereference(_result), new PtrArray(_result).readLen(), false);
}
}
/**
* Retrieves the current line number and the number of the character on
* that line. Intended for use in error messages; there are no strict
* semantics for what constitutes the "current" line number other than
* "the best number we could come up with for error messages."
*
* @param lineNumber return location for a line number
* @param charNumber return location for a char-on-line number
*/
public void getPosition(@Nullable Out lineNumber, @Nullable Out charNumber) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _lineNumberPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _charNumberPointer = _arena.allocate(ValueLayout.JAVA_INT);
try {
MethodHandles.gtk_buildable_parse_context_get_position.invokeExact(handle(),
(MemorySegment) (lineNumber == null ? MemorySegment.NULL : _lineNumberPointer),
(MemorySegment) (charNumber == null ? MemorySegment.NULL : _charNumberPointer));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (lineNumber != null) {
lineNumber.set(_lineNumberPointer.get(ValueLayout.JAVA_INT, 0));
}
if (charNumber != null) {
charNumber.set(_charNumberPointer.get(ValueLayout.JAVA_INT, 0));
}
}
}
/**
* Completes the process of a temporary sub-parser redirection.
*
* This function exists to collect the user_data allocated by a
* matching call to gtk_buildable_parse_context_push(). It must be called
* in the end_element handler corresponding to the start_element
* handler during which gtk_buildable_parse_context_push() was called.
* You must not call this function from the error callback -- the
* {@code userData} is provided directly to the callback in that case.
*
* This function is not intended to be directly called by users
* interested in invoking subparsers. Instead, it is intended to
* be used by the subparsers themselves to implement a higher-level
* interface.
*
* @return the user data passed to gtk_buildable_parse_context_push()
*/
public MemorySegment pop() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_buildable_parse_context_pop.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Temporarily redirects markup data to a sub-parser.
*
* This function may only be called from the start_element handler of
* a {@code GtkBuildableParser}. It must be matched with a corresponding call to
* gtk_buildable_parse_context_pop() in the matching end_element handler
* (except in the case that the parser aborts due to an error).
*
* All tags, text and other data between the matching tags is
* redirected to the subparser given by {@code parser}. {@code userData} is used
* as the user_data for that parser. {@code userData} is also passed to the
* error callback in the event that an error occurs. This includes
* errors that occur in subparsers of the subparser.
*
* The end tag matching the start tag for which this call was made is
* handled by the previous parser (which is given its own user_data)
* which is why gtk_buildable_parse_context_pop() is provided to allow "one
* last access" to the {@code userData} provided to this function. In the
* case of error, the {@code userData} provided here is passed directly to
* the error callback of the subparser and gtk_buildable_parse_context_pop()
* should not be called. In either case, if {@code userData} was allocated
* then it ought to be freed from both of these locations.
*
* This function is not intended to be directly called by users
* interested in invoking subparsers. Instead, it is intended to be
* used by the subparsers themselves to implement a higher-level
* interface.
*
* For an example of how to use this, see g_markup_parse_context_push() which
* has the same kind of API.
*
* @param parser a {@code GtkBuildableParser}
* @param userData user data to pass to {@code GtkBuildableParser} functions
*/
public void push(BuildableParser parser, @Nullable MemorySegment userData) {
try {
MethodHandles.gtk_buildable_parse_context_push.invokeExact(handle(),
(MemorySegment) (parser == null ? MemorySegment.NULL : parser.handle()),
(MemorySegment) (userData == null ? MemorySegment.NULL : userData));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
private static final class MethodHandles {
static final MethodHandle gtk_buildable_parse_context_get_element = Interop.downcallHandle(
"gtk_buildable_parse_context_get_element",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_buildable_parse_context_get_element_stack = Interop.downcallHandle(
"gtk_buildable_parse_context_get_element_stack",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_buildable_parse_context_get_position = Interop.downcallHandle(
"gtk_buildable_parse_context_get_position",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_buildable_parse_context_pop = Interop.downcallHandle(
"gtk_buildable_parse_context_pop", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_buildable_parse_context_push = Interop.downcallHandle(
"gtk_buildable_parse_context_push", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
}
}