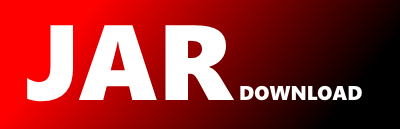
org.gnome.gtk.EventControllerKey Maven / Gradle / Ivy
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.FunctionPointer;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.SignalConnection;
import io.github.jwharm.javagi.gobject.types.Signals;
import io.github.jwharm.javagi.interop.Arenas;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.FunctionalInterface;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.gnome.gdk.ModifierType;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.TypeClass;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkEventControllerKey} is an event controller that provides access
* to key events.
*/
@Generated("io.github.jwharm.JavaGI")
public class EventControllerKey extends EventController {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a EventControllerKey proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public EventControllerKey(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new event controller that will handle key events.
*/
public EventControllerKey() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the EventControllerKey class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_event_controller_key_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected EventControllerKey asParent() {
EventControllerKey _parent = new EventControllerKey(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_event_controller_key_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Forwards the current event of this this EventControllerKey to a {@code widget}.
*
* This function can only be used in handlers for the
* {@code Gtk.EventControllerKey::key-pressed},
* {@code Gtk.EventControllerKey::key-released}
* or {@code Gtk.EventControllerKey::modifiers} signals.
*
* @param widget a {@code GtkWidget}
* @return whether the {@code widget} handled the event
*/
public boolean forward(Widget widget) {
int _result;
try {
_result = (int) MethodHandles.gtk_event_controller_key_forward.invokeExact(handle(),
(MemorySegment) (widget == null ? MemorySegment.NULL : widget.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Gets the key group of the current event of this this EventControllerKey.
*
* See {@link org.gnome.gdk.KeyEvent#getLayout}.
*
* @return the key group
*/
public int getGroup() {
int _result;
try {
_result = (int) MethodHandles.gtk_event_controller_key_get_group.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the input method context of the key this EventControllerKey.
*
* @return the {@code GtkIMContext}
*/
public IMContext getImContext() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_event_controller_key_get_im_context.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (IMContext) InstanceCache.getForType(_result, IMContext.IMContextImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.IMContext %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Sets the input method context of the key this EventControllerKey.
*
* @param imContext a {@code GtkIMContext}
*/
public void setImContext(@Nullable IMContext imContext) {
try {
MethodHandles.gtk_event_controller_key_set_im_context.invokeExact(handle(),
(MemorySegment) (imContext == null ? MemorySegment.NULL : imContext.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted whenever the input method context filters away
* a keypress and prevents the {@code controller} receiving it.
*
* See {@link EventControllerKey#setImContext} and
* {@link IMContext#filterKeypress}.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see ImUpdateCallback#run
*/
public SignalConnection onImUpdate(ImUpdateCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("im-update", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "im-update" signal. See {@link #onImUpdate}.
*/
public void emitImUpdate() {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("im-update", _arena);
Object[] _args = new Object[0];
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted whenever a key is pressed.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see KeyPressedCallback#run
*/
public SignalConnection onKeyPressed(KeyPressedCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("key-pressed", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "key-pressed" signal. See {@link #onKeyPressed}.
*/
public boolean emitKeyPressed(int keyval, int keycode, Set state) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _result = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _name = Interop.allocateNativeString("key-pressed", _arena);
Object[] _args = new Object[] {keyval, keycode, Interop.enumSetToInt(state), _result};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
return _result.get(ValueLayout.JAVA_INT, 0) != 0;
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted whenever a key is released.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see KeyReleasedCallback#run
*/
public SignalConnection onKeyReleased(KeyReleasedCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("key-released", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "key-released" signal. See {@link #onKeyReleased}.
*/
public void emitKeyReleased(int keyval, int keycode, Set state) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("key-released", _arena);
Object[] _args = new Object[] {keyval, keycode, Interop.enumSetToInt(state)};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted whenever the state of modifier keys and pointer buttons change.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see ModifiersCallback#run
*/
public SignalConnection onModifiers(ModifiersCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("modifiers", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "modifiers" signal. See {@link #onModifiers}.
*/
public boolean emitModifiers(Set state) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _result = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _name = Interop.allocateNativeString("modifiers", _arena);
Object[] _args = new Object[] {Interop.enumSetToInt(state), _result};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
return _result.get(ValueLayout.JAVA_INT, 0) != 0;
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code EventControllerKey}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Functional interface declaration of the {@code ImUpdateCallback} callback.
*
* @see ImUpdateCallback#run
*/
@FunctionalInterface
public interface ImUpdateCallback extends FunctionPointer {
/**
* Emitted whenever the input method context filters away
* a keypress and prevents the {@code controller} receiving it.
*
* See {@link EventControllerKey#setImContext} and
* {@link IMContext#filterKeypress}.
*/
void run();
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceEventControllerKey) {
run();
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), ImUpdateCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Functional interface declaration of the {@code KeyPressedCallback} callback.
*
* @see KeyPressedCallback#run
*/
@FunctionalInterface
public interface KeyPressedCallback extends FunctionPointer {
/**
* Emitted whenever a key is pressed.
*/
boolean run(int keyval, int keycode, Set state);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default int upcall(MemorySegment sourceEventControllerKey, int keyval, int keycode,
int state) {
var _result = run(keyval, keycode, Interop.intToEnumSet(ModifierType.class, ModifierType::of, state));
return _result ? 1 : 0;
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.JAVA_INT,
ValueLayout.JAVA_INT);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), KeyPressedCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Functional interface declaration of the {@code KeyReleasedCallback} callback.
*
* @see KeyReleasedCallback#run
*/
@FunctionalInterface
public interface KeyReleasedCallback extends FunctionPointer {
/**
* Emitted whenever a key is released.
*/
void run(int keyval, int keycode, Set state);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceEventControllerKey, int keyval, int keycode,
int state) {
run(keyval, keycode, Interop.intToEnumSet(ModifierType.class, ModifierType::of, state));
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT, ValueLayout.JAVA_INT, ValueLayout.JAVA_INT);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), KeyReleasedCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Functional interface declaration of the {@code ModifiersCallback} callback.
*
* @see ModifiersCallback#run
*/
@FunctionalInterface
public interface ModifiersCallback extends FunctionPointer {
/**
* Emitted whenever the state of modifier keys and pointer buttons change.
*/
boolean run(Set state);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default int upcall(MemorySegment sourceEventControllerKey, int state) {
var _result = run(Interop.intToEnumSet(ModifierType.class, ModifierType::of, state));
return _result ? 1 : 0;
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), ModifiersCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
public static class EventControllerKeyClass extends TypeClass {
/**
* Create a EventControllerKeyClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public EventControllerKeyClass(MemorySegment address) {
super(address);
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends EventController.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code EventControllerKey} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code EventControllerKey}.
*
* @return a new instance of {@code EventControllerKey} with the properties
* that were set in the Builder object.
*/
public EventControllerKey build() {
try {
var _instance = (EventControllerKey) GObject.withProperties(EventControllerKey.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* Emitted whenever the input method context filters away
* a keypress and prevents the {@code controller} receiving it.
*
* See {@link EventControllerKey#setImContext} and
* {@link IMContext#filterKeypress}.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see ImUpdateCallback#run
*/
public B onImUpdate(ImUpdateCallback handler) {
connect("im-update", handler);
return (B) this;
}
/**
* Emitted whenever a key is pressed.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see KeyPressedCallback#run
*/
public B onKeyPressed(KeyPressedCallback handler) {
connect("key-pressed", handler);
return (B) this;
}
/**
* Emitted whenever a key is released.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see KeyReleasedCallback#run
*/
public B onKeyReleased(KeyReleasedCallback handler) {
connect("key-released", handler);
return (B) this;
}
/**
* Emitted whenever the state of modifier keys and pointer buttons change.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see ModifiersCallback#run
*/
public B onModifiers(ModifiersCallback handler) {
connect("modifiers", handler);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_event_controller_key_new = Interop.downcallHandle(
"gtk_event_controller_key_new", FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_event_controller_key_forward = Interop.downcallHandle(
"gtk_event_controller_key_forward", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_event_controller_key_get_group = Interop.downcallHandle(
"gtk_event_controller_key_get_group", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_event_controller_key_get_im_context = Interop.downcallHandle(
"gtk_event_controller_key_get_im_context",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_event_controller_key_set_im_context = Interop.downcallHandle(
"gtk_event_controller_key_set_im_context",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
}
}