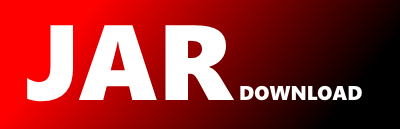
org.gnome.gtk.FileChooserNative Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.Deprecated;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkFileChooserNative} is an abstraction of a dialog suitable
* for use with “File Open” or “File Save as” commands.
*
* By default, this just uses a {@code GtkFileChooserDialog} to implement
* the actual dialog. However, on some platforms, such as Windows and
* macOS, the native platform file chooser is used instead. When the
* application is running in a sandboxed environment without direct
* filesystem access (such as Flatpak), {@code GtkFileChooserNative} may call
* the proper APIs (portals) to let the user choose a file and make it
* available to the application.
*
* While the API of {@code GtkFileChooserNative} closely mirrors {@code GtkFileChooserDialog},
* the main difference is that there is no access to any {@code GtkWindow} or {@code GtkWidget}
* for the dialog. This is required, as there may not be one in the case of a
* platform native dialog.
*
* Showing, hiding and running the dialog is handled by the
* {@link NativeDialog} functions.
*
* Note that unlike {@code GtkFileChooserDialog}, {@code GtkFileChooserNative} objects
* are not toplevel widgets, and GTK does not keep them alive. It is your
* responsibility to keep a reference until you are done with the
* object.
*
* Typical usage
* In the simplest of cases, you can the following code to use
* {@code GtkFileChooserNative} to select a file for opening:
*
{@code static void
* on_response (GtkNativeDialog *native,
* int response)
* {
* if (response == GTK_RESPONSE_ACCEPT)
* {
* GtkFileChooser *chooser = GTK_FILE_CHOOSER (native);
* GFile *file = gtk_file_chooser_get_file (chooser);
*
* open_file (file);
*
* g_object_unref (file);
* }
*
* g_object_unref (native);
* }
*
* // ...
* GtkFileChooserNative *native;
* GtkFileChooserAction action = GTK_FILE_CHOOSER_ACTION_OPEN;
*
* native = gtk_file_chooser_native_new ("Open File",
* parent_window,
* action,
* "_Open",
* "_Cancel");
*
* g_signal_connect (native, "response", G_CALLBACK (on_response), NULL);
* gtk_native_dialog_show (GTK_NATIVE_DIALOG (native));
* }
*
* To use a {@code GtkFileChooserNative} for saving, you can use this:
*
{@code static void
* on_response (GtkNativeDialog *native,
* int response)
* {
* if (response == GTK_RESPONSE_ACCEPT)
* {
* GtkFileChooser *chooser = GTK_FILE_CHOOSER (native);
* GFile *file = gtk_file_chooser_get_file (chooser);
*
* save_to_file (file);
*
* g_object_unref (file);
* }
*
* g_object_unref (native);
* }
*
* // ...
* GtkFileChooserNative *native;
* GtkFileChooser *chooser;
* GtkFileChooserAction action = GTK_FILE_CHOOSER_ACTION_SAVE;
*
* native = gtk_file_chooser_native_new ("Save File",
* parent_window,
* action,
* "_Save",
* "_Cancel");
* chooser = GTK_FILE_CHOOSER (native);
*
* if (user_edited_a_new_document)
* gtk_file_chooser_set_current_name (chooser, _("Untitled document"));
* else
* gtk_file_chooser_set_file (chooser, existing_file, NULL);
*
* g_signal_connect (native, "response", G_CALLBACK (on_response), NULL);
* gtk_native_dialog_show (GTK_NATIVE_DIALOG (native));
* }
*
* For more information on how to best set up a file dialog,
* see the {@link FileChooserDialog} documentation.
*
* Response Codes
* {@code GtkFileChooserNative} inherits from {@link NativeDialog},
* which means it will return {@link org.gnome.gtk.ResponseType#ACCEPT} if the user accepted,
* and {@link org.gnome.gtk.ResponseType#CANCEL} if he pressed cancel. It can also return
* {@link org.gnome.gtk.ResponseType#DELETE_EVENT} if the window was unexpectedly closed.
*
* Differences from {@code GtkFileChooserDialog}
* There are a few things in the {@link FileChooser} interface that
* are not possible to use with {@code GtkFileChooserNative}, as such use would
* prohibit the use of a native dialog.
*
* No operations that change the dialog work while the dialog is visible.
* Set all the properties that are required before showing the dialog.
*
* Win32 details
* On windows the {@code IFileDialog} implementation (added in Windows Vista) is
* used. It supports many of the features that {@code GtkFileChooser} has, but
* there are some things it does not handle:
*
* - Any {@link FileFilter} added using a mimetype
*
*
* If any of these features are used the regular {@code GtkFileChooserDialog}
* will be used in place of the native one.
*
* Portal details
* When the {@code org.freedesktop.portal.FileChooser} portal is available on
* the session bus, it is used to bring up an out-of-process file chooser.
* Depending on the kind of session the application is running in, this may
* or may not be a GTK file chooser.
*
* macOS details
* On macOS the {@code NSSavePanel} and {@code NSOpenPanel} classes are used to provide
* native file chooser dialogs. Some features provided by {@code GtkFileChooser}
* are not supported:
*
* - Shortcut folders.
*
*/
@Generated("io.github.jwharm.JavaGI")
@Deprecated
public class FileChooserNative extends NativeDialog implements FileChooser {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a FileChooserNative proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FileChooserNative(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkFileChooserNative}.
*
* @param title Title of the native
* @param parent Transient parent of the native
* @param action Open or save mode for the dialog
* @param acceptLabel text to go in the accept button, or {@code null} for the default
* @param cancelLabel text to go in the cancel button, or {@code null} for the default
* @deprecated Use {@link FileDialog} instead
*/
@Deprecated
public FileChooserNative(@Nullable String title, @Nullable Window parent,
FileChooserAction action, @Nullable String acceptLabel, @Nullable String cancelLabel) {
this(constructNew(title, parent, action, acceptLabel, cancelLabel));
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the FileChooserNative class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_file_chooser_native_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected FileChooserNative asParent() {
FileChooserNative _parent = new FileChooserNative(handle());
_parent.callParent(true);
return _parent;
}
@Deprecated
private static MemorySegment constructNew(@Nullable String title, @Nullable Window parent,
FileChooserAction action, @Nullable String acceptLabel, @Nullable String cancelLabel) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_chooser_native_new.invokeExact(
(MemorySegment) (title == null ? MemorySegment.NULL : Interop.allocateNativeString(title, _arena)),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
action.getValue(),
(MemorySegment) (acceptLabel == null ? MemorySegment.NULL : Interop.allocateNativeString(acceptLabel, _arena)),
(MemorySegment) (cancelLabel == null ? MemorySegment.NULL : Interop.allocateNativeString(cancelLabel, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Retrieves the custom label text for the accept button.
*
* @return The custom label
* @deprecated Use {@link FileDialog} instead
*/
@Deprecated
public String getAcceptLabel() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_chooser_native_get_accept_label.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Retrieves the custom label text for the cancel button.
*
* @return The custom label
* @deprecated Use {@link FileDialog} instead
*/
@Deprecated
public String getCancelLabel() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_chooser_native_get_cancel_label.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Sets the custom label text for the accept button.
*
* If characters in {@code label} are preceded by an underscore, they are
* underlined. If you need a literal underscore character in a label,
* use “__” (two underscores). The first underlined character represents
* a keyboard accelerator called a mnemonic.
*
* Pressing Alt and that key should activate the button.
*
* @param acceptLabel custom label
* @deprecated Use {@link FileDialog} instead
*/
@Deprecated
public void setAcceptLabel(@Nullable String acceptLabel) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_file_chooser_native_set_accept_label.invokeExact(handle(),
(MemorySegment) (acceptLabel == null ? MemorySegment.NULL : Interop.allocateNativeString(acceptLabel, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the custom label text for the cancel button.
*
* If characters in {@code label} are preceded by an underscore, they are
* underlined. If you need a literal underscore character in a label,
* use “__” (two underscores). The first underlined character represents
* a keyboard accelerator called a mnemonic.
*
* Pressing Alt and that key should activate the button.
*
* @param cancelLabel custom label
* @deprecated Use {@link FileDialog} instead
*/
@Deprecated
public void setCancelLabel(@Nullable String cancelLabel) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_file_chooser_native_set_cancel_label.invokeExact(handle(),
(MemorySegment) (cancelLabel == null ? MemorySegment.NULL : Interop.allocateNativeString(cancelLabel, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* A {@link Builder} object constructs a {@code FileChooserNative}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class FileChooserNativeClass extends NativeDialog.NativeDialogClass {
/**
* Create a FileChooserNativeClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FileChooserNativeClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new FileChooserNativeClass.
*
* @param arena to control the memory allocation scope
*/
public FileChooserNativeClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new FileChooserNativeClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public FileChooserNativeClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
NativeDialog.NativeDialogClass.getMemoryLayout().withName("parent_class")
).withName("GtkFileChooserNativeClass");
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends NativeDialog.Builder implements FileChooser.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code FileChooserNative} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code FileChooserNative}.
*
* @return a new instance of {@code FileChooserNative} with the properties
* that were set in the Builder object.
*/
public FileChooserNative build() {
try {
var _instance = (FileChooserNative) GObject.withProperties(FileChooserNative.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The text used for the label on the accept button in the dialog, or
* {@code null} to use the default text.
*
* @param acceptLabel the value for the {@code accept-label} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAcceptLabel(String acceptLabel) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(acceptLabel);
addBuilderProperty("accept-label", _value);
return (B) this;
}
/**
* The text used for the label on the cancel button in the dialog, or
* {@code null} to use the default text.
*
* @param cancelLabel the value for the {@code cancel-label} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setCancelLabel(String cancelLabel) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(cancelLabel);
addBuilderProperty("cancel-label", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_file_chooser_native_new = Interop.downcallHandle(
"gtk_file_chooser_native_new", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_chooser_native_get_accept_label = Interop.downcallHandle(
"gtk_file_chooser_native_get_accept_label",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_chooser_native_get_cancel_label = Interop.downcallHandle(
"gtk_file_chooser_native_get_cancel_label",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_chooser_native_set_accept_label = Interop.downcallHandle(
"gtk_file_chooser_native_set_accept_label",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_chooser_native_set_cancel_label = Interop.downcallHandle(
"gtk_file_chooser_native_set_cancel_label",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
}
}