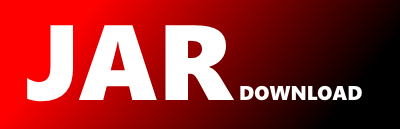
org.gnome.gtk.FileDialog Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.GErrorException;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.ArenaCloseAction;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.gio.AsyncReadyCallback;
import org.gnome.gio.AsyncResult;
import org.gnome.gio.Cancellable;
import org.gnome.gio.File;
import org.gnome.gio.ListModel;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* A {@code GtkFileDialog} object collects the arguments that
* are needed to present a file chooser dialog to the
* user, such as a title for the dialog and whether it
* should be modal.
*
* The dialog is shown with {@link FileDialog#open},
* {@link FileDialog#save}, etc.
*
* @version 4.10
*/
@Generated("io.github.jwharm.JavaGI")
public class FileDialog extends GObject {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a FileDialog proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FileDialog(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkFileDialog} object.
*/
public FileDialog() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the FileDialog class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_file_dialog_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected FileDialog asParent() {
FileDialog _parent = new FileDialog(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Retrieves the text used by the dialog on its accept button.
*
* @return the label shown on the file chooser's accept button.
*/
public String getAcceptLabel() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_accept_label.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the filter that will be selected by default
* in the file chooser dialog.
*
* @return the current filter
*/
public FileFilter getDefaultFilter() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_default_filter.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (FileFilter) InstanceCache.getForType(_result, FileFilter::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.FileFilter %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the filters that will be offered to the user
* in the file chooser dialog.
*
* @return the filters, as
* a {@code GListModel} of {@code GtkFileFilters}
*/
public ListModel getFilters() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_filters.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (ListModel) InstanceCache.getForType(_result, ListModel.ListModelImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.ListModel %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the file that will be initially selected in
* the file chooser dialog.
*
* @return the file
*/
public File getInitialFile() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_initial_file.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (File) InstanceCache.getForType(_result, File.FileImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.File %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the folder that will be set as the
* initial folder in the file chooser dialog.
*
* @return the folder
*/
public File getInitialFolder() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_initial_folder.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (File) InstanceCache.getForType(_result, File.FileImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.File %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the name for the file that should be initially set.
*
* @return the name
*/
public String getInitialName() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_initial_name.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Returns whether the file chooser dialog
* blocks interaction with the parent window
* while it is presented.
*
* @return {@code TRUE} if the file chooser dialog is modal
*/
public boolean getModal() {
int _result;
try {
_result = (int) MethodHandles.gtk_file_dialog_get_modal.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Returns the title that will be shown on the
* file chooser dialog.
*
* @return the title
*/
public String getTitle() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_get_title.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* This function initiates a file selection operation by
* presenting a file chooser dialog to the user.
*
* The {@code callback} will be called when the dialog is dismissed.
*
* @param parent the parent {@code GtkWindow}
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void open(@Nullable Window parent, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_file_dialog_open.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FileDialog#open} call and
* returns the resulting file.
*
* @param result a {@code GAsyncResult}
* @return the file that was selected.
* Otherwise, {@code NULL} is returned and {@code error} is set
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public File openFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_open_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (File) InstanceCache.getForType(_result, File.FileImpl::new, true);
}
}
/**
* This function initiates a multi-file selection operation by
* presenting a file chooser dialog to the user.
*
* The file chooser will initially be opened in the directory
* {@code Gtk.FileDialog:initial-folder}.
*
* The {@code callback} will be called when the dialog is dismissed.
*
* @param parent the parent {@code GtkWindow}
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void openMultiple(@Nullable Window parent, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_file_dialog_open_multiple.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FileDialog#open} call and
* returns the resulting files in a {@code GListModel}.
*
* @param result a {@code GAsyncResult}
* @return the file that was selected,
* as a {@code GListModel} of {@code GFiles}. Otherwise, {@code NULL} is returned
* and {@code error} is set
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public ListModel openMultipleFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_open_multiple_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (ListModel) InstanceCache.getForType(_result, ListModel.ListModelImpl::new, true);
}
}
/**
* This function initiates a file save operation by
* presenting a file chooser dialog to the user.
*
* The {@code callback} will be called when the dialog is dismissed.
*
* @param parent the parent {@code GtkWindow}
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void save(@Nullable Window parent, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_file_dialog_save.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FileDialog#save} call and
* returns the resulting file.
*
* @param result a {@code GAsyncResult}
* @return the file that was selected.
* Otherwise, {@code NULL} is returned and {@code error} is set
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public File saveFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_save_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (File) InstanceCache.getForType(_result, File.FileImpl::new, true);
}
}
/**
* This function initiates a directory selection operation by
* presenting a file chooser dialog to the user.
*
* If you pass {@code initialFolder}, the file chooser will initially be
* opened in the parent directory of that folder, otherwise, it
* will be in the directory {@code Gtk.FileDialog:initial-folder}.
*
* The {@code callback} will be called when the dialog is dismissed.
*
* @param parent the parent {@code GtkWindow}
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void selectFolder(@Nullable Window parent, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_file_dialog_select_folder.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FileDialog#selectFolder} call and
* returns the resulting file.
*
* @param result a {@code GAsyncResult}
* @return the file that was selected.
* Otherwise, {@code NULL} is returned and {@code error} is set
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public File selectFolderFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_select_folder_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (File) InstanceCache.getForType(_result, File.FileImpl::new, true);
}
}
/**
* This function initiates a multi-directory selection operation by
* presenting a file chooser dialog to the user.
*
* The file chooser will initially be opened in the directory
* {@code Gtk.FileDialog:initial-folder}.
*
* The {@code callback} will be called when the dialog is dismissed.
*
* @param parent the parent {@code GtkWindow}
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void selectMultipleFolders(@Nullable Window parent, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_file_dialog_select_multiple_folders.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FileDialog#selectMultipleFolders}
* call and returns the resulting files in a {@code GListModel}.
*
* @param result a {@code GAsyncResult}
* @return the file that was selected,
* as a {@code GListModel} of {@code GFiles}. Otherwise, {@code NULL} is returned
* and {@code error} is set
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public ListModel selectMultipleFoldersFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_file_dialog_select_multiple_folders_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (ListModel) InstanceCache.getForType(_result, ListModel.ListModelImpl::new, true);
}
}
/**
* Sets the label shown on the file chooser's accept button.
*
* Leaving the accept label unset or setting it as {@code NULL} will fall back to
* a default label, depending on what API is used to launch the file dialog.
*
* @param acceptLabel the new accept label
*/
public void setAcceptLabel(@Nullable String acceptLabel) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_file_dialog_set_accept_label.invokeExact(handle(),
(MemorySegment) (acceptLabel == null ? MemorySegment.NULL : Interop.allocateNativeString(acceptLabel, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the filter that will be selected by default
* in the file chooser dialog.
*
* If set to {@code null}, the first item in {@code Gtk.FileDialog:filters}
* will be used as the default filter. If that list is empty, the dialog
* will be unfiltered.
*
* @param filter a {@code GtkFileFilter}
*/
public void setDefaultFilter(@Nullable FileFilter filter) {
try {
MethodHandles.gtk_file_dialog_set_default_filter.invokeExact(handle(),
(MemorySegment) (filter == null ? MemorySegment.NULL : filter.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the filters that will be offered to the user
* in the file chooser dialog.
*
* @param filters a {@code GListModel} of {@code GtkFileFilters}
*/
public void setFilters(@Nullable ListModel filters) {
try {
MethodHandles.gtk_file_dialog_set_filters.invokeExact(handle(),
(MemorySegment) (filters == null ? MemorySegment.NULL : filters.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the file that will be initially selected in
* the file chooser dialog.
*
* This function is a shortcut for calling both
* gtk_file_dialog_set_initial_folder() and
* gtk_file_dialog_set_initial_name() with the directory and
* name of {@code file} respectively.
*
* @param file a {@code GFile}
*/
public void setInitialFile(@Nullable File file) {
try {
MethodHandles.gtk_file_dialog_set_initial_file.invokeExact(handle(),
(MemorySegment) (file == null ? MemorySegment.NULL : file.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the folder that will be set as the
* initial folder in the file chooser dialog.
*
* @param folder a {@code GFile}
*/
public void setInitialFolder(@Nullable File folder) {
try {
MethodHandles.gtk_file_dialog_set_initial_folder.invokeExact(handle(),
(MemorySegment) (folder == null ? MemorySegment.NULL : folder.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the name for the file that should be initially set.
* For saving dialogs, this will usually be pre-entered into the name field.
*
* If a file with this name already exists in the directory set via
* {@code Gtk.FileDialog:initial-folder}, the dialog should preselect it.
*
* @param name a UTF8 string
*/
public void setInitialName(@Nullable String name) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_file_dialog_set_initial_name.invokeExact(handle(),
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets whether the file chooser dialog
* blocks interaction with the parent window
* while it is presented.
*
* @param modal the new value
*/
public void setModal(boolean modal) {
try {
MethodHandles.gtk_file_dialog_set_modal.invokeExact(handle(), modal ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the title that will be shown on the
* file chooser dialog.
*
* @param title the new title
*/
public void setTitle(String title) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_file_dialog_set_title.invokeExact(handle(),
(MemorySegment) (title == null ? MemorySegment.NULL : Interop.allocateNativeString(title, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* A {@link Builder} object constructs a {@code FileDialog}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class FileDialogClass extends GObject.ObjectClass {
/**
* Create a FileDialogClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FileDialogClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new FileDialogClass.
*
* @param arena to control the memory allocation scope
*/
public FileDialogClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new FileDialogClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public FileDialogClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
GObject.ObjectClass.getMemoryLayout().withName("parent_class")
).withName("GtkFileDialogClass");
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code FileDialog} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code FileDialog}.
*
* @return a new instance of {@code FileDialog} with the properties
* that were set in the Builder object.
*/
public FileDialog build() {
try {
var _instance = (FileDialog) GObject.withProperties(FileDialog.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* Label for the file chooser's accept button.
*
* @param acceptLabel the value for the {@code accept-label} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAcceptLabel(String acceptLabel) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(acceptLabel);
addBuilderProperty("accept-label", _value);
return (B) this;
}
/**
* The default filter, that is, the filter that is initially
* active in the file chooser dialog.
*
* If the default filter is {@code null}, the first filter of {@code Gtk.FileDialog:filters}
* is used as the default filter. If that property contains no filter, the dialog will
* be unfiltered.
*
* If {@code Gtk.FileDialog:filters} is not {@code null}, the default filter should be part
* of the list. If it is not, the dialog may choose to not make it available.
*
* @param defaultFilter the value for the {@code default-filter} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setDefaultFilter(FileFilter defaultFilter) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(FileFilter.getType());
_value.setObject((GObject) defaultFilter);
addBuilderProperty("default-filter", _value);
return (B) this;
}
/**
* The list of filters.
*
* See {@code Gtk.FileDialog:default-filter} about how those two properties interact.
*
* @param filters the value for the {@code filters} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFilters(ListModel filters) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(ListModel.getType());
_value.setObject((GObject) filters);
addBuilderProperty("filters", _value);
return (B) this;
}
/**
* The initial file, that is, the file that is initially selected
* in the file chooser dialog
*
* This is a utility property that sets both {@code Gtk.FileDialog:initial-folder} and
* {@code Gtk.FileDialog:initial-name}.
*
* @param initialFile the value for the {@code initial-file} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setInitialFile(File initialFile) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(File.getType());
_value.setObject((GObject) initialFile);
addBuilderProperty("initial-file", _value);
return (B) this;
}
/**
* The initial folder, that is, the directory that is initially
* opened in the file chooser dialog
*
* @param initialFolder the value for the {@code initial-folder} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setInitialFolder(File initialFolder) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(File.getType());
_value.setObject((GObject) initialFolder);
addBuilderProperty("initial-folder", _value);
return (B) this;
}
/**
* The initial name, that is, the filename that is initially
* selected in the file chooser dialog.
*
* @param initialName the value for the {@code initial-name} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setInitialName(String initialName) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(initialName);
addBuilderProperty("initial-name", _value);
return (B) this;
}
/**
* Whether the file chooser dialog is modal.
*
* @param modal the value for the {@code modal} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setModal(boolean modal) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(modal);
addBuilderProperty("modal", _value);
return (B) this;
}
/**
* A title that may be shown on the file chooser dialog.
*
* @param title the value for the {@code title} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setTitle(String title) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(title);
addBuilderProperty("title", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_file_dialog_new = Interop.downcallHandle(
"gtk_file_dialog_new", FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_accept_label = Interop.downcallHandle(
"gtk_file_dialog_get_accept_label", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_default_filter = Interop.downcallHandle(
"gtk_file_dialog_get_default_filter", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_filters = Interop.downcallHandle(
"gtk_file_dialog_get_filters", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_initial_file = Interop.downcallHandle(
"gtk_file_dialog_get_initial_file", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_initial_folder = Interop.downcallHandle(
"gtk_file_dialog_get_initial_folder", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_initial_name = Interop.downcallHandle(
"gtk_file_dialog_get_initial_name", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_modal = Interop.downcallHandle(
"gtk_file_dialog_get_modal", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_get_title = Interop.downcallHandle(
"gtk_file_dialog_get_title", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_open = Interop.downcallHandle(
"gtk_file_dialog_open", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_file_dialog_open_finish = Interop.downcallHandle(
"gtk_file_dialog_open_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_open_multiple = Interop.downcallHandle(
"gtk_file_dialog_open_multiple", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_file_dialog_open_multiple_finish = Interop.downcallHandle(
"gtk_file_dialog_open_multiple_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_save = Interop.downcallHandle(
"gtk_file_dialog_save", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_file_dialog_save_finish = Interop.downcallHandle(
"gtk_file_dialog_save_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_select_folder = Interop.downcallHandle(
"gtk_file_dialog_select_folder", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_file_dialog_select_folder_finish = Interop.downcallHandle(
"gtk_file_dialog_select_folder_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_select_multiple_folders = Interop.downcallHandle(
"gtk_file_dialog_select_multiple_folders",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_select_multiple_folders_finish = Interop.downcallHandle(
"gtk_file_dialog_select_multiple_folders_finish",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_accept_label = Interop.downcallHandle(
"gtk_file_dialog_set_accept_label", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_default_filter = Interop.downcallHandle(
"gtk_file_dialog_set_default_filter", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_filters = Interop.downcallHandle(
"gtk_file_dialog_set_filters", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_initial_file = Interop.downcallHandle(
"gtk_file_dialog_set_initial_file", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_initial_folder = Interop.downcallHandle(
"gtk_file_dialog_set_initial_folder", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_initial_name = Interop.downcallHandle(
"gtk_file_dialog_set_initial_name", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_file_dialog_set_modal = Interop.downcallHandle(
"gtk_file_dialog_set_modal", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_file_dialog_set_title = Interop.downcallHandle(
"gtk_file_dialog_set_title", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}