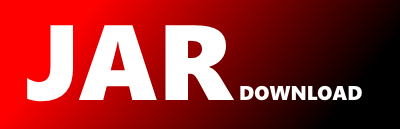
org.gnome.gtk.FlowBoxChild Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.Constants;
import io.github.jwharm.javagi.base.FunctionPointer;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.SignalConnection;
import io.github.jwharm.javagi.gobject.types.Overrides;
import io.github.jwharm.javagi.gobject.types.Signals;
import io.github.jwharm.javagi.interop.Arenas;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.FunctionalInterface;
import java.lang.NullPointerException;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import javax.annotation.processing.Generated;
import org.gnome.glib.GLib;
import org.gnome.glib.LogLevelFlags;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkFlowBoxChild} is the kind of widget that can be added to a {@code GtkFlowBox}.
*/
@Generated("io.github.jwharm.JavaGI")
public class FlowBoxChild extends Widget implements Accessible, Buildable, ConstraintTarget {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a FlowBoxChild proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FlowBoxChild(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkFlowBoxChild}.
*
* This should only be used as a child of a {@code GtkFlowBox}.
*/
public FlowBoxChild() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the FlowBoxChild class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_flow_box_child_get_type");
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
Widget.getMemoryLayout().withName("parent_instance")
).withName("GtkFlowBoxChild");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected FlowBoxChild asParent() {
FlowBoxChild _parent = new FlowBoxChild(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_flow_box_child_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Marks this FlowBoxChild as changed, causing any state that depends on this
* to be updated.
*
* This affects sorting and filtering.
*
* Note that calls to this method must be in sync with the data
* used for the sorting and filtering functions. For instance, if
* the list is mirroring some external data set, and two children
* changed in the external data set when you call
* gtk_flow_box_child_changed() on the first child, the sort function
* must only read the new data for the first of the two changed
* children, otherwise the resorting of the children will be wrong.
*
* This generally means that if you don’t fully control the data
* model, you have to duplicate the data that affects the sorting
* and filtering functions into the widgets themselves.
*
* Another alternative is to call {@link FlowBox#invalidateSort}
* on any model change, but that is more expensive.
*/
public void changed() {
try {
MethodHandles.gtk_flow_box_child_changed.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Gets the child widget of this FlowBoxChild.
*
* @return the child widget of this FlowBoxChild
*/
public Widget getChild() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_flow_box_child_get_child.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Widget %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the current index of the this FlowBoxChild in its {@code GtkFlowBox} container.
*
* @return the index of the this FlowBoxChild, or -1 if the this FlowBoxChild is not
* in a flow box
*/
public int getIndex() {
int _result;
try {
_result = (int) MethodHandles.gtk_flow_box_child_get_index.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Returns whether the this FlowBoxChild is currently selected in its
* {@code GtkFlowBox} container.
*
* @return {@code true} if this FlowBoxChild is selected
*/
public boolean isSelected() {
int _result;
try {
_result = (int) MethodHandles.gtk_flow_box_child_is_selected.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Sets the child widget of this FlowBoxChild.
*
* @param child the child widget
*/
public void setChild(@Nullable Widget child) {
try {
MethodHandles.gtk_flow_box_child_set_child.invokeExact(handle(),
(MemorySegment) (child == null ? MemorySegment.NULL : child.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
protected void activate() {
try {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FlowBoxChildClass.getMemoryLayout(), "activate");
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
Interop.downcallHandle(_func, _fdesc).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted when the user activates a child widget in a {@code GtkFlowBox}.
*
* This can happen either by clicking or double-clicking,
* or via a keybinding.
*
* This is a keybinding signal,
* but it can be used by applications for their own purposes.
*
* The default bindings are {@code Space} and {@code Enter}.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see ActivateCallback#run
*/
public SignalConnection onActivate(ActivateCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("activate", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "activate" signal. See {@link #onActivate}.
*/
public void emitActivate() {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("activate", _arena);
Object[] _args = new Object[0];
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code FlowBoxChild}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Functional interface declaration of the {@code ActivateCallback} callback.
*
* @see ActivateCallback#run
*/
@FunctionalInterface
public interface ActivateCallback extends FunctionPointer {
/**
* Emitted when the user activates a child widget in a {@code GtkFlowBox}.
*
* This can happen either by clicking or double-clicking,
* or via a keybinding.
*
* This is a keybinding signal,
* but it can be used by applications for their own purposes.
*
* The default bindings are {@code Space} and {@code Enter}.
*/
void run();
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceFlowBoxChild) {
run();
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), ActivateCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
public static class FlowBoxChildClass extends Widget.WidgetClass {
private Method _activateMethod;
/**
* Create a FlowBoxChildClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FlowBoxChildClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new FlowBoxChildClass.
*
* @param arena to control the memory allocation scope
*/
public FlowBoxChildClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new FlowBoxChildClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public FlowBoxChildClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
Widget.WidgetClass.getMemoryLayout().withName("parent_class"),
ValueLayout.ADDRESS.withName("activate"),
MemoryLayout.sequenceLayout(8, ValueLayout.ADDRESS).withName("padding")
).withName("GtkFlowBoxChildClass");
}
/**
* Override virtual method {@code activate}.
*
* @param method the method to invoke
*/
public void overrideActivate(Arena arena, Method method) {
this._activateMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), FlowBoxChildClass.class, "activateUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("activate"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private void activateUpcall(MemorySegment child) {
try {
Arena _arena = Arena.ofAuto();
this._activateMethod.invoke((FlowBoxChild) InstanceCache.getForType(child, FlowBoxChild::new, false));
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _activateMethod);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends Widget.Builder implements Accessible.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code FlowBoxChild} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code FlowBoxChild}.
*
* @return a new instance of {@code FlowBoxChild} with the properties
* that were set in the Builder object.
*/
public FlowBoxChild build() {
try {
var _instance = (FlowBoxChild) GObject.withProperties(FlowBoxChild.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The child widget.
*
* @param child the value for the {@code child} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setChild(Widget child) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Widget.getType());
_value.setObject((GObject) child);
addBuilderProperty("child", _value);
return (B) this;
}
/**
* Emitted when the user activates a child widget in a {@code GtkFlowBox}.
*
* This can happen either by clicking or double-clicking,
* or via a keybinding.
*
* This is a keybinding signal,
* but it can be used by applications for their own purposes.
*
* The default bindings are {@code Space} and {@code Enter}.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see ActivateCallback#run
*/
public B onActivate(ActivateCallback handler) {
connect("activate", handler);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_flow_box_child_new = Interop.downcallHandle(
"gtk_flow_box_child_new", FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_flow_box_child_changed = Interop.downcallHandle(
"gtk_flow_box_child_changed", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_flow_box_child_get_child = Interop.downcallHandle(
"gtk_flow_box_child_get_child", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_flow_box_child_get_index = Interop.downcallHandle(
"gtk_flow_box_child_get_index", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_flow_box_child_is_selected = Interop.downcallHandle(
"gtk_flow_box_child_is_selected", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_flow_box_child_set_child = Interop.downcallHandle(
"gtk_flow_box_child_set_child", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}