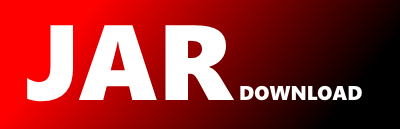
org.gnome.gtk.FontChooser Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.Constants;
import io.github.jwharm.javagi.base.FunctionPointer;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.base.Proxy;
import io.github.jwharm.javagi.gobject.BuilderInterface;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.SignalConnection;
import io.github.jwharm.javagi.gobject.types.Overrides;
import io.github.jwharm.javagi.gobject.types.Signals;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Arenas;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.Deprecated;
import java.lang.FunctionalInterface;
import java.lang.NullPointerException;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.EnumSet;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.gnome.glib.GLib;
import org.gnome.glib.LogLevelFlags;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.TypeInstance;
import org.gnome.gobject.TypeInterface;
import org.gnome.gobject.Value;
import org.gnome.pango.FontDescription;
import org.gnome.pango.FontFace;
import org.gnome.pango.FontFamily;
import org.gnome.pango.FontMap;
import org.jetbrains.annotations.Nullable;
/**
* {@code GtkFontChooser} is an interface that can be implemented by widgets
* for choosing fonts.
*
* In GTK, the main objects that implement this interface are
* {@link FontChooserWidget}, {@link FontChooserDialog} and
* {@link FontButton}.
*/
@Generated("io.github.jwharm.JavaGI")
@Deprecated
public interface FontChooser extends Proxy {
/**
* Get the GType of the FontChooser class
*
* @return the GType
*/
static Type getType() {
return Interop.getType("gtk_font_chooser_get_type");
}
/**
* Gets the currently-selected font name.
*
* Note that this can be a different string than what you set with
* {@link FontChooser#setFont}, as the font chooser widget may
* normalize font names and thus return a string with a different
* structure. For example, “Helvetica Italic Bold 12” could be
* normalized to “Helvetica Bold Italic 12”.
*
* Use {@link org.gnome.pango.FontDescription#equal} if you want to compare two
* font descriptions.
*
* @return A string with the name
* of the current font
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default String getFont() {
MemorySegment _result;
try {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, true);
}
/**
* Gets the currently-selected font.
*
* Note that this can be a different string than what you set with
* {@link FontChooser#setFont}, as the font chooser widget may
* normalize font names and thus return a string with a different
* structure. For example, “Helvetica Italic Bold 12” could be
* normalized to “Helvetica Bold Italic 12”.
*
* Use {@link org.gnome.pango.FontDescription#equal} if you want to compare two
* font descriptions.
*
* @return A {@code PangoFontDescription} for the
* current font
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default FontDescription getFontDesc() {
MemorySegment _result;
try {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font_desc.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new FontDescription(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, FontDescription.getType());
}
return _instance;
}
/**
* Gets the {@code PangoFontFace} representing the selected font group
* details (i.e. family, slant, weight, width, etc).
*
* If the selected font is not installed, returns {@code null}.
*
* @return A {@code PangoFontFace} representing the
* selected font group details
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default FontFace getFontFace() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "get_font_face", FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font_face.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (FontFace) InstanceCache.getForType(_result, FontFace.FontFaceImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.pango.FontFace %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the {@code PangoFontFamily} representing the selected font family.
*
* Font families are a collection of font faces.
*
* If the selected font is not installed, returns {@code null}.
*
* @return A {@code PangoFontFamily} representing the
* selected font family
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default FontFamily getFontFamily() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "get_font_family",
FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font_family.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (FontFamily) InstanceCache.getForType(_result, FontFamily.FontFamilyImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.pango.FontFamily %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the currently-selected font features.
*
* The format of the returned string is compatible with the
* CSS font-feature-settings property.
* It can be passed to {@link org.gnome.pango.AttrFontFeatures#new_}.
*
* @return the currently selected font features
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default String getFontFeatures() {
MemorySegment _result;
try {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font_features.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, true);
}
/**
* Gets the custom font map of this font chooser widget,
* or {@code null} if it does not have one.
*
* @return a {@code PangoFontMap}
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default FontMap getFontMap() {
MemorySegment _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "get_font_map", FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (MemorySegment) Interop.downcallHandle(_func, _fdesc).invokeExact(
handle());
} else {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_font_map.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (FontMap) InstanceCache.getForType(_result, FontMap.FontMapImpl::new, true);
}
/**
* The selected font size.
*
* @return A n integer representing the selected font size,
* or -1 if no font size is selected.
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default int getFontSize() {
int _result;
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "get_font_size", FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
_result = (int) Interop.downcallHandle(_func, _fdesc).invokeExact(handle());
} else {
_result = (int) FontChooserMethodHandles.gtk_font_chooser_get_font_size.invokeExact(
handle());
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the language that is used for font features.
*
* @return the currently selected language
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default String getLanguage() {
MemorySegment _result;
try {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_language.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, true);
}
/**
* Returns the current level of granularity for selecting fonts.
*
* @return the current granularity level
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default Set getLevel() {
int _result;
try {
_result = (int) FontChooserMethodHandles.gtk_font_chooser_get_level.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.intToEnumSet(FontChooserLevel.class, FontChooserLevel::of, _result);
}
/**
* Gets the text displayed in the preview area.
*
* @return the text displayed in the preview area
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default String getPreviewText() {
MemorySegment _result;
try {
_result = (MemorySegment) FontChooserMethodHandles.gtk_font_chooser_get_preview_text.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, true);
}
/**
* Returns whether the preview entry is shown or not.
*
* @return {@code true} if the preview entry is shown or {@code false} if it is hidden.
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default boolean getShowPreviewEntry() {
int _result;
try {
_result = (int) FontChooserMethodHandles.gtk_font_chooser_get_show_preview_entry.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Adds a filter function that decides which fonts to display
* in the font chooser.
*
* @param filter a {@code GtkFontFilterFunc}
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setFilterFunc(@Nullable FontFilterFunc filter) {
try (var _arena = Arena.ofConfined()) {
final Arena _filterScope = Arena.ofConfined();
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "set_filter_func",
FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
Interop.downcallHandle(_func, _fdesc).invokeExact(handle(),
(MemorySegment) (filter == null ? MemorySegment.NULL : filter.toCallback(_filterScope)),
Arenas.cacheArena(_filterScope), Arenas.CLOSE_CB_SYM);
} else {
FontChooserMethodHandles.gtk_font_chooser_set_filter_func.invokeExact(handle(),
(MemorySegment) (filter == null ? MemorySegment.NULL : filter.toCallback(_filterScope)),
Arenas.cacheArena(_filterScope), Arenas.CLOSE_CB_SYM);
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the currently-selected font.
*
* @param fontname a font name like “Helvetica 12” or “Times Bold 18”
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setFont(String fontname) {
try (var _arena = Arena.ofConfined()) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_font.invokeExact(handle(),
(MemorySegment) (fontname == null ? MemorySegment.NULL : Interop.allocateNativeString(fontname, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the currently-selected font from {@code fontDesc}.
*
* @param fontDesc a {@code PangoFontDescription}
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setFontDesc(FontDescription fontDesc) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_font_desc.invokeExact(handle(),
(MemorySegment) (fontDesc == null ? MemorySegment.NULL : fontDesc.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets a custom font map to use for this font chooser widget.
*
* A custom font map can be used to present application-specific
* fonts instead of or in addition to the normal system fonts.
*
{@code FcConfig *config;
* PangoFontMap *fontmap;
*
* config = FcInitLoadConfigAndFonts ();
* FcConfigAppFontAddFile (config, my_app_font_file);
*
* fontmap = pango_cairo_font_map_new_for_font_type (CAIRO_FONT_TYPE_FT);
* pango_fc_font_map_set_config (PANGO_FC_FONT_MAP (fontmap), config);
*
* gtk_font_chooser_set_font_map (font_chooser, fontmap);
* }
*
* Note that other GTK widgets will only be able to use the
* application-specific font if it is present in the font map they use:
*
{@code context = gtk_widget_get_pango_context (label);
* pango_context_set_font_map (context, fontmap);
* }
*
* @param fontmap a {@code PangoFontMap}
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setFontMap(@Nullable FontMap fontmap) {
try {
if (((TypeInstance) this).callParent()) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MemorySegment _func = Overrides.lookupVirtualMethodParent(handle(),
FontChooserIface.getMemoryLayout(), "set_font_map", FontChooser.getType());
if (_func.equals(MemorySegment.NULL)) throw new NullPointerException();
Interop.downcallHandle(_func, _fdesc).invokeExact(handle(),
(MemorySegment) (fontmap == null ? MemorySegment.NULL : fontmap.handle()));
} else {
FontChooserMethodHandles.gtk_font_chooser_set_font_map.invokeExact(handle(),
(MemorySegment) (fontmap == null ? MemorySegment.NULL : fontmap.handle()));
}
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the language to use for font features.
*
* @param language a language
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setLanguage(String language) {
try (var _arena = Arena.ofConfined()) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_language.invokeExact(handle(),
(MemorySegment) (language == null ? MemorySegment.NULL : Interop.allocateNativeString(language, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the desired level of granularity for selecting fonts.
*
* @param level the desired level of granularity
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setLevel(Set level) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_level.invokeExact(handle(),
Interop.enumSetToInt(level));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the desired level of granularity for selecting fonts.
*
* @param level the desired level of granularity
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setLevel(FontChooserLevel... level) {
setLevel((level == null ? null : (level.length == 0) ? EnumSet.noneOf(FontChooserLevel.class) : EnumSet.of(level[0], level)));
}
/**
* Sets the text displayed in the preview area.
*
* The {@code text} is used to show how the selected font looks.
*
* @param text the text to display in the preview area
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setPreviewText(String text) {
try (var _arena = Arena.ofConfined()) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_preview_text.invokeExact(handle(),
(MemorySegment) (text == null ? MemorySegment.NULL : Interop.allocateNativeString(text, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Shows or hides the editable preview entry.
*
* @param showPreviewEntry whether to show the editable preview entry or not
* @deprecated Use {@link FontDialog} and {@link FontDialogButton}
* instead
*/
@Deprecated
default void setShowPreviewEntry(boolean showPreviewEntry) {
try {
FontChooserMethodHandles.gtk_font_chooser_set_show_preview_entry.invokeExact(handle(),
showPreviewEntry ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted when a font is activated.
*
* This usually happens when the user double clicks an item,
* or an item is selected and the user presses one of the keys
* Space, Shift+Space, Return or Enter.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @deprecated Use {@link FontDialog} and {@link FontDialogButton} instead
* @see FontActivatedCallback#run
*/
@Deprecated
default SignalConnection onFontActivated(FontActivatedCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("font-activated", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "font-activated" signal. See {@link #onFontActivated}.
*/
@Deprecated
default void emitFontActivated(String fontname) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("font-activated", _arena);
Object[] _args = new Object[] {
(MemorySegment) (fontname == null ? MemorySegment.NULL : Interop.allocateNativeString(fontname, _arena))};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* The FontChooserImpl type represents a native instance of the FontChooser interface.
*/
class FontChooserImpl extends GObject implements FontChooser {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Creates a new instance of FontChooser for the provided memory address.
*
* @param address the memory address of the instance
*/
public FontChooserImpl(MemorySegment address) {
super(address);
}
}
/**
* Functional interface declaration of the {@code FontActivatedCallback} callback.
*
* @see FontActivatedCallback#run
*/
@FunctionalInterface
@Deprecated
interface FontActivatedCallback extends FunctionPointer {
/**
* Emitted when a font is activated.
*
* This usually happens when the user double clicks an item,
* or an item is selected and the user presses one of the keys
* Space, Shift+Space, Return or Enter.
*
* @deprecated Use {@link FontDialog} and {@link FontDialogButton} instead
*/
@Deprecated
void run(String fontname);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceFontChooser, MemorySegment fontname) {
Arena _arena = Arena.ofAuto();
run(Interop.getStringFrom(fontname, false));
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontActivatedCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
class FontChooserIface extends TypeInterface {
private Method _getFontFamilyMethod;
private Method _getFontFaceMethod;
private Method _getFontSizeMethod;
private Method _setFilterFuncMethod;
private Method _fontActivatedMethod;
private Method _setFontMapMethod;
private Method _getFontMapMethod;
/**
* Create a FontChooserIface proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FontChooserIface(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new FontChooserIface.
*
* @param arena to control the memory allocation scope
*/
public FontChooserIface(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new FontChooserIface.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public FontChooserIface() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
TypeInterface.getMemoryLayout().withName("base_iface"),
ValueLayout.ADDRESS.withName("get_font_family"),
ValueLayout.ADDRESS.withName("get_font_face"),
ValueLayout.ADDRESS.withName("get_font_size"),
ValueLayout.ADDRESS.withName("set_filter_func"),
ValueLayout.ADDRESS.withName("font_activated"),
ValueLayout.ADDRESS.withName("set_font_map"),
ValueLayout.ADDRESS.withName("get_font_map"),
MemoryLayout.sequenceLayout(10, ValueLayout.ADDRESS).withName("padding")
).withName("GtkFontChooserIface");
}
/**
* Override virtual method {@code get_font_family}.
*
* @param method the method to invoke
*/
public void overrideGetFontFamily(Arena arena, Method method) {
this._getFontFamilyMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "getFontFamilyUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_font_family"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getFontFamilyUpcall(MemorySegment fontchooser) {
try {
Arena _arena = Arena.ofAuto();
var _result = (FontFamily) this._getFontFamilyMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false));
if (_result == null) return MemorySegment.NULL;
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getFontFamilyMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_font_face}.
*
* @param method the method to invoke
*/
public void overrideGetFontFace(Arena arena, Method method) {
this._getFontFaceMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "getFontFaceUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_font_face"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getFontFaceUpcall(MemorySegment fontchooser) {
try {
Arena _arena = Arena.ofAuto();
var _result = (FontFace) this._getFontFaceMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false));
if (_result == null) return MemorySegment.NULL;
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getFontFaceMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_font_size}.
*
* @param method the method to invoke
*/
public void overrideGetFontSize(Arena arena, Method method) {
this._getFontSizeMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "getFontSizeUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_font_size"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private int getFontSizeUpcall(MemorySegment fontchooser) {
try {
Arena _arena = Arena.ofAuto();
var _result = (int) this._getFontSizeMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false));
return _result;
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getFontSizeMethod);
return 0;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code set_filter_func}.
*
* @param method the method to invoke
*/
public void overrideSetFilterFunc(Arena arena, Method method) {
this._setFilterFuncMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "setFilterFuncUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("set_filter_func"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private void setFilterFuncUpcall(MemorySegment fontchooser, MemorySegment filter,
MemorySegment userData, MemorySegment destroy) {
try {
Arena _arena = Arena.ofAuto();
this._setFilterFuncMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false));
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _setFilterFuncMethod);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code font_activated}.
*
* @param method the method to invoke
*/
public void overrideFontActivated(Arena arena, Method method) {
this._fontActivatedMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "fontActivatedUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("font_activated"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private void fontActivatedUpcall(MemorySegment chooser, MemorySegment fontname) {
try {
Arena _arena = Arena.ofAuto();
this._fontActivatedMethod.invoke((FontChooser) InstanceCache.getForType(chooser, FontChooserImpl::new, false), Interop.getStringFrom(fontname, false));
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _fontActivatedMethod);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code set_font_map}.
*
* @param method the method to invoke
*/
public void overrideSetFontMap(Arena arena, Method method) {
this._setFontMapMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "setFontMapUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("set_font_map"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private void setFontMapUpcall(MemorySegment fontchooser, MemorySegment fontmap) {
try {
Arena _arena = Arena.ofAuto();
this._setFontMapMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false), (FontMap) InstanceCache.getForType(fontmap, FontMap.FontMapImpl::new, false));
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _setFontMapMethod);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Override virtual method {@code get_font_map}.
*
* @param method the method to invoke
*/
public void overrideGetFontMap(Arena arena, Method method) {
this._getFontMapMethod = method;
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), FontChooserIface.class, "getFontMapUpcall", _fdesc);
MemorySegment _address = Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("get_font_map"))
.set(handle(), 0, (method == null ? MemorySegment.NULL : _address));
}
private MemorySegment getFontMapUpcall(MemorySegment fontchooser) {
try {
Arena _arena = Arena.ofAuto();
var _result = (FontMap) this._getFontMapMethod.invoke((FontChooser) InstanceCache.getForType(fontchooser, FontChooserImpl::new, false));
if (_result == null) return MemorySegment.NULL;
if (_result instanceof GObject _gobject) _gobject.ref();
return _result.handle();
} catch (InvocationTargetException ite) {
GLib.log(Constants.LOG_DOMAIN, LogLevelFlags.LEVEL_WARNING, ite.getCause().toString() + " in " + _getFontMapMethod);
return MemorySegment.NULL;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
interface Builder> extends BuilderInterface {
/**
* The font description as a string, e.g. "Sans Italic 12".
*
* @param font the value for the {@code font} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setFont(String font) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(font);
addBuilderProperty("font", _value);
return (B) this;
}
/**
* The font description as a {@code PangoFontDescription}.
*
* @param fontDesc the value for the {@code font-desc} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setFontDesc(FontDescription fontDesc) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(FontDescription.getType());
_value.setBoxed(fontDesc.handle());
addBuilderProperty("font-desc", _value);
return (B) this;
}
/**
* The language for which the font features were selected.
*
* @param language the value for the {@code language} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setLanguage(String language) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(language);
addBuilderProperty("language", _value);
return (B) this;
}
/**
* The level of granularity to offer for selecting fonts.
*
* @param level the value for the {@code level} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setLevel(Set level) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(FontChooserLevel.getType());
_value.setFlags(Interop.enumSetToInt(level));
addBuilderProperty("level", _value);
return (B) this;
}
/**
* The string with which to preview the font.
*
* @param previewText the value for the {@code preview-text} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setPreviewText(String previewText) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(previewText);
addBuilderProperty("preview-text", _value);
return (B) this;
}
/**
* Whether to show an entry to change the preview text.
*
* @param showPreviewEntry the value for the {@code show-preview-entry} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setShowPreviewEntry(boolean showPreviewEntry) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(showPreviewEntry);
addBuilderProperty("show-preview-entry", _value);
return (B) this;
}
/**
* The level of granularity to offer for selecting fonts.
*
* @param level the value for the {@code level} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
@Deprecated
default B setLevel(FontChooserLevel... level) {
return setLevel((level == null ? null : (level.length == 0) ? EnumSet.noneOf(FontChooserLevel.class) : EnumSet.of(level[0], level)));
}
}
}