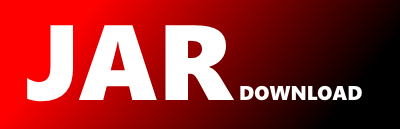
org.gnome.gtk.FontDialog Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.GErrorException;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.ArenaCloseAction;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.gio.AsyncReadyCallback;
import org.gnome.gio.AsyncResult;
import org.gnome.gio.Cancellable;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.gnome.pango.FontDescription;
import org.gnome.pango.FontFace;
import org.gnome.pango.FontFamily;
import org.gnome.pango.FontMap;
import org.gnome.pango.Language;
import org.jetbrains.annotations.Nullable;
/**
* A {@code GtkFontDialog} object collects the arguments that
* are needed to present a font chooser dialog to the
* user, such as a title for the dialog and whether it
* should be modal.
*
* The dialog is shown with the {@link FontDialog#chooseFont}
* function or its variants.
*
* See {@link FontDialogButton} for a convenient control
* that uses {@code GtkFontDialog} and presents the results.
*
* @version 4.10
*/
@Generated("io.github.jwharm.JavaGI")
public class FontDialog extends GObject {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a FontDialog proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FontDialog(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkFontDialog} object.
*/
public FontDialog() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the FontDialog class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_font_dialog_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected FontDialog asParent() {
FontDialog _parent = new FontDialog(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* This function initiates a font selection operation by
* presenting a dialog to the user for selecting a font face
* (i.e. a font family and style, but not a specific font size).
*
* @param parent the parent {@code GtkWindow}
* @param initialValue the initial value
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void chooseFace(@Nullable Window parent, @Nullable FontFace initialValue,
@Nullable Cancellable cancellable, @Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_font_dialog_choose_face.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (initialValue == null ? MemorySegment.NULL : initialValue.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FontDialog#chooseFace} call
* and returns the resulting font face.
*
* @param result a {@code GAsyncResult}
* @return the selected font face
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public FontFace chooseFaceFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_choose_face_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (FontFace) InstanceCache.getForType(_result, FontFace.FontFaceImpl::new, true);
}
}
/**
* This function initiates a font selection operation by
* presenting a dialog to the user for selecting a font family.
*
* @param parent the parent {@code GtkWindow}
* @param initialValue the initial value
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void chooseFamily(@Nullable Window parent, @Nullable FontFamily initialValue,
@Nullable Cancellable cancellable, @Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_font_dialog_choose_family.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (initialValue == null ? MemorySegment.NULL : initialValue.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FontDialog#chooseFamily} call
* and returns the resulting family.
*
* This function never returns an error. If the operation is
* not finished successfully, the value passed as {@code initialValue}
* to {@link FontDialog#chooseFamily} is returned.
*
* @param result a {@code GAsyncResult}
* @return the selected family
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public FontFamily chooseFamilyFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_choose_family_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (FontFamily) InstanceCache.getForType(_result, FontFamily.FontFamilyImpl::new, true);
}
}
/**
* This function initiates a font selection operation by
* presenting a dialog to the user for selecting a font.
*
* If you want to let the user select font features as well,
* use {@link FontDialog#chooseFontAndFeatures} instead.
*
* @param parent the parent {@code GtkWindow}
* @param initialValue the font to select initially
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void chooseFont(@Nullable Window parent, @Nullable FontDescription initialValue,
@Nullable Cancellable cancellable, @Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_font_dialog_choose_font.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (initialValue == null ? MemorySegment.NULL : initialValue.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* This function initiates a font selection operation by
* presenting a dialog to the user for selecting a font and
* font features.
*
* Font features affect how the font is rendered, for example
* enabling glyph variants or ligatures.
*
* @param parent the parent {@code GtkWindow}
* @param initialValue the font to select initially
* @param cancellable a {@code GCancellable} to cancel the operation
* @param callback a callback to call when the
* operation is complete
*/
public void chooseFontAndFeatures(@Nullable Window parent,
@Nullable FontDescription initialValue, @Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.gtk_font_dialog_choose_font_and_features.invokeExact(handle(),
(MemorySegment) (parent == null ? MemorySegment.NULL : parent.handle()),
(MemorySegment) (initialValue == null ? MemorySegment.NULL : initialValue.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes the {@link FontDialog#chooseFont} call
* and returns the resulting font description.
*
* @param result a {@code GAsyncResult}
* @return the selected font
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public FontDescription chooseFontFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_choose_font_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new FontDescription(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, FontDescription.getType());
}
return _instance;
}
}
/**
* Returns the filter that decides which fonts to display
* in the font chooser dialog.
*
* @return the filter
*/
public Filter getFilter() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_get_filter.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (Filter) InstanceCache.getForType(_result, Filter::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Filter %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Returns the fontmap from which fonts are selected,
* or {@code NULL} for the default fontmap.
*
* @return the fontmap
*/
public FontMap getFontMap() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_get_font_map.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (FontMap) InstanceCache.getForType(_result, FontMap.FontMapImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.pango.FontMap %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Returns the language for which font features are applied.
*
* @return the language for font features
*/
public Language getLanguage() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_get_language.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new Language(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, Language.getType());
}
return _instance;
}
/**
* Returns whether the font chooser dialog
* blocks interaction with the parent window
* while it is presented.
*
* @return {@code TRUE} if the font chooser dialog is modal
*/
public boolean getModal() {
int _result;
try {
_result = (int) MethodHandles.gtk_font_dialog_get_modal.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Returns the title that will be shown on the
* font chooser dialog.
*
* @return the title
*/
public String getTitle() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_font_dialog_get_title.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Adds a filter that decides which fonts to display
* in the font chooser dialog.
*
* The {@code GtkFilter} must be able to handle both {@code PangoFontFamily}
* and {@code PangoFontFace} objects.
*
* @param filter a {@code GtkFilter}
*/
public void setFilter(@Nullable Filter filter) {
try {
MethodHandles.gtk_font_dialog_set_filter.invokeExact(handle(),
(MemorySegment) (filter == null ? MemorySegment.NULL : filter.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the fontmap from which fonts are selected.
*
* If {@code fontmap} is {@code NULL}, the default fontmap is used.
*
* @param fontmap the fontmap
*/
public void setFontMap(@Nullable FontMap fontmap) {
try {
MethodHandles.gtk_font_dialog_set_font_map.invokeExact(handle(),
(MemorySegment) (fontmap == null ? MemorySegment.NULL : fontmap.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the language for which font features are applied.
*
* @param language the language for font features
*/
public void setLanguage(Language language) {
try {
MethodHandles.gtk_font_dialog_set_language.invokeExact(handle(),
(MemorySegment) (language == null ? MemorySegment.NULL : language.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets whether the font chooser dialog
* blocks interaction with the parent window
* while it is presented.
*
* @param modal the new value
*/
public void setModal(boolean modal) {
try {
MethodHandles.gtk_font_dialog_set_modal.invokeExact(handle(), modal ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the title that will be shown on the
* font chooser dialog.
*
* @param title the new title
*/
public void setTitle(String title) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_font_dialog_set_title.invokeExact(handle(),
(MemorySegment) (title == null ? MemorySegment.NULL : Interop.allocateNativeString(title, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* A {@link Builder} object constructs a {@code FontDialog}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class FontDialogClass extends GObject.ObjectClass {
/**
* Create a FontDialogClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public FontDialogClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new FontDialogClass.
*
* @param arena to control the memory allocation scope
*/
public FontDialogClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new FontDialogClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public FontDialogClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
GObject.ObjectClass.getMemoryLayout().withName("parent_class")
).withName("GtkFontDialogClass");
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code FontDialog} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code FontDialog}.
*
* @return a new instance of {@code FontDialog} with the properties
* that were set in the Builder object.
*/
public FontDialog build() {
try {
var _instance = (FontDialog) GObject.withProperties(FontDialog.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* Sets a filter to restrict what fonts are shown
* in the font chooser dialog.
*
* @param filter the value for the {@code filter} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFilter(Filter filter) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Filter.getType());
_value.setObject((GObject) filter);
addBuilderProperty("filter", _value);
return (B) this;
}
/**
* Sets a custom font map to select fonts from.
*
* A custom font map can be used to present application-specific
* fonts instead of or in addition to the normal system fonts.
*
* @param fontMap the value for the {@code font-map} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFontMap(FontMap fontMap) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(FontMap.getType());
_value.setObject((GObject) fontMap);
addBuilderProperty("font-map", _value);
return (B) this;
}
/**
* The language for which the font features are selected.
*
* @param language the value for the {@code language} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setLanguage(Language language) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Language.getType());
_value.setBoxed(language.handle());
addBuilderProperty("language", _value);
return (B) this;
}
/**
* Whether the font chooser dialog is modal.
*
* @param modal the value for the {@code modal} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setModal(boolean modal) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(modal);
addBuilderProperty("modal", _value);
return (B) this;
}
/**
* A title that may be shown on the font chooser
* dialog that is presented by {@link FontDialog#chooseFont}.
*
* @param title the value for the {@code title} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setTitle(String title) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(title);
addBuilderProperty("title", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_font_dialog_new = Interop.downcallHandle(
"gtk_font_dialog_new", FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_face = Interop.downcallHandle(
"gtk_font_dialog_choose_face", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_face_finish = Interop.downcallHandle(
"gtk_font_dialog_choose_face_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_family = Interop.downcallHandle(
"gtk_font_dialog_choose_family", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_family_finish = Interop.downcallHandle(
"gtk_font_dialog_choose_family_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_font = Interop.downcallHandle(
"gtk_font_dialog_choose_font", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_choose_font_and_features = Interop.downcallHandle(
"gtk_font_dialog_choose_font_and_features",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_font_dialog_choose_font_finish = Interop.downcallHandle(
"gtk_font_dialog_choose_font_finish", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_get_filter = Interop.downcallHandle(
"gtk_font_dialog_get_filter", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_get_font_map = Interop.downcallHandle(
"gtk_font_dialog_get_font_map", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_get_language = Interop.downcallHandle(
"gtk_font_dialog_get_language", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_get_modal = Interop.downcallHandle(
"gtk_font_dialog_get_modal", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_get_title = Interop.downcallHandle(
"gtk_font_dialog_get_title", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_set_filter = Interop.downcallHandle(
"gtk_font_dialog_set_filter", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_set_font_map = Interop.downcallHandle(
"gtk_font_dialog_set_font_map", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_set_language = Interop.downcallHandle(
"gtk_font_dialog_set_language", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_font_dialog_set_modal = Interop.downcallHandle(
"gtk_font_dialog_set_modal", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_font_dialog_set_title = Interop.downcallHandle(
"gtk_font_dialog_set_title", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}