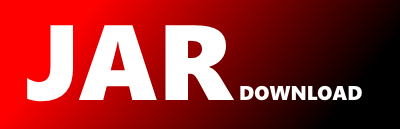
org.gnome.gtk.Image Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.Deprecated;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.gdk.Paintable;
import org.gnome.gdkpixbuf.Pixbuf;
import org.gnome.gio.Icon;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* The {@code GtkImage} widget displays an image.
*
*
*
* Various kinds of object can be displayed as an image; most typically,
* you would load a {@code GdkTexture} from a file, using the convenience function
* {@link Image#fromFile}, for instance:
*
{@code GtkWidget *image = gtk_image_new_from_file ("myfile.png");
* }
*
* If the file isn’t loaded successfully, the image will contain a
* “broken image” icon similar to that used in many web browsers.
*
* If you want to handle errors in loading the file yourself,
* for example by displaying an error message, then load the image with
* {@link org.gnome.gdk.Texture#fromFile}, then create the {@code GtkImage} with
* {@link Image#fromPaintable}.
*
* Sometimes an application will want to avoid depending on external data
* files, such as image files. See the documentation of {@code GResource} inside
* GIO, for details. In this case, {@code Gtk.Image:resource},
* {@link Image#fromResource}, and {@link Image#setFromResource}
* should be used.
*
* {@code GtkImage} displays its image as an icon, with a size that is determined
* by the application. See {@link Picture} if you want to show an image
* at is actual size.
*
* CSS nodes
* {@code GtkImage} has a single CSS node with the name {@code image}. The style classes
* {@code .normal-icons} or {@code .large-icons} may appear, depending on the
* {@code Gtk.Image:icon-size} property.
*
* Accessibility
* {@code GtkImage} uses the {@code GTK_ACCESSIBLE_ROLE_IMG} role.
*/
@Generated("io.github.jwharm.JavaGI")
public class Image extends Widget implements Accessible, Buildable, ConstraintTarget {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a Image proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public Image(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new empty {@code GtkImage} widget.
*/
public Image() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the Image class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_image_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected Image asParent() {
Image _parent = new Image(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Creates a new {@code GtkImage} displaying the file {@code filename}.
*
* If the file isn’t found or can’t be loaded, the resulting {@code GtkImage}
* will display a “broken image” icon. This function never returns {@code null},
* it always returns a valid {@code GtkImage} widget.
*
* If you need to detect failures to load the file, use
* {@link org.gnome.gdk.Texture#fromFile} to load the file yourself,
* then create the {@code GtkImage} from the texture.
*
* The storage type (see {@link Image#getStorageType})
* of the returned image is not defined, it will be whatever
* is appropriate for displaying the file.
*
* @param filename a filename
* @return a new {@code GtkImage}
*/
public static Image fromFile(String filename) {
var _result = constructFromFile(filename);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
private static MemorySegment constructFromFile(String filename) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_file.invokeExact(
(MemorySegment) (filename == null ? MemorySegment.NULL : Interop.allocateNativeString(filename, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Creates a {@code GtkImage} displaying an icon from the current icon theme.
*
* If the icon name isn’t known, a “broken image” icon will be
* displayed instead. If the current icon theme is changed, the icon
* will be updated appropriately.
*
* @param icon an icon
* @return a new {@code GtkImage} displaying the themed icon
*/
public static Image fromGicon(Icon icon) {
var _result = constructFromGicon(icon);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
private static MemorySegment constructFromGicon(Icon icon) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_gicon.invokeExact(
(MemorySegment) (icon == null ? MemorySegment.NULL : icon.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Creates a {@code GtkImage} displaying an icon from the current icon theme.
*
* If the icon name isn’t known, a “broken image” icon will be
* displayed instead. If the current icon theme is changed, the icon
* will be updated appropriately.
*
* @param iconName an icon name
* @return a new {@code GtkImage} displaying the themed icon
*/
public static Image fromIconName(@Nullable String iconName) {
var _result = constructFromIconName(iconName);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
private static MemorySegment constructFromIconName(@Nullable String iconName) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_icon_name.invokeExact(
(MemorySegment) (iconName == null ? MemorySegment.NULL : Interop.allocateNativeString(iconName, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Creates a new {@code GtkImage} displaying {@code paintable}.
*
* The {@code GtkImage} does not assume a reference to the paintable; you still
* need to unref it if you own references. {@code GtkImage} will add its own
* reference rather than adopting yours.
*
* The {@code GtkImage} will track changes to the {@code paintable} and update
* its size and contents in response to it.
*
* @param paintable a {@code GdkPaintable}
* @return a new {@code GtkImage}
*/
public static Image fromPaintable(@Nullable Paintable paintable) {
var _result = constructFromPaintable(paintable);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
private static MemorySegment constructFromPaintable(@Nullable Paintable paintable) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_paintable.invokeExact(
(MemorySegment) (paintable == null ? MemorySegment.NULL : paintable.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Creates a new {@code GtkImage} displaying {@code pixbuf}.
*
* The {@code GtkImage} does not assume a reference to the pixbuf; you still
* need to unref it if you own references. {@code GtkImage} will add its own
* reference rather than adopting yours.
*
* This is a helper for {@link Image#fromPaintable}, and you can't
* get back the exact pixbuf once this is called, only a texture.
*
* Note that this function just creates an {@code GtkImage} from the pixbuf.
* The {@code GtkImage} created will not react to state changes. Should you
* want that, you should use {@link Image#fromIconName}.
*
* @param pixbuf a {@code GdkPixbuf}
* @return a new {@code GtkImage}
* @deprecated Use {@link Image#fromPaintable} and
* {@link org.gnome.gdk.Texture#forPixbuf} instead
*/
@Deprecated
public static Image fromPixbuf(@Nullable Pixbuf pixbuf) {
var _result = constructFromPixbuf(pixbuf);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
@Deprecated
private static MemorySegment constructFromPixbuf(@Nullable Pixbuf pixbuf) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_pixbuf.invokeExact(
(MemorySegment) (pixbuf == null ? MemorySegment.NULL : pixbuf.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Creates a new {@code GtkImage} displaying the resource file {@code resourcePath}.
*
* If the file isn’t found or can’t be loaded, the resulting {@code GtkImage} will
* display a “broken image” icon. This function never returns {@code null},
* it always returns a valid {@code GtkImage} widget.
*
* If you need to detect failures to load the file, use
* {@link org.gnome.gdkpixbuf.Pixbuf#fromFile} to load the file yourself,
* then create the {@code GtkImage} from the pixbuf.
*
* The storage type (see {@link Image#getStorageType}) of
* the returned image is not defined, it will be whatever is
* appropriate for displaying the file.
*
* @param resourcePath a resource path
* @return a new {@code GtkImage}
*/
public static Image fromResource(String resourcePath) {
var _result = constructFromResource(resourcePath);
var _object = (Widget) InstanceCache.getForType(_result, Widget.WidgetImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.Image %ld", _gobject.handle().address());
_gobject.ref();
}
return (Image) _object;
}
private static MemorySegment constructFromResource(String resourcePath) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_new_from_resource.invokeExact(
(MemorySegment) (resourcePath == null ? MemorySegment.NULL : Interop.allocateNativeString(resourcePath, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Resets the image to be empty.
*/
public void clear() {
try {
MethodHandles.gtk_image_clear.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Gets the {@code GIcon} being displayed by the {@code GtkImage}.
*
* The storage type of the image must be {@link org.gnome.gtk.ImageType#EMPTY} or
* {@link org.gnome.gtk.ImageType#GICON} (see {@link Image#getStorageType}).
* The caller of this function does not own a reference to the
* returned {@code GIcon}.
*
* @return a {@code GIcon}
*/
public Icon getGicon() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_get_gicon.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (Icon) InstanceCache.getForType(_result, Icon.IconImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.Icon %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the icon name and size being displayed by the {@code GtkImage}.
*
* The storage type of the image must be {@link org.gnome.gtk.ImageType#EMPTY} or
* {@link org.gnome.gtk.ImageType#ICON_NAME} (see {@link Image#getStorageType}).
* The returned string is owned by the {@code GtkImage} and should not
* be freed.
*
* @return the icon name
*/
public String getIconName() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_get_icon_name.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the icon size used by the this Image when rendering icons.
*
* @return the image size used by icons
*/
public IconSize getIconSize() {
int _result;
try {
_result = (int) MethodHandles.gtk_image_get_icon_size.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return IconSize.of(_result);
}
/**
* Gets the image {@code GdkPaintable} being displayed by the {@code GtkImage}.
*
* The storage type of the image must be {@link org.gnome.gtk.ImageType#EMPTY} or
* {@link org.gnome.gtk.ImageType#PAINTABLE} (see {@link Image#getStorageType}).
* The caller of this function does not own a reference to the
* returned paintable.
*
* @return the displayed paintable
*/
public Paintable getPaintable() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_image_get_paintable.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _object = (Paintable) InstanceCache.getForType(_result, Paintable.PaintableImpl::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gdk.Paintable %ld", _gobject.handle().address());
_gobject.ref();
}
return _object;
}
/**
* Gets the pixel size used for named icons.
*
* @return the pixel size used for named icons.
*/
public int getPixelSize() {
int _result;
try {
_result = (int) MethodHandles.gtk_image_get_pixel_size.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the type of representation being used by the {@code GtkImage}
* to store image data.
*
* If the {@code GtkImage} has no image data, the return value will
* be {@link org.gnome.gtk.ImageType#EMPTY}.
*
* @return image representation being used
*/
public ImageType getStorageType() {
int _result;
try {
_result = (int) MethodHandles.gtk_image_get_storage_type.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return ImageType.of(_result);
}
/**
* Sets a {@code GtkImage} to show a file.
*
* See {@link Image#fromFile} for details.
*
* @param filename a filename
*/
public void setFromFile(@Nullable String filename) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_image_set_from_file.invokeExact(handle(),
(MemorySegment) (filename == null ? MemorySegment.NULL : Interop.allocateNativeString(filename, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets a {@code GtkImage} to show a {@code GIcon}.
*
* See {@link Image#fromGicon} for details.
*
* @param icon an icon
*/
public void setFromGicon(Icon icon) {
try {
MethodHandles.gtk_image_set_from_gicon.invokeExact(handle(),
(MemorySegment) (icon == null ? MemorySegment.NULL : icon.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets a {@code GtkImage} to show a named icon.
*
* See {@link Image#fromIconName} for details.
*
* @param iconName an icon name
*/
public void setFromIconName(@Nullable String iconName) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_image_set_from_icon_name.invokeExact(handle(),
(MemorySegment) (iconName == null ? MemorySegment.NULL : Interop.allocateNativeString(iconName, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets a {@code GtkImage} to show a {@code GdkPaintable}.
*
* See {@link Image#fromPaintable} for details.
*
* @param paintable a {@code GdkPaintable}
*/
public void setFromPaintable(@Nullable Paintable paintable) {
try {
MethodHandles.gtk_image_set_from_paintable.invokeExact(handle(),
(MemorySegment) (paintable == null ? MemorySegment.NULL : paintable.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets a {@code GtkImage} to show a {@code GdkPixbuf}.
*
* See {@link Image#fromPixbuf} for details.
*
* Note: This is a helper for {@link Image#setFromPaintable},
* and you can't get back the exact pixbuf once this is called,
* only a paintable.
*
* @param pixbuf a {@code GdkPixbuf} or {@code NULL}
* @deprecated Use {@link Image#setFromPaintable} instead
*/
@Deprecated
public void setFromPixbuf(@Nullable Pixbuf pixbuf) {
try {
MethodHandles.gtk_image_set_from_pixbuf.invokeExact(handle(),
(MemorySegment) (pixbuf == null ? MemorySegment.NULL : pixbuf.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets a {@code GtkImage} to show a resource.
*
* See {@link Image#fromResource} for details.
*
* @param resourcePath a resource path
*/
public void setFromResource(@Nullable String resourcePath) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_image_set_from_resource.invokeExact(handle(),
(MemorySegment) (resourcePath == null ? MemorySegment.NULL : Interop.allocateNativeString(resourcePath, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Suggests an icon size to the theme for named icons.
*
* @param iconSize the new icon size
*/
public void setIconSize(IconSize iconSize) {
try {
MethodHandles.gtk_image_set_icon_size.invokeExact(handle(), iconSize.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the pixel size to use for named icons.
*
* If the pixel size is set to a value != -1, it is used instead
* of the icon size set by {@link Image#setFromIconName}.
*
* @param pixelSize the new pixel size
*/
public void setPixelSize(int pixelSize) {
try {
MethodHandles.gtk_image_set_pixel_size.invokeExact(handle(), pixelSize);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code Image}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends Widget.Builder implements Accessible.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code Image} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code Image}.
*
* @return a new instance of {@code Image} with the properties
* that were set in the Builder object.
*/
public Image build() {
try {
var _instance = (Image) GObject.withProperties(Image.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* A path to the file to display.
*
* @param file the value for the {@code file} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFile(String file) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(file);
addBuilderProperty("file", _value);
return (B) this;
}
/**
* The {@code GIcon} displayed in the GtkImage.
*
* For themed icons, If the icon theme is changed, the image will be updated
* automatically.
*
* @param gicon the value for the {@code gicon} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setGicon(Icon gicon) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Icon.getType());
_value.setObject((GObject) gicon);
addBuilderProperty("gicon", _value);
return (B) this;
}
/**
* The name of the icon in the icon theme.
*
* If the icon theme is changed, the image will be updated automatically.
*
* @param iconName the value for the {@code icon-name} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setIconName(String iconName) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(iconName);
addBuilderProperty("icon-name", _value);
return (B) this;
}
/**
* The symbolic size to display icons at.
*
* @param iconSize the value for the {@code icon-size} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setIconSize(IconSize iconSize) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(IconSize.getType());
_value.setEnum(iconSize.getValue());
addBuilderProperty("icon-size", _value);
return (B) this;
}
/**
* The {@code GdkPaintable} to display.
*
* @param paintable the value for the {@code paintable} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setPaintable(Paintable paintable) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Paintable.getType());
_value.setObject((GObject) paintable);
addBuilderProperty("paintable", _value);
return (B) this;
}
/**
* The size in pixels to display icons at.
*
* If set to a value != -1, this property overrides the
* {@code Gtk.Image:icon-size} property for images of type
* {@code GTK_IMAGE_ICON_NAME}.
*
* @param pixelSize the value for the {@code pixel-size} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setPixelSize(int pixelSize) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.INT);
_value.setInt(pixelSize);
addBuilderProperty("pixel-size", _value);
return (B) this;
}
/**
* A path to a resource file to display.
*
* @param resource the value for the {@code resource} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setResource(String resource) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(resource);
addBuilderProperty("resource", _value);
return (B) this;
}
/**
* Whether the icon displayed in the {@code GtkImage} will use
* standard icon names fallback.
*
* The value of this property is only relevant for images of type
* {@link org.gnome.gtk.ImageType#ICON_NAME} and {@link org.gnome.gtk.ImageType#GICON}.
*
* @param useFallback the value for the {@code use-fallback} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setUseFallback(boolean useFallback) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(useFallback);
addBuilderProperty("use-fallback", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_image_new = Interop.downcallHandle("gtk_image_new",
FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_file = Interop.downcallHandle(
"gtk_image_new_from_file", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_gicon = Interop.downcallHandle(
"gtk_image_new_from_gicon", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_icon_name = Interop.downcallHandle(
"gtk_image_new_from_icon_name", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_paintable = Interop.downcallHandle(
"gtk_image_new_from_paintable", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_pixbuf = Interop.downcallHandle(
"gtk_image_new_from_pixbuf", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_new_from_resource = Interop.downcallHandle(
"gtk_image_new_from_resource", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_clear = Interop.downcallHandle("gtk_image_clear",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_gicon = Interop.downcallHandle(
"gtk_image_get_gicon", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_icon_name = Interop.downcallHandle(
"gtk_image_get_icon_name", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_icon_size = Interop.downcallHandle(
"gtk_image_get_icon_size", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_paintable = Interop.downcallHandle(
"gtk_image_get_paintable", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_pixel_size = Interop.downcallHandle(
"gtk_image_get_pixel_size", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_get_storage_type = Interop.downcallHandle(
"gtk_image_get_storage_type", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_file = Interop.downcallHandle(
"gtk_image_set_from_file", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_gicon = Interop.downcallHandle(
"gtk_image_set_from_gicon", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_icon_name = Interop.downcallHandle(
"gtk_image_set_from_icon_name", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_paintable = Interop.downcallHandle(
"gtk_image_set_from_paintable", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_pixbuf = Interop.downcallHandle(
"gtk_image_set_from_pixbuf", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_from_resource = Interop.downcallHandle(
"gtk_image_set_from_resource", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_image_set_icon_size = Interop.downcallHandle(
"gtk_image_set_icon_size", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_image_set_pixel_size = Interop.downcallHandle(
"gtk_image_set_pixel_size", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
}
}