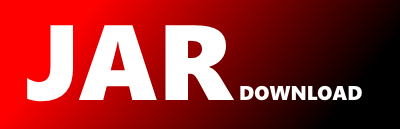
org.gnome.gtk.License Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.Enumeration;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.IllegalStateException;
import java.lang.Override;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
/**
* The type of license for an application.
*
* This enumeration can be expanded at later date.
*/
@Generated("io.github.jwharm.JavaGI")
public enum License implements Enumeration {
/**
* No license specified
*/
UNKNOWN(0),
/**
* A license text is going to be specified by the
* developer
*/
CUSTOM(1),
/**
* The GNU General Public License, version 2.0 or later
*/
GPL_2_0(2),
/**
* The GNU General Public License, version 3.0 or later
*/
GPL_3_0(3),
/**
* The GNU Lesser General Public License, version 2.1 or later
*/
LGPL_2_1(4),
/**
* The GNU Lesser General Public License, version 3.0 or later
*/
LGPL_3_0(5),
/**
* The BSD standard license
*/
BSD(6),
/**
* The MIT/X11 standard license
*/
MIT_X11(7),
/**
* The Artistic License, version 2.0
*/
ARTISTIC(8),
/**
* The GNU General Public License, version 2.0 only
*/
GPL_2_0_ONLY(9),
/**
* The GNU General Public License, version 3.0 only
*/
GPL_3_0_ONLY(10),
/**
* The GNU Lesser General Public License, version 2.1 only
*/
LGPL_2_1_ONLY(11),
/**
* The GNU Lesser General Public License, version 3.0 only
*/
LGPL_3_0_ONLY(12),
/**
* The GNU Affero General Public License, version 3.0 or later
*/
AGPL_3_0(13),
/**
* The GNU Affero General Public License, version 3.0 only
*/
AGPL_3_0_ONLY(14),
/**
* The 3-clause BSD licence
*/
BSD_3(15),
/**
* The Apache License, version 2.0
*/
APACHE_2_0(16),
/**
* The Mozilla Public License, version 2.0
*/
MPL_2_0(17),
/**
* Zero-Clause BSD license
*/
_0BSD(18);
static {
Gtk.javagi$ensureInitialized();
}
private final int value;
/**
* Create a new License for the provided value
*
* @param value the enum value
*/
private License(int value) {
this.value = value;
}
/**
* Create a new License for the provided value
*
* @param value the enum value
* @return the enum for the provided value
*/
public static License of(int value) {
return switch(value) {
case 0 -> UNKNOWN;
case 1 -> CUSTOM;
case 2 -> GPL_2_0;
case 3 -> GPL_3_0;
case 4 -> LGPL_2_1;
case 5 -> LGPL_3_0;
case 6 -> BSD;
case 7 -> MIT_X11;
case 8 -> ARTISTIC;
case 9 -> GPL_2_0_ONLY;
case 10 -> GPL_3_0_ONLY;
case 11 -> LGPL_2_1_ONLY;
case 12 -> LGPL_3_0_ONLY;
case 13 -> AGPL_3_0;
case 14 -> AGPL_3_0_ONLY;
case 15 -> BSD_3;
case 16 -> APACHE_2_0;
case 17 -> MPL_2_0;
case 18 -> _0BSD;
default -> throw new IllegalStateException("Unexpected value: " + value);
} ;
}
/**
* Get the numeric value of this enum
*
* @return the enum value
*/
@Override
public int getValue() {
return value;
}
/**
* Get the GType of the License class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_license_get_type");
}
}