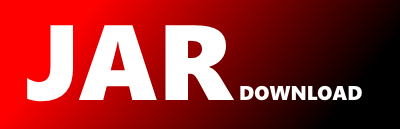
org.gnome.gtk.PrintSettings Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.base.GErrorException;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.base.Out;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.Integer;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.KeyFile;
import org.gnome.glib.Type;
import org.gnome.glib.Variant;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* A {@code GtkPrintSettings} object represents the settings of a print dialog in
* a system-independent way.
*
* The main use for this object is that once you’ve printed you can get a
* settings object that represents the settings the user chose, and the next
* time you print you can pass that object in so that the user doesn’t have
* to re-set all his settings.
*
* Its also possible to enumerate the settings so that you can easily save
* the settings for the next time your app runs, or even store them in a
* document. The predefined keys try to use shared values as much as possible
* so that moving such a document between systems still works.
*/
@Generated("io.github.jwharm.JavaGI")
public class PrintSettings extends GObject {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a PrintSettings proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public PrintSettings(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new {@code GtkPrintSettings} object.
*/
public PrintSettings() {
this(constructNew());
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the PrintSettings class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_print_settings_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected PrintSettings asParent() {
PrintSettings _parent = new PrintSettings(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_new.invokeExact();
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Reads the print settings from {@code fileName}.
*
* Returns a new {@code GtkPrintSettings} object with the restored settings,
* or {@code null} if an error occurred. If the file could not be loaded then
* error is set to either a {@code GFileError} or {@code GKeyFileError}.
*
* See {@link PrintSettings#toFile}.
*
* @param fileName the filename to read the settings from
* @return the restored {@code GtkPrintSettings}
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public static PrintSettings fromFile(String fileName) throws GErrorException {
var _result = constructFromFile(fileName);
var _object = (PrintSettings) InstanceCache.getForType(_result, PrintSettings::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.PrintSettings %ld", _gobject.handle().address());
_gobject.ref();
}
return (PrintSettings) _object;
}
private static MemorySegment constructFromFile(String fileName) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_new_from_file.invokeExact(
(MemorySegment) (fileName == null ? MemorySegment.NULL : Interop.allocateNativeString(fileName, _arena)), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result;
}
}
/**
* Deserialize print settings from an a{sv} variant.
*
* The variant must be in the format produced by
* {@link PrintSettings#toGvariant}.
*
* @param variant an a{sv} {@code GVariant}
* @return a new {@code GtkPrintSettings} object
*/
public static PrintSettings fromGvariant(Variant variant) {
var _result = constructFromGvariant(variant);
var _object = (PrintSettings) InstanceCache.getForType(_result, PrintSettings::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.PrintSettings %ld", _gobject.handle().address());
_gobject.ref();
}
return (PrintSettings) _object;
}
private static MemorySegment constructFromGvariant(Variant variant) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_new_from_gvariant.invokeExact(
(MemorySegment) (variant == null ? MemorySegment.NULL : variant.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Reads the print settings from the group {@code groupName} in {@code keyFile}.
*
* Returns a new {@code GtkPrintSettings} object with the restored settings,
* or {@code null} if an error occurred. If the file could not be loaded then
* error is set to either {@code GFileError} or {@code GKeyFileError}.
*
* @param keyFile the {@code GKeyFile} to retrieve the settings from
* @param groupName the name of the group to use, or {@code null} to use
* the default “Print Settings”
* @return the restored {@code GtkPrintSettings}
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public static PrintSettings fromKeyFile(KeyFile keyFile, @Nullable String groupName) throws
GErrorException {
var _result = constructFromKeyFile(keyFile, groupName);
var _object = (PrintSettings) InstanceCache.getForType(_result, PrintSettings::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gtk.PrintSettings %ld", _gobject.handle().address());
_gobject.ref();
}
return (PrintSettings) _object;
}
private static MemorySegment constructFromKeyFile(KeyFile keyFile, @Nullable String groupName)
throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_new_from_key_file.invokeExact(
(MemorySegment) (keyFile == null ? MemorySegment.NULL : keyFile.handle()),
(MemorySegment) (groupName == null ? MemorySegment.NULL : Interop.allocateNativeString(groupName, _arena)), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result;
}
}
/**
* Copies a {@code GtkPrintSettings} object.
*
* @return a newly allocated copy of this PrintSettings
*/
public PrintSettings copy() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_copy.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return (PrintSettings) InstanceCache.getForType(_result, PrintSettings::new, true);
}
/**
* Calls {@code func} for each key-value pair of this PrintSettings.
*
* @param func the function to call
*/
public void foreach(PrintSettingsFunc func) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_foreach.invokeExact(handle(),
(MemorySegment) (func == null ? MemorySegment.NULL : func.toCallback(_arena)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Looks up the string value associated with {@code key}.
*
* @param key a key
* @return the string value for {@code key}
*/
public String getString(String key) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get.invokeExact(
handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
}
/**
* Returns the boolean represented by the value
* that is associated with {@code key}.
*
* The string “true” represents {@code true}, any other
* string {@code false}.
*
* @param key a key
* @return {@code true}, if {@code key} maps to a true value.
*/
public boolean getBool(String key) {
try (var _arena = Arena.ofConfined()) {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_bool.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_COLLATE}.
*
* @return whether to collate the printed pages
*/
public boolean getCollate() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_collate.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_DEFAULT_SOURCE}.
*
* @return the default source
*/
public String getDefaultSource() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_default_source.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_DITHER}.
*
* @return the dithering that is used
*/
public String getDither() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_dither.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Returns the double value associated with {@code key}, or 0.
*
* @param key a key
* @return the double value of {@code key}
*/
public double getDouble(String key) {
try (var _arena = Arena.ofConfined()) {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_double.invokeExact(
handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Returns the floating point number represented by
* the value that is associated with {@code key}, or {@code defaultVal}
* if the value does not represent a floating point number.
*
* Floating point numbers are parsed with g_ascii_strtod().
*
* @param key a key
* @param def the default value
* @return the floating point number associated with {@code key}
*/
public double getDoubleWithDefault(String key, double def) {
try (var _arena = Arena.ofConfined()) {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_double_with_default.invokeExact(
handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
def);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_DUPLEX}.
*
* @return whether to print the output in duplex.
*/
public PrintDuplex getDuplex() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_duplex.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return PrintDuplex.of(_result);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_FINISHINGS}.
*
* @return the finishings
*/
public String getFinishings() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_finishings.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Returns the integer value of {@code key}, or 0.
*
* @param key a key
* @return the integer value of {@code key}
*/
public int getInt(String key) {
try (var _arena = Arena.ofConfined()) {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_int.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Returns the value of {@code key}, interpreted as
* an integer, or the default value.
*
* @param key a key
* @param def the default value
* @return the integer value of {@code key}
*/
public int getIntWithDefault(String key, int def) {
try (var _arena = Arena.ofConfined()) {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_int_with_default.invokeExact(
handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
def);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Returns the value associated with {@code key}, interpreted
* as a length.
*
* The returned value is converted to {@code units}.
*
* @param key a key
* @param unit the unit of the return value
* @return the length value of {@code key}, converted to {@code unit}
*/
public double getLength(String key, Unit unit) {
try (var _arena = Arena.ofConfined()) {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_length.invokeExact(
handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_MEDIA_TYPE}.
*
* The set of media types is defined in PWG 5101.1-2002 PWG.
*
* @return the media type
*/
public String getMediaType() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_media_type.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_N_COPIES}.
*
* @return the number of copies to print
*/
public int getNCopies() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_n_copies.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_NUMBER_UP}.
*
* @return the number of pages per sheet
*/
public int getNumberUp() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_number_up.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_NUMBER_UP_LAYOUT}.
*
* @return layout of page in number-up mode
*/
public NumberUpLayout getNumberUpLayout() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_number_up_layout.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return NumberUpLayout.of(_result);
}
/**
* Get the value of {@code GTK_PRINT_SETTINGS_ORIENTATION},
* converted to a {@code GtkPageOrientation}.
*
* @return the orientation
*/
public PageOrientation getOrientation() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_orientation.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return PageOrientation.of(_result);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_OUTPUT_BIN}.
*
* @return the output bin
*/
public String getOutputBin() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_output_bin.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PAGE_RANGES}.
*
* @return an array
* of {@code GtkPageRange}s. Use g_free() to free the array when
* it is no longer needed.
*/
public PageRange[] getPageRanges() {
try (var _arena = Arena.ofConfined()) {
MemorySegment _numRangesPointer = _arena.allocate(ValueLayout.JAVA_INT);
_numRangesPointer.set(ValueLayout.JAVA_INT, 0L, 0);
Out numRanges = new Out<>();
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_page_ranges.invokeExact(
handle(), _numRangesPointer);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
numRanges.set(_numRangesPointer.get(ValueLayout.JAVA_INT, 0));
return Interop.getStructArrayFrom(_result, (int) numRanges.get().intValue(), PageRange.class, PageRange::new, PageRange.getMemoryLayout());
}
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PAGE_SET}.
*
* @return the set of pages to print
*/
public PageSet getPageSet() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_page_set.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return PageSet.of(_result);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PAPER_HEIGHT},
* converted to {@code unit}.
*
* @param unit the unit for the return value
* @return the paper height, in units of {@code unit}
*/
public double getPaperHeight(Unit unit) {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_paper_height.invokeExact(
handle(), unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PAPER_FORMAT},
* converted to a {@code GtkPaperSize}.
*
* @return the paper size
*/
public PaperSize getPaperSize() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_paper_size.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new PaperSize(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, PaperSize.getType());
}
return _instance;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PAPER_WIDTH},
* converted to {@code unit}.
*
* @param unit the unit for the return value
* @return the paper width, in units of {@code unit}
*/
public double getPaperWidth(Unit unit) {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_paper_width.invokeExact(
handle(), unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PRINT_PAGES}.
*
* @return which pages to print
*/
public PrintPages getPrintPages() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_print_pages.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return PrintPages.of(_result);
}
/**
* Convenience function to obtain the value of
* {@code GTK_PRINT_SETTINGS_PRINTER}.
*
* @return the printer name
*/
public String getPrinter() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_get_printer.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_PRINTER_LPI}.
*
* @return the resolution in lpi (lines per inch)
*/
public double getPrinterLpi() {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_printer_lpi.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_QUALITY}.
*
* @return the print quality
*/
public PrintQuality getQuality() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_quality.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return PrintQuality.of(_result);
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_RESOLUTION}.
*
* @return the resolution in dpi
*/
public int getResolution() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_resolution.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_RESOLUTION_X}.
*
* @return the horizontal resolution in dpi
*/
public int getResolutionX() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_resolution_x.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_RESOLUTION_Y}.
*
* @return the vertical resolution in dpi
*/
public int getResolutionY() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_resolution_y.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_REVERSE}.
*
* @return whether to reverse the order of the printed pages
*/
public boolean getReverse() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_reverse.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_SCALE}.
*
* @return the scale in percent
*/
public double getScale() {
double _result;
try {
_result = (double) MethodHandles.gtk_print_settings_get_scale.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Gets the value of {@code GTK_PRINT_SETTINGS_USE_COLOR}.
*
* @return whether to use color
*/
public boolean getUseColor() {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_get_use_color.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Returns {@code true}, if a value is associated with {@code key}.
*
* @param key a key
* @return {@code true}, if {@code key} has a value
*/
public boolean hasKey(String key) {
try (var _arena = Arena.ofConfined()) {
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_has_key.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
}
/**
* Reads the print settings from {@code fileName}.
*
* If the file could not be loaded then error is set to either
* a {@code GFileError} or {@code GKeyFileError}.
*
* See {@link PrintSettings#toFile}.
*
* @param fileName the filename to read the settings from
* @return {@code true} on success
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean loadFile(String fileName) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_load_file.invokeExact(handle(),
(MemorySegment) (fileName == null ? MemorySegment.NULL : Interop.allocateNativeString(fileName, _arena)), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Reads the print settings from the group {@code groupName} in {@code keyFile}.
*
* If the file could not be loaded then error is set to either a
* {@code GFileError} or {@code GKeyFileError}.
*
* @param keyFile the {@code GKeyFile} to retrieve the settings from
* @param groupName the name of the group to use, or {@code null}
* to use the default “Print Settings”
* @return {@code true} on success
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean loadKeyFile(KeyFile keyFile, @Nullable String groupName) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_load_key_file.invokeExact(
handle(),
(MemorySegment) (keyFile == null ? MemorySegment.NULL : keyFile.handle()),
(MemorySegment) (groupName == null ? MemorySegment.NULL : Interop.allocateNativeString(groupName, _arena)), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Associates {@code value} with {@code key}.
*
* @param key a key
* @param value a string value
*/
public void set(String key, @Nullable String value) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
(MemorySegment) (value == null ? MemorySegment.NULL : Interop.allocateNativeString(value, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets {@code key} to a boolean value.
*
* @param key a key
* @param value a boolean
*/
public void setBool(String key, boolean value) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_bool.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
value ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_COLLATE}.
*
* @param collate whether to collate the output
*/
public void setCollate(boolean collate) {
try {
MethodHandles.gtk_print_settings_set_collate.invokeExact(handle(), collate ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_DEFAULT_SOURCE}.
*
* @param defaultSource the default source
*/
public void setDefaultSource(String defaultSource) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_default_source.invokeExact(handle(),
(MemorySegment) (defaultSource == null ? MemorySegment.NULL : Interop.allocateNativeString(defaultSource, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_DITHER}.
*
* @param dither the dithering that is used
*/
public void setDither(String dither) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_dither.invokeExact(handle(),
(MemorySegment) (dither == null ? MemorySegment.NULL : Interop.allocateNativeString(dither, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets {@code key} to a double value.
*
* @param key a key
* @param value a double value
*/
public void setDouble(String key, double value) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_double.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
value);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_DUPLEX}.
*
* @param duplex a {@code GtkPrintDuplex} value
*/
public void setDuplex(PrintDuplex duplex) {
try {
MethodHandles.gtk_print_settings_set_duplex.invokeExact(handle(), duplex.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_FINISHINGS}.
*
* @param finishings the finishings
*/
public void setFinishings(String finishings) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_finishings.invokeExact(handle(),
(MemorySegment) (finishings == null ? MemorySegment.NULL : Interop.allocateNativeString(finishings, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets {@code key} to an integer value.
*
* @param key a key
* @param value an integer
*/
public void setInt(String key, int value) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_int.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
value);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Associates a length in units of {@code unit} with {@code key}.
*
* @param key a key
* @param value a length
* @param unit the unit of {@code length}
*/
public void setLength(String key, double value, Unit unit) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_length.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)),
value, unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_MEDIA_TYPE}.
*
* The set of media types is defined in PWG 5101.1-2002 PWG.
*
* @param mediaType the media type
*/
public void setMediaType(String mediaType) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_media_type.invokeExact(handle(),
(MemorySegment) (mediaType == null ? MemorySegment.NULL : Interop.allocateNativeString(mediaType, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_N_COPIES}.
*
* @param numCopies the number of copies
*/
public void setNCopies(int numCopies) {
try {
MethodHandles.gtk_print_settings_set_n_copies.invokeExact(handle(), numCopies);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_NUMBER_UP}.
*
* @param numberUp the number of pages per sheet
*/
public void setNumberUp(int numberUp) {
try {
MethodHandles.gtk_print_settings_set_number_up.invokeExact(handle(), numberUp);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_NUMBER_UP_LAYOUT}.
*
* @param numberUpLayout a {@code GtkNumberUpLayout} value
*/
public void setNumberUpLayout(NumberUpLayout numberUpLayout) {
try {
MethodHandles.gtk_print_settings_set_number_up_layout.invokeExact(handle(),
numberUpLayout.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_ORIENTATION}.
*
* @param orientation a page orientation
*/
public void setOrientation(PageOrientation orientation) {
try {
MethodHandles.gtk_print_settings_set_orientation.invokeExact(handle(),
orientation.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_OUTPUT_BIN}.
*
* @param outputBin the output bin
*/
public void setOutputBin(String outputBin) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_output_bin.invokeExact(handle(),
(MemorySegment) (outputBin == null ? MemorySegment.NULL : Interop.allocateNativeString(outputBin, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PAGE_RANGES}.
*
* @param pageRanges an array of {@code GtkPageRange}s
*/
public void setPageRanges(PageRange[] pageRanges) {
try (var _arena = Arena.ofConfined()) {
int numRanges = pageRanges == null ? 0 : pageRanges.length;
try {
MethodHandles.gtk_print_settings_set_page_ranges.invokeExact(handle(),
(MemorySegment) (pageRanges == null ? MemorySegment.NULL : Interop.allocateNativeArray(pageRanges, PageRange.getMemoryLayout(), false, _arena)),
numRanges);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PAGE_SET}.
*
* @param pageSet a {@code GtkPageSet} value
*/
public void setPageSet(PageSet pageSet) {
try {
MethodHandles.gtk_print_settings_set_page_set.invokeExact(handle(), pageSet.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PAPER_HEIGHT}.
*
* @param height the paper height
* @param unit the units of {@code height}
*/
public void setPaperHeight(double height, Unit unit) {
try {
MethodHandles.gtk_print_settings_set_paper_height.invokeExact(handle(), height,
unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PAPER_FORMAT},
* {@code GTK_PRINT_SETTINGS_PAPER_WIDTH} and
* {@code GTK_PRINT_SETTINGS_PAPER_HEIGHT}.
*
* @param paperSize a paper size
*/
public void setPaperSize(PaperSize paperSize) {
try {
MethodHandles.gtk_print_settings_set_paper_size.invokeExact(handle(),
(MemorySegment) (paperSize == null ? MemorySegment.NULL : paperSize.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PAPER_WIDTH}.
*
* @param width the paper width
* @param unit the units of {@code width}
*/
public void setPaperWidth(double width, Unit unit) {
try {
MethodHandles.gtk_print_settings_set_paper_width.invokeExact(handle(), width,
unit.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PRINT_PAGES}.
*
* @param pages a {@code GtkPrintPages} value
*/
public void setPrintPages(PrintPages pages) {
try {
MethodHandles.gtk_print_settings_set_print_pages.invokeExact(handle(),
pages.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Convenience function to set {@code GTK_PRINT_SETTINGS_PRINTER}
* to {@code printer}.
*
* @param printer the printer name
*/
public void setPrinter(String printer) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_set_printer.invokeExact(handle(),
(MemorySegment) (printer == null ? MemorySegment.NULL : Interop.allocateNativeString(printer, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_PRINTER_LPI}.
*
* @param lpi the resolution in lpi (lines per inch)
*/
public void setPrinterLpi(double lpi) {
try {
MethodHandles.gtk_print_settings_set_printer_lpi.invokeExact(handle(), lpi);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_QUALITY}.
*
* @param quality a {@code GtkPrintQuality} value
*/
public void setQuality(PrintQuality quality) {
try {
MethodHandles.gtk_print_settings_set_quality.invokeExact(handle(), quality.getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the values of {@code GTK_PRINT_SETTINGS_RESOLUTION},
* {@code GTK_PRINT_SETTINGS_RESOLUTION_X} and
* {@code GTK_PRINT_SETTINGS_RESOLUTION_Y}.
*
* @param resolution the resolution in dpi
*/
public void setResolution(int resolution) {
try {
MethodHandles.gtk_print_settings_set_resolution.invokeExact(handle(), resolution);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the values of {@code GTK_PRINT_SETTINGS_RESOLUTION},
* {@code GTK_PRINT_SETTINGS_RESOLUTION_X} and
* {@code GTK_PRINT_SETTINGS_RESOLUTION_Y}.
*
* @param resolutionX the horizontal resolution in dpi
* @param resolutionY the vertical resolution in dpi
*/
public void setResolutionXy(int resolutionX, int resolutionY) {
try {
MethodHandles.gtk_print_settings_set_resolution_xy.invokeExact(handle(), resolutionX,
resolutionY);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_REVERSE}.
*
* @param reverse whether to reverse the output
*/
public void setReverse(boolean reverse) {
try {
MethodHandles.gtk_print_settings_set_reverse.invokeExact(handle(), reverse ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_SCALE}.
*
* @param scale the scale in percent
*/
public void setScale(double scale) {
try {
MethodHandles.gtk_print_settings_set_scale.invokeExact(handle(), scale);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the value of {@code GTK_PRINT_SETTINGS_USE_COLOR}.
*
* @param useColor whether to use color
*/
public void setUseColor(boolean useColor) {
try {
MethodHandles.gtk_print_settings_set_use_color.invokeExact(handle(), useColor ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* This function saves the print settings from this PrintSettings to {@code fileName}.
*
* If the file could not be written then error is set to either a
* {@code GFileError} or {@code GKeyFileError}.
*
* @param fileName the file to save to
* @return {@code true} on success
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean toFile(String fileName) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.gtk_print_settings_to_file.invokeExact(handle(),
(MemorySegment) (fileName == null ? MemorySegment.NULL : Interop.allocateNativeString(fileName, _arena)), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Serialize print settings to an a{sv} variant.
*
* @return a new, floating, {@code GVariant}
*/
public Variant toGvariant() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gtk_print_settings_to_gvariant.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return MemorySegment.NULL.equals(_result) ? null : new Variant(_result);
}
/**
* This function adds the print settings from this PrintSettings to {@code keyFile}.
*
* @param keyFile the {@code GKeyFile} to save the print settings to
* @param groupName the group to add the settings to in {@code keyFile}, or
* {@code null} to use the default “Print Settings”
*/
public void toKeyFile(KeyFile keyFile, @Nullable String groupName) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_to_key_file.invokeExact(handle(),
(MemorySegment) (keyFile == null ? MemorySegment.NULL : keyFile.handle()),
(MemorySegment) (groupName == null ? MemorySegment.NULL : Interop.allocateNativeString(groupName, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Removes any value associated with {@code key}.
*
* This has the same effect as setting the value to {@code null}.
*
* @param key a key
*/
public void unset(String key) {
try (var _arena = Arena.ofConfined()) {
try {
MethodHandles.gtk_print_settings_unset.invokeExact(handle(),
(MemorySegment) (key == null ? MemorySegment.NULL : Interop.allocateNativeString(key, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* A {@link Builder} object constructs a {@code PrintSettings}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code PrintSettings} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code PrintSettings}.
*
* @return a new instance of {@code PrintSettings} with the properties
* that were set in the Builder object.
*/
public PrintSettings build() {
try {
var _instance = (PrintSettings) GObject.withProperties(PrintSettings.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
}
private static final class MethodHandles {
static final MethodHandle gtk_print_settings_new = Interop.downcallHandle(
"gtk_print_settings_new", FunctionDescriptor.of(ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_new_from_file = Interop.downcallHandle(
"gtk_print_settings_new_from_file", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_new_from_gvariant = Interop.downcallHandle(
"gtk_print_settings_new_from_gvariant", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_new_from_key_file = Interop.downcallHandle(
"gtk_print_settings_new_from_key_file", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_copy = Interop.downcallHandle(
"gtk_print_settings_copy", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_foreach = Interop.downcallHandle(
"gtk_print_settings_foreach", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get = Interop.downcallHandle(
"gtk_print_settings_get", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_bool = Interop.downcallHandle(
"gtk_print_settings_get_bool", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_collate = Interop.downcallHandle(
"gtk_print_settings_get_collate", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_default_source = Interop.downcallHandle(
"gtk_print_settings_get_default_source", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_dither = Interop.downcallHandle(
"gtk_print_settings_get_dither", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_double = Interop.downcallHandle(
"gtk_print_settings_get_double", FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_double_with_default = Interop.downcallHandle(
"gtk_print_settings_get_double_with_default",
FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_DOUBLE), false);
static final MethodHandle gtk_print_settings_get_duplex = Interop.downcallHandle(
"gtk_print_settings_get_duplex", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_finishings = Interop.downcallHandle(
"gtk_print_settings_get_finishings", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_int = Interop.downcallHandle(
"gtk_print_settings_get_int", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_int_with_default = Interop.downcallHandle(
"gtk_print_settings_get_int_with_default",
FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_get_length = Interop.downcallHandle(
"gtk_print_settings_get_length", FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_get_media_type = Interop.downcallHandle(
"gtk_print_settings_get_media_type", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_n_copies = Interop.downcallHandle(
"gtk_print_settings_get_n_copies", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_number_up = Interop.downcallHandle(
"gtk_print_settings_get_number_up", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_number_up_layout = Interop.downcallHandle(
"gtk_print_settings_get_number_up_layout",
FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_orientation = Interop.downcallHandle(
"gtk_print_settings_get_orientation", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_output_bin = Interop.downcallHandle(
"gtk_print_settings_get_output_bin", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_page_ranges = Interop.downcallHandle(
"gtk_print_settings_get_page_ranges", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_page_set = Interop.downcallHandle(
"gtk_print_settings_get_page_set", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_paper_height = Interop.downcallHandle(
"gtk_print_settings_get_paper_height",
FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE, ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_get_paper_size = Interop.downcallHandle(
"gtk_print_settings_get_paper_size", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_paper_width = Interop.downcallHandle(
"gtk_print_settings_get_paper_width", FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_get_print_pages = Interop.downcallHandle(
"gtk_print_settings_get_print_pages", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_printer = Interop.downcallHandle(
"gtk_print_settings_get_printer", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_printer_lpi = Interop.downcallHandle(
"gtk_print_settings_get_printer_lpi", FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_quality = Interop.downcallHandle(
"gtk_print_settings_get_quality", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_resolution = Interop.downcallHandle(
"gtk_print_settings_get_resolution", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_resolution_x = Interop.downcallHandle(
"gtk_print_settings_get_resolution_x", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_resolution_y = Interop.downcallHandle(
"gtk_print_settings_get_resolution_y", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_reverse = Interop.downcallHandle(
"gtk_print_settings_get_reverse", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_scale = Interop.downcallHandle(
"gtk_print_settings_get_scale", FunctionDescriptor.of(ValueLayout.JAVA_DOUBLE,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_get_use_color = Interop.downcallHandle(
"gtk_print_settings_get_use_color", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_has_key = Interop.downcallHandle(
"gtk_print_settings_has_key", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_load_file = Interop.downcallHandle(
"gtk_print_settings_load_file", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_load_key_file = Interop.downcallHandle(
"gtk_print_settings_load_key_file", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS),
false);
static final MethodHandle gtk_print_settings_set = Interop.downcallHandle(
"gtk_print_settings_set", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_bool = Interop.downcallHandle(
"gtk_print_settings_set_bool", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_collate = Interop.downcallHandle(
"gtk_print_settings_set_collate", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_default_source = Interop.downcallHandle(
"gtk_print_settings_set_default_source",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_dither = Interop.downcallHandle(
"gtk_print_settings_set_dither", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_double = Interop.downcallHandle(
"gtk_print_settings_set_double", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_DOUBLE), false);
static final MethodHandle gtk_print_settings_set_duplex = Interop.downcallHandle(
"gtk_print_settings_set_duplex", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_finishings = Interop.downcallHandle(
"gtk_print_settings_set_finishings", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_int = Interop.downcallHandle(
"gtk_print_settings_set_int", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_length = Interop.downcallHandle(
"gtk_print_settings_set_length", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_DOUBLE, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_media_type = Interop.downcallHandle(
"gtk_print_settings_set_media_type", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_n_copies = Interop.downcallHandle(
"gtk_print_settings_set_n_copies", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_number_up = Interop.downcallHandle(
"gtk_print_settings_set_number_up", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_number_up_layout = Interop.downcallHandle(
"gtk_print_settings_set_number_up_layout",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_orientation = Interop.downcallHandle(
"gtk_print_settings_set_orientation", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_output_bin = Interop.downcallHandle(
"gtk_print_settings_set_output_bin", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_page_ranges = Interop.downcallHandle(
"gtk_print_settings_set_page_ranges", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_page_set = Interop.downcallHandle(
"gtk_print_settings_set_page_set", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_paper_height = Interop.downcallHandle(
"gtk_print_settings_set_paper_height",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.JAVA_DOUBLE,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_paper_size = Interop.downcallHandle(
"gtk_print_settings_set_paper_size", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_paper_width = Interop.downcallHandle(
"gtk_print_settings_set_paper_width", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_DOUBLE, ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_print_pages = Interop.downcallHandle(
"gtk_print_settings_set_print_pages", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_printer = Interop.downcallHandle(
"gtk_print_settings_set_printer", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_set_printer_lpi = Interop.downcallHandle(
"gtk_print_settings_set_printer_lpi", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_DOUBLE), false);
static final MethodHandle gtk_print_settings_set_quality = Interop.downcallHandle(
"gtk_print_settings_set_quality", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_resolution = Interop.downcallHandle(
"gtk_print_settings_set_resolution", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_resolution_xy = Interop.downcallHandle(
"gtk_print_settings_set_resolution_xy",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.JAVA_INT,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_reverse = Interop.downcallHandle(
"gtk_print_settings_set_reverse", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_set_scale = Interop.downcallHandle(
"gtk_print_settings_set_scale", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_DOUBLE), false);
static final MethodHandle gtk_print_settings_set_use_color = Interop.downcallHandle(
"gtk_print_settings_set_use_color", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle gtk_print_settings_to_file = Interop.downcallHandle(
"gtk_print_settings_to_file", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_to_gvariant = Interop.downcallHandle(
"gtk_print_settings_to_gvariant", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_to_key_file = Interop.downcallHandle(
"gtk_print_settings_to_key_file", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle gtk_print_settings_unset = Interop.downcallHandle(
"gtk_print_settings_unset", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}