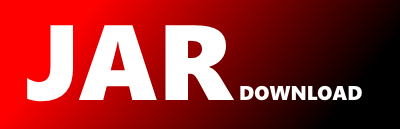
org.gnome.gtk.ShortcutsGroup Maven / Gradle / Ivy
Show all versions of gtk Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gtk;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.TypeClass;
import org.gnome.gobject.Value;
/**
* A {@code GtkShortcutsGroup} represents a group of related keyboard shortcuts
* or gestures.
*
* The group has a title. It may optionally be associated with a view
* of the application, which can be used to show only relevant shortcuts
* depending on the application context.
*
* This widget is only meant to be used with {@link ShortcutsWindow}.
*
* The recommended way to construct a {@code GtkShortcutsGroup} is with
* {@link GtkBuilder}, by using the {@code } tag to populate a
* {@code GtkShortcutsGroup} with one or more {@link ShortcutsShortcut}
* instances.
*
* If you need to add a shortcut programmatically, use
* {@link ShortcutsGroup#addShortcut}.
*/
@Generated("io.github.jwharm.JavaGI")
public class ShortcutsGroup extends Box implements Accessible, Buildable, ConstraintTarget, Orientable {
static {
Gtk.javagi$ensureInitialized();
}
/**
* Create a ShortcutsGroup proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public ShortcutsGroup(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Get the GType of the ShortcutsGroup class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gtk_shortcuts_group_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected ShortcutsGroup asParent() {
ShortcutsGroup _parent = new ShortcutsGroup(handle());
_parent.callParent(true);
return _parent;
}
/**
* Adds a shortcut to the shortcuts group.
*
* This is the programmatic equivalent to using {@link GtkBuilder} and a
* {@code } tag to add the child. Adding children with other API is not
* appropriate as {@code GtkShortcutsGroup} manages its children internally.
*
* @param shortcut the {@code GtkShortcutsShortcut} to add
*/
public void addShortcut(ShortcutsShortcut shortcut) {
try {
MethodHandles.gtk_shortcuts_group_add_shortcut.invokeExact(handle(),
(MemorySegment) (shortcut == null ? MemorySegment.NULL : shortcut.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code ShortcutsGroup}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class ShortcutsGroupClass extends TypeClass {
/**
* Create a ShortcutsGroupClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public ShortcutsGroupClass(MemorySegment address) {
super(address);
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends Box.Builder implements Accessible.Builder, Orientable.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code ShortcutsGroup} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code ShortcutsGroup}.
*
* @return a new instance of {@code ShortcutsGroup} with the properties
* that were set in the Builder object.
*/
public ShortcutsGroup build() {
try {
var _instance = (ShortcutsGroup) GObject.withProperties(ShortcutsGroup.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The size group for the accelerator portion of shortcuts in this group.
*
* This is used internally by GTK, and must not be modified by applications.
*
* @param accelSizeGroup the value for the {@code accel-size-group} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAccelSizeGroup(SizeGroup accelSizeGroup) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(SizeGroup.getType());
_value.setObject((GObject) accelSizeGroup);
addBuilderProperty("accel-size-group", _value);
return (B) this;
}
/**
* The title for this group of shortcuts.
*
* @param title the value for the {@code title} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setTitle(String title) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(title);
addBuilderProperty("title", _value);
return (B) this;
}
/**
* The size group for the textual portion of shortcuts in this group.
*
* This is used internally by GTK, and must not be modified by applications.
*
* @param titleSizeGroup the value for the {@code title-size-group} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setTitleSizeGroup(SizeGroup titleSizeGroup) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(SizeGroup.getType());
_value.setObject((GObject) titleSizeGroup);
addBuilderProperty("title-size-group", _value);
return (B) this;
}
/**
* An optional view that the shortcuts in this group are relevant for.
*
* The group will be hidden if the {@code Gtk.ShortcutsWindow:view-name}
* property does not match the view of this group.
*
* Set this to {@code null} to make the group always visible.
*
* @param view the value for the {@code view} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setView(String view) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(view);
addBuilderProperty("view", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle gtk_shortcuts_group_add_shortcut = Interop.downcallHandle(
"gtk_shortcuts_group_add_shortcut", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}