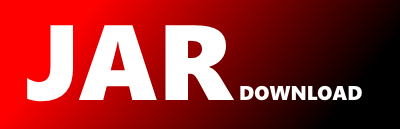
org.freedesktop.harfbuzz.GlyphInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of harfbuzz Show documentation
Show all versions of harfbuzz Show documentation
Java language bindings for Harfbuzz, generated with Java-GI
// Java-GI - Java language bindings for GObject-Introspection-based libraries
// Copyright (C) 2022-2024 Jan-Willem Harmannij
//
// SPDX-License-Identifier: LGPL-2.1-or-later
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this library; if not, see .
//
// This file has been generated with Java-GI.
// Do not edit this file directly!
// Visit for more information.
//
package org.freedesktop.harfbuzz;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.Deprecated;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
/**
* The {@code hb_glyph_info_t} is the structure that holds information about the
* glyphs and their relation to input text.
*/
@Generated("io.github.jwharm.JavaGI")
public class GlyphInfo extends ProxyInstance {
static {
HarfBuzz.javagi$ensureInitialized();
}
/**
* Create a GlyphInfo proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public GlyphInfo(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new GlyphInfo.
*
* @param arena to control the memory allocation scope
*/
public GlyphInfo(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new GlyphInfo.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public GlyphInfo() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* Allocate a new GlyphInfo with the fields set to the provided values.
*
* @param codepoint value for the field {@code codepoint}
* @param mask value for the field {@code mask}
* @param cluster value for the field {@code cluster}
* @param var1 value for the field {@code var1}
* @param var2 value for the field {@code var2}
* @param arena to control the memory allocation scope
*/
public GlyphInfo(Codepoint codepoint, Mask mask, int cluster, VarInt var1, VarInt var2,
Arena arena) {
this(arena);
writeCodepoint(codepoint);
writeMask(mask);
writeCluster(cluster);
writeVar1(var1);
writeVar2(var2);
}
/**
* Allocate a new GlyphInfo with the fields set to the provided values.
* The memory is allocated with {@link Arena#ofAuto}.
*
* @param codepoint value for the field {@code codepoint}
* @param mask value for the field {@code mask}
* @param cluster value for the field {@code cluster}
* @param var1 value for the field {@code var1}
* @param var2 value for the field {@code var2}
*/
public GlyphInfo(Codepoint codepoint, Mask mask, int cluster, VarInt var1, VarInt var2) {
this(Arena.ofAuto());
writeCodepoint(codepoint);
writeMask(mask);
writeCluster(cluster);
writeVar1(var1);
writeVar2(var2);
}
/**
* Get the GType of the GlyphInfo class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("hb_gobject_glyph_info_get_type");
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
ValueLayout.JAVA_INT.withName("codepoint"),
ValueLayout.JAVA_INT.withName("mask"),
ValueLayout.JAVA_INT.withName("cluster"),
MemoryLayout.paddingLayout(4),
VarInt.getMemoryLayout().withName("var1"),
VarInt.getMemoryLayout().withName("var2")
).withName("hb_glyph_info_t");
}
/**
* Allocate a new GlyphInfo.
*
* @param arena to control the memory allocation scope
* @return a new, uninitialized {@link GlyphInfo}
* @deprecated Replaced by {@link GlyphInfo#GlyphInfo(Arena)}
*/
@Deprecated
public static GlyphInfo allocate(Arena arena) {
MemorySegment segment = arena.allocate(getMemoryLayout());
return new GlyphInfo(segment);
}
/**
* Allocate a new GlyphInfo with the fields set to the provided values.
*
* @param arena to control the memory allocation scope
* @param codepoint value for the field {@code codepoint}
* @param mask value for the field {@code mask}
* @param cluster value for the field {@code cluster}
* @param var1 value for the field {@code var1}
* @param var2 value for the field {@code var2}
* @return a new {@link GlyphInfo} with the fields set to the provided values
* @deprecated Replaced by {@link GlyphInfo#GlyphInfo(org.freedesktop.harfbuzz.Codepoint, org.freedesktop.harfbuzz.Mask, int, org.freedesktop.harfbuzz.VarInt, org.freedesktop.harfbuzz.VarInt, Arena)}
*/
@Deprecated
public static GlyphInfo allocate(Arena arena, Codepoint codepoint, Mask mask, int cluster,
VarInt var1, VarInt var2) {
return new GlyphInfo(codepoint, mask, cluster, var1, var2, arena);
}
/**
* Read the value of the field {@code codepoint}.
*
* @return The value of the field {@code codepoint}
*/
public Codepoint readCodepoint() {
var _result = (int) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("codepoint")).get(handle(), 0);
return new Codepoint(_result);
}
/**
* Write a value in the field {@code codepoint}.
*
* @param codepoint The new value for the field {@code codepoint}
*/
public void writeCodepoint(Codepoint codepoint) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("codepoint"))
.set(handle(), 0, codepoint.getValue().intValue());
}
/**
* Read the value of the field {@code mask}.
*
* @return The value of the field {@code mask}
*/
public Mask readMask() {
var _result = (int) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("mask")).get(handle(), 0);
return new Mask(_result);
}
/**
* Write a value in the field {@code mask}.
*
* @param mask The new value for the field {@code mask}
*/
public void writeMask(Mask mask) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("mask"))
.set(handle(), 0, mask.getValue().intValue());
}
/**
* Read the value of the field {@code cluster}.
*
* @return The value of the field {@code cluster}
*/
public int readCluster() {
var _result = (int) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("cluster")).get(handle(), 0);
return _result;
}
/**
* Write a value in the field {@code cluster}.
*
* @param cluster The new value for the field {@code cluster}
*/
public void writeCluster(int cluster) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("cluster"))
.set(handle(), 0, cluster);
}
/**
* Read the value of the field {@code var1}.
*
* @return The value of the field {@code var1}
*/
public VarInt readVar1() {
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("var1")).get(handle(), 0);
return MemorySegment.NULL.equals(_result) ? null : new VarInt(_result);
}
/**
* Write a value in the field {@code var1}.
*
* @param var1 The new value for the field {@code var1}
*/
public void writeVar1(VarInt var1) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("var1"))
.set(handle(), 0, (var1 == null ? MemorySegment.NULL : var1.handle()));
}
/**
* Read the value of the field {@code var2}.
*
* @return The value of the field {@code var2}
*/
public VarInt readVar2() {
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("var2")).get(handle(), 0);
return MemorySegment.NULL.equals(_result) ? null : new VarInt(_result);
}
/**
* Write a value in the field {@code var2}.
*
* @param var2 The new value for the field {@code var2}
*/
public void writeVar2(VarInt var2) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("var2"))
.set(handle(), 0, (var2 == null ? MemorySegment.NULL : var2.handle()));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy