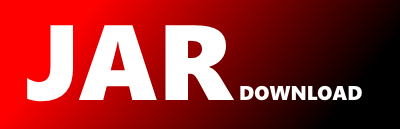
net.kender.core.mc.EXTRAS.EXTRAS Maven / Gradle / Ivy
package net.kender.core.mc.EXTRAS;
import java.io.*;
import java.net.MalformedURLException;
import java.net.URI;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import net.k3nder.CompressUtils;
import net.kender.kutils.OperativeSystem;
import org.apache.commons.compress.archivers.tar.TarArchiveEntry;
import org.apache.commons.compress.archivers.tar.TarArchiveInputStream;
import org.apache.commons.compress.compressors.gzip.GzipCompressorInputStream;
import org.apache.commons.io.FileUtils;
import net.kender.core.JsonUtils;
import net.kender.core.UtilsFiles;
import net.kender.core.mc.CommandConstructor;
import net.kender.core.mc.Vmc;
public class EXTRAS {
public EXTRAS(){
throw new IllegalArgumentException("utils class no instanciable");
}
public static void createJsonVersion(Path __DEST__, Vmc __VERSION__) {
UtilsFiles.createFile(__DEST__.toString() + "\\versions\\" + __VERSION__ + "\\", "json", __VERSION__.toString(),
JsonUtils.getJsonVersion(__VERSION__.getUrl().toString()));
}
public static void natives(Path libs, Path jdk) {
// Utiliza el objeto Files para obtener un Stream de Paths
try (DirectoryStream stream = Files.newDirectoryStream(libs)) {
// Itera sobre los Paths e imprime sus nombres
for (Path archivo : stream) {
if (archivo.toString().contains("natives")) {
CompressUtils.extract(archivo.toFile(), Paths.get(jdk + "\\").toFile());
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
static String quitarUltimaParteRuta(String ruta) {
if (ruta == null || ruta.isEmpty()) {
return ruta;
}
int ultimoSeparador = ruta.lastIndexOf(File.separator);
if (ultimoSeparador != -1) {
return ruta.substring(0, ultimoSeparador);
} else {
return ruta;
}
}
public static void createFile(File d, String fileContent) {
// Crea el archivo con el contenido especificado
try {
FileUtils.writeStringToFile(d, fileContent, "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
// log( SEPARATOR);
// log( "the arch is creted: " + d.getAbsolutePath());
// log( SEPARATOR);
}
public static void download(URI url, File h) throws IOException {
FileUtils.copyURLToFile(url.toURL(), h);
}
private static PrintStream stream = System.out;
private static Process process;
private static final OperativeSystem SYSTEM = OperativeSystem.thisOperativeSystem();
public static void run(CommandConstructor command) {
try {
System.out.println(command.toString());
String[] commands = (SYSTEM == OperativeSystem.Windows ? new String[]{"cmd.exe", "/c", command.command} : new String[]{"bin/sh", "-c", command.command});
ProcessBuilder processBuilder = new ProcessBuilder(commands);
processBuilder.redirectErrorStream(true); // Redirige la salida de ERROR al mismo flujo de entrada
process = processBuilder.start();
InputStream inputStream = process.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String linea;
while ((linea = reader.readLine()) != null) {
stream.println(linea);
}
} catch (IOException e) {
e.printStackTrace();
Thread.currentThread().interrupt();
}
}
public static void close(){
process.destroy();
}
public static PrintStream getCommandOutputStrem(){
return stream;
}
public static void setPrintStream(PrintStream str){
stream = str;
}
public static String read(File e) {
try {
BufferedReader lector = new BufferedReader(new FileReader(e));
String linea;
while ((linea = lector.readLine()) != null) {
System.out.println(linea); // Imprime cada línea del archivo
}
lector.close();
return linea;
} catch (IOException e1) {
e1.printStackTrace();
return "";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy