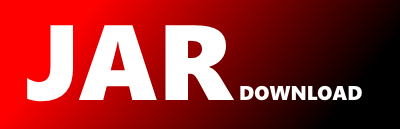
net.kender.core.mc.Mc Maven / Gradle / Ivy
package net.kender.core.mc;
import static net.kender.core.mc.EXTRAS.EXTRAS.*;
import static net.kender.core.mc.EXTRAS.LIBS.*;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.UUID;
import net.k3nder.CompressUtils;
import net.kender.core.Json.Version.javaV;
import net.kender.core.JsonUtils;
import net.kender.core.assetsDowloader;
import net.kender.core.Json.Version.versionJson;
import net.kender.core.mc.EXTRAS.EXTRAS;
import net.kender.core.mc.Quikplays.Quikplay;
import net.kender.core.mc.Quikplays.profile;
import net.kender.kutils.OperativeSystem;
public class Mc {
private McEventStep onStep = (float step,String stepname) -> {
System.out.println("[OK] task " + stepname + " number " + step);
};
private McEventException onErr = (Exception e) -> {
System.out.println("[ERR] task failed: " + e.getMessage());
};
private Runnable onDone = () -> {
System.out.println("DONE");
};
private static final OperativeSystem system = OperativeSystem.thisOperativeSystem();
public static final String USER_NAME = System.getProperty("user.name");
public static final String DISC = (system == OperativeSystem.Linux ? "/home/" : "C:/Users/");
public static String MINECRAFT_PATH = DISC + USER_NAME
+ (system == OperativeSystem.Linux ? "/.m" : "/AppData/Roaming/.minecraft");
private Path destination;
private Vmc version;
private Path libreries;
private String username = "testName";
private Path gamedir = Paths.get(MINECRAFT_PATH);
private Path jrepath = Paths
.get("C:/Users/krist/Documents/.LT/LT-2.0/LT/MC/versions/1.20.2/jre/bin/java");
private UUID uuid = UUID.fromString("d0db8a3d-c392-4ae7-96e5-9365de33ab52");
private String xuid = "0";
private Path assetsdir = Paths.get(MINECRAFT_PATH + "/assets/");
private String accestoken = "0";
private String clientid = "";
private String usertype = "";
public boolean __STOP__ = false;
private int maxRam = 4;
private int minRam = 2;
private Path nativespath = Paths.get("debug/path");
private static final String JRE8 = (system == OperativeSystem.Linux ? "https://github.com/adoptium/temurin8-binaries/releases/download/jdk8u392-b08/OpenJDK8U-jdk_x64_linux_hotspot_8u392b08.tar.gz" : "https://github.com/adoptium/temurin8-binaries/releases/download/jdk8u382-b05/OpenJDK8U-jre_x64_windows_hotspot_8u382b05.zip");
private static final String JRE20 = (system == OperativeSystem.Linux ? "https://github.com/adoptium/temurin20-binaries/releases/download/jdk-20.0.2%2B9/OpenJDK20U-jdk_x64_linux_hotspot_20.0.2_9.tar.gz" : "https://github.com/adoptium/temurin20-binaries/releases/download/jdk-20.0.1%2B9/OpenJDK20U-jre_x64_windows_hotspot_20.0.1_9.zip");
public Mc(Path destination, Vmc version) {
this.destination = destination;
this.version = version;
nativespath = Paths.get(destination + "\\versions\\" + version.getID() + "\\bin\\");
try {
download(new URI(
JRE8),
new File(destination + "/JRE/8/jre.zip"));
} catch (URISyntaxException | IOException e) {
// TODO Auto-generated catch block
onErr.run(e);
}
CompressUtils.extract(new File(destination + "/JRE/8/jre.zip"), Paths.get(destination + "/JRE/8/jre/").toFile());
try {
download(new URI(
JRE20),
new File(destination + "/JRE/20/jre.zip"));
} catch (URISyntaxException | IOException e) {
// TODO Auto-generated catch block
onErr.run(e);
}
CompressUtils.extract(new File(destination + "/JRE/20/jre.zip"),
Paths.get(destination + "/JRE/20/jre/").toFile());
}
public Mc(profile profile) throws IOException, URISyntaxException {
this.destination = profile.getGameDir();
if (!destination.toFile().exists()) {
destination.toFile().createNewFile();
}
this.version = profile.getVersion();
nativespath = Paths.get(destination + "\\versions\\" + version.getID() + "\\bin\\");
jrepath = profile.getJre();
try {
download(new URI(
JRE8),
new File(destination + "/JRE/8/jre.zip"));
} catch (URISyntaxException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
CompressUtils.extract(new File(destination + "/JRE/8/jre.zip"),
Paths.get(destination + "/JRE/8/jre/").toFile());
try {
download(new URI(
JRE20),
new File(destination + "/JRE/20/jre.zip"));
} catch (URISyntaxException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
CompressUtils.extract(new File(destination + "/JRE/20/jre.zip"),
Paths.get(destination + "/JRE/20/jre/").toFile());
}
private void createJsVe() {
libreries = Paths.get(destination + "\\versions\\" + version + "\\libraries\\");
if (!new File(destination.toString() + "\\versions\\" + version + "\\" + version + ".json").exists()) {
// down.set(2, "preparing");
createJsonVersion(destination, version);
}
}
private void downloadAndPreparating(versionJson vanilla, File JarV) throws URISyntaxException, IOException {
download(new URI(vanilla.downloads.client.url), JarV);
getlibs(libreries, vanilla.libraries);
natives(libreries, Paths.get(destination.toString() + "\\versions\\" + version + "\\bin"));
assets(destination, version.toString(), vanilla.assets);
getJRE(vanilla.javaVersion);
}
private void downloadAndPreparating(versionJson vanilla, versionJson a, File JarV) throws URISyntaxException, IOException {
download(new URI(vanilla.downloads.client.url), JarV);
getlibs(libreries, vanilla.libraries);
getlibs(libreries, a.libraries);
natives(libreries, Paths.get(destination.toString() + "\\versions\\" + version + "\\bin"));
assets(destination, a.inheritsFrom, vanilla.assets);
getJRE(vanilla.javaVersion);
}
public void run() {
Thread r = new Thread(new Runnable() {
public void run() {
try {
onStep.run(20,"init");
File jsonVersion = new File(destination.toString() + "\\versions\\" + version.getID(),
version.getID() + ".json");
File jarVersion = new File(destination.toString() + "\\versions\\" + version.getID(),
version.getID() + ".jar");
createJsVe();
onStep.run(40,"create json version file");
versionJson a = versionJson.load(
new File(destination.toString() + "\\versions\\" + version, version + ".json"));
onStep.run(60,"load json version: custom: " + a.isCustom());
if (a.isCustom()) {
if (jsonVersion.exists()) {
URI vanillaVersionJ = new Manifest().getVersion(a.inheritsFrom).getUrl();
onStep.run(80,"detected custom json version");
versionJson vanilla = versionJson.load(vanillaVersionJ);
onStep.run(90,"get vanilla json version");
downloadAndPreparating(vanilla, a, jarVersion);
onDone.run();
CommandConstructor t = new CommandConstructor(username, maxRam, minRam, vanilla.assets,
libreries.toString() + "\\*", a.mainClass, a.id, gamedir.toString(),
jrepath.toString(), destination, uuid, accestoken, assetsdir, xuid, clientid,
usertype, a.type, nativespath);
EXTRAS.run(t);
}
} else {
if (jsonVersion.exists()) {
onStep.run(80,"json vanilla version");
downloadAndPreparating(a, jarVersion);
onStep.run(90,"download jar");
onDone.run();
CommandConstructor t = new CommandConstructor(username, maxRam, minRam, a.assets,
libreries.toString() + "\\*", a.mainClass, a.id, gamedir.toString(),
jrepath.toString(), destination, uuid, accestoken, assetsdir, xuid, clientid,
usertype, a.type, nativespath);
EXTRAS.run(t);
}
}
} catch (IOException | URISyntaxException e) {
onErr.run(e);
}
}
});
r.start();
}
public void run(Quikplay torun) {
Thread r = new Thread(new Runnable() {
public void run() {
try {
onStep.run(20,"init");
File JsonV = new File(
destination.toString() + "\\versions\\" + version.getID(), version.getID() + ".json");
File JarV = new File(
destination.toString() + "\\versions\\" + version.getID(), version.getID() + ".jar");
createJsVe();
onStep.run(40,"create json version file");
versionJson a = versionJson.load(
new File(destination.toString() + "\\versions\\" + version + "\\" + version + ".json"));
onStep.run(60,"load json version");
if (a.isCustom()) {
if (JsonV.exists()) {
URI vanillaVersionJ = new Manifest().getVersion(a.inheritsFrom).getUrl();
onStep.run(80,"detected custom json version");
versionJson vanilla = versionJson.load(vanillaVersionJ);
onStep.run(90,"get vanilla json version");
downloadAndPreparating(vanilla, a, JarV);
onDone.run();
CommandConstructor t = new CommandConstructor(username, maxRam, minRam, vanilla.assets,
libreries.toString() + "\\*", a.mainClass, a.id, gamedir.toString(), jrepath.toString(),
destination, uuid, accestoken, assetsdir, xuid, clientid, usertype, a.type, torun,
nativespath);
EXTRAS.run(t);
}
} else {
if (JsonV.exists()) {
onStep.run(80,"json vanilla version");
downloadAndPreparating(a, JarV);
onStep.run(90,"download jar");
onDone.run();
CommandConstructor t = new CommandConstructor(username, maxRam, minRam, a.assets,
libreries.toString() + "\\*", a.mainClass, a.id, gamedir.toString(), jrepath.toString(),
destination, uuid, accestoken, assetsdir, xuid, clientid, usertype, a.type, torun,
nativespath);
EXTRAS.run(t);
}
}
} catch(IOException | URISyntaxException e) {
onErr.run(e);
}
}
});
r.start();
}
public void setRam(int maxRam, int minRam) {
this.maxRam = maxRam;
this.minRam = minRam;
}
public void setDest(Path e) {
destination = e;
}
public void setUser(String user) {
username = user;
}
public void setGameDir(Path GameDir) {
gamedir = GameDir;
}
public void setJavaJRE(Path bin) {
jrepath = bin;
}
public void setUUID(UUID e) {
uuid = e;
}
public void setXUID(String XUID) {
this.xuid = XUID;
}
public void setAssetsDir(Path ASSETSDIR) {
assetsdir = ASSETSDIR;
}
public void setAccesToken(String AccesToken) {
accestoken = AccesToken;
}
public void setClientId(String ClientId) {
clientid = ClientId;
}
public void setUserType(String UserType) {
usertype = UserType;
}
public void setNativesPath(Path a) {
nativespath = a;
}
public void setOnStep(McEventStep s) {
onStep = s;
}
public void setOnErr(McEventException err){
onErr = err;
}
public void onFinaly(Runnable s) {
onDone = s;
}
private void getJRE(javaV javanode) {
if(javanode == null) {
jrepath = Paths.get(destination + "\\JRE\\8\\jre\\jdk8u382-b05-jre");
return;
}
int javaVersion = javanode.majorVersion;
if (javaVersion <= 8) {
jrepath = Paths.get(destination + "\\JRE\\8\\jre\\jdk8u382-b05-jre");
// int JavaV = 8;
// UtilsFiles.getnatives(destination + "\\versions\\" + __VERSION__ +
// "\\libraries\\", DirectoyOfBin + "\\" + JreUUID8.toString(), ".zip");
} else {
jrepath = Paths.get(destination + "\\JRE\\20\\jre\\jdk-20.0.1+9-jre");
// int JavaV = 20;
}
}
private void assets(Path dest, String version, String index) throws URISyntaxException {
String url = JsonUtils.readAssetsIndexUrlFromJson(dest + "\\versions\\" + version + "\\" + version + ".json");
String cont = JsonUtils.getJsonVersion(url);
EXTRAS.createFile(new File(dest + "\\assets\\indexes\\" + index + ".json"), cont);
try {
assetsDowloader.AssetsDownload(dest + "\\assets\\indexes\\" + index + ".json",
dest + "\\assets\\virtual\\legacy\\", dest + "\\assets\\objects\\");
} catch (IOException e) {
onErr.run(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy