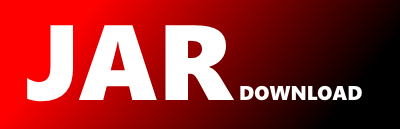
net.k3nder.core.mc.Manifest Maven / Gradle / Ivy
The newest version!
package net.k3nder.core.mc;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import net.kender.Kjson.Json;
public class Manifest {
public static final String MANIFEST_URL_DEFAULT = "https://launchermeta.mojang.com/mc/game/version_manifest.json";
private JsonNode last;
private Map list = new HashMap<>();
public Manifest(URL manifest) throws JsonProcessingException{
// itarar sobre cada objeto en el json en el objeto versions
for(JsonNode l:Json.jContentOf(manifest.toString()).get("versions")){
try {
// añadir al mapa de versiones
list.put(l.get("id").asText(),new Vmc(l.get("id").asText(), VersionType.valueOf(l.get("type").asText()),new URI(l.get("url").asText()),l.get("id").asText()));
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
last = Json.jContentOf(manifest.toString()).get("latest");
}
public Manifest() throws JsonProcessingException {
try {
// itarar sobre cada objeto en el json en el objeto versions
for(JsonNode l:Json.jContentOf(MANIFEST_URL_DEFAULT).get("versions")){
// añadir al mapa de versiones
list.put(l.get("id").asText(),new Vmc(l.get("id").asText(), VersionType.valueOf(l.get("type").asText()),new URI(l.get("url").asText()),l.get("id").asText()));
}
last = Json.jContentOf(new URI(MANIFEST_URL_DEFAULT)).get("latest");
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
public boolean exist(String version){
if(version == null) {
throw new IllegalArgumentException("invalid argument null ");
}
return list.containsKey(version);
}
public Vmc getVersion(String of){
if(of.equals("latest-release")){
return this.getLastVersion();
}
if(of.equals("latest-snapshot")){
return this.getLastSnapshot();
}
if(!list.containsKey(of)){
throw new IllegalArgumentException("version not exist in the manifest: " + of);
}
return list.get(of);
}
public List getList(){
ArrayList li = new ArrayList<>();
for(Entry l:list.entrySet()){
li.add(l.getValue());
}
return li;
}
public Vmc getLastVersion(){
String x = last.get("release").asText();
return list.get(x);
}
public Vmc getLastSnapshot(){
String x = last.get("snapshot").asText();
return list.get(x);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy