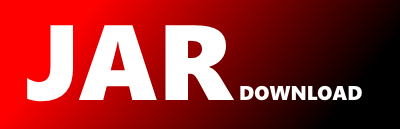
net.k3nder.core.mc.Mc Maven / Gradle / Ivy
The newest version!
package net.k3nder.core.mc;
import static net.k3nder.core.mc.utils.Utils.*;
import static net.k3nder.core.mc.utils.LibsUtils.getlibs;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.UUID;
import com.fasterxml.jackson.core.JsonProcessingException;
import net.k3nder.CompressUtils;
import net.k3nder.core.json.version.javaV;
import net.k3nder.core.JsonUtils;
import net.k3nder.core.AssetsUtils;
import net.k3nder.core.json.version.versionJson;
import net.k3nder.core.mc.utils.Utils;
import net.k3nder.core.mc.quickplays.Quikplay;
import net.kender.kutils.OperativeSystem;
import org.apache.commons.io.FileUtils;
public class Mc {
private McEventStep onStep = (float step, String stepname) -> {
System.out.println("[OK] task " + stepname + " number " + step);
};
private McEventException onErr = (Exception e) -> {
System.out.println("[ERR] task failed: " + e.getMessage());
};
private Runnable onDone = () -> {
System.out.println("DONE");
};
private static final OperativeSystem SYSTEM = OperativeSystem.thisOperativeSystem();
public static final String USER_NAME = System.getProperty("user.name");
public static final String DISC = (SYSTEM == OperativeSystem.Linux ? "/home/" : "C:/Users/");
public static String MINECRAFT_PATH = DISC + USER_NAME
+ (SYSTEM == OperativeSystem.Linux ? "/.m" : "/AppData/Roaming/.minecraft");
private Path destination;
private Path libraries;
private String username = "testName";
private Path gamedir = Paths.get(MINECRAFT_PATH);
private Path jrepath = Paths
.get("C:/Users/krist/Documents/.LT/LT-2.0/LT/MC/versions/1.20.2/jre/bin/java");
private UUID uuid = UUID.fromString("d0db8a3d-c392-4ae7-96e5-9365de33ab52");
private String xuid = "0";
private Path assetsdir = Paths.get(MINECRAFT_PATH + "/assets/");
private String accestoken = "0";
private String clientid = "";
private String usertype = "";
private int maxRam = 4;
private int minRam = 2;
private Path nativespath;
private static final String JRE8 = (SYSTEM == OperativeSystem.Linux ? "https://github.com/adoptium/temurin8-binaries/releases/download/jdk8u392-b08/OpenJDK8U-jdk_x64_linux_hotspot_8u392b08.tar.gz" : "https://github.com/adoptium/temurin8-binaries/releases/download/jdk8u382-b05/OpenJDK8U-jre_x64_windows_hotspot_8u382b05.zip");
private static final String JRE20 = (SYSTEM == OperativeSystem.Linux ? "https://github.com/adoptium/temurin21-binaries/releases/download/jdk-21.0.3%2B9/OpenJDK21U-jre_x64_linux_hotspot_21.0.3_9.tar.gz" : "https://github.com/adoptium/temurin21-binaries/releases/download/jdk-21.0.3%2B9/OpenJDK21U-jre_x64_windows_hotspot_21.0.3_9.zip");
public Mc(Path destination) {
this.destination = destination;
downloadJres(destination);
}
private void downloadJres(Path destination) {
try {
FileUtils.copyURLToFile(new URI(
JRE8).toURL(),
new File(destination + "/JRE/8/jre.zip"));
FileUtils.copyURLToFile(new URI(
JRE20).toURL(),
new File(destination + "/JRE/20/jre.zip"));
CompressUtils.extract(new File(destination + "/JRE/8/jre.zip"), Paths.get(destination + "/JRE/8/jre/").toFile());
CompressUtils.extract(new File(destination + "/JRE/20/jre.zip"),
Paths.get(destination + "/JRE/20/jre/").toFile());
} catch (URISyntaxException | IOException e) {
// TODO Auto-generated catch block
onErr.run(e);
}
}
private void downloadAndPrepare(versionJson vanilla,versionJson version, File JarV) throws URISyntaxException, IOException {
FileUtils.copyURLToFile(new URI(vanilla.downloads.client.url).toURL(), JarV);
getlibs(libraries, vanilla.libraries);
natives(libraries, Paths.get(destination.toString() + "\\versions\\" + version + "\\bin"));
assets(destination, version);
getJRE(vanilla.javaVersion);
}
private void downloadAndPrepare(versionJson vanilla, versionJson a,versionJson version, File JarV) throws URISyntaxException, IOException {
FileUtils.copyURLToFile(new URI(vanilla.downloads.client.url).toURL(), JarV);
getlibs(libraries, vanilla.libraries);
getlibs(libraries, a.libraries);
natives(libraries, Paths.get(destination.toString() + "\\versions\\" + version + "\\bin"));
assets(destination, vanilla);
getJRE(vanilla.javaVersion);
}
public void download(versionJson version) {
libraries = Paths.get(destination + "\\versions\\" + version.id + "\\libraries\\");
try {
onStep.run(20, "init");
File jarVersion = new File(destination + "\\versions\\" + version.id,
version.id + ".jar");
onStep.run(60, "load json version: custom: " + version.isCustom());
if (version.isCustom()) {
URI vanillaVersionJ = new Manifest().getVersion(version.inheritsFrom).getUrl();
onStep.run(80, "detected custom json version");
versionJson vanilla = versionJson.load(vanillaVersionJ);
onStep.run(90, "get vanilla json version");
downloadAndPrepare(vanilla, version, version, jarVersion);
} else {
onStep.run(80, "json vanilla version");
downloadAndPrepare(version, version, jarVersion);
onStep.run(90, "download jar");
}
} catch (IOException | URISyntaxException e) {
throw new RuntimeException(e);
}
}
public void run(versionJson a) throws IOException {
versionJson vanilla;
libraries = Paths.get(destination + "\\versions\\" + a.id + "\\libraries\\");
nativespath = Paths.get(destination + "\\versions\\" + a.id + "\\bin\\");
getJRE(a.javaVersion);
if(a.inheritsFrom != null){
URI vanillaVersionJ = new Manifest().getVersion(a.inheritsFrom).getUrl();
onStep.run(80, "detected custom json version");
vanilla = versionJson.load(vanillaVersionJ);
getJRE(vanilla.javaVersion);
} else vanilla = a;
onDone.run();
CommandConstructor t = new CommandConstructor(new CommandConstructor.Builder(username, maxRam, minRam, vanilla.assets,
libraries.toString() + "\\*", a.mainClass, a.id, gamedir.toString(),
jrepath.toString(), destination, uuid, accestoken, assetsdir, xuid, clientid,
usertype, a.type, nativespath));
Utils.run(t);
}
public void run() {
}
public void setRam(int maxRam, int minRam) {
this.maxRam = maxRam;
this.minRam = minRam;
}
public void setDest(Path e) {
destination = e;
}
public void setUser(String user) {
username = user;
}
public void setGameDir(Path GameDir) {
gamedir = GameDir;
}
public void setJavaJRE(Path bin) {
jrepath = bin;
}
public void setUUID(UUID e) {
uuid = e;
}
public void setXUID(String XUID) {
this.xuid = XUID;
}
public void setAssetsDir(Path ASSETSDIR) {
assetsdir = ASSETSDIR;
}
public void setAccesToken(String AccesToken) {
accestoken = AccesToken;
}
public void setClientId(String ClientId) {
clientid = ClientId;
}
public void setUserType(String UserType) {
usertype = UserType;
}
public void setNativesPath(Path a) {
nativespath = a;
}
public void setOnStep(McEventStep s) {
onStep = s;
}
public void setOnErr(McEventException err){
onErr = err;
}
public void onFinal(Runnable s) {
onDone = s;
}
private void getJRE(javaV javanode) {
if(javanode == null) {
jrepath = Paths.get(destination + "\\JRE\\8\\jre\\jdk8u382-b05-jre");
return;
}
int javaVersion = javanode.majorVersion;
if (javaVersion <= 8) {
jrepath = Paths.get(destination + "\\JRE\\8\\jre\\jdk8u382-b05-jre");
} else {
jrepath = Paths.get(destination + "\\JRE\\20\\jre\\jdk-21.0.3+9-jre");
}
}
private void assets(Path dest, versionJson version) throws URISyntaxException {
String cont = JsonUtils.getJsonVersion(version.assetIndex.url);
String index = version.assets;
Utils.createFile(new File(dest + "\\assets\\indexes\\" + index + ".json"), cont);
try {
AssetsUtils.AssetsDownload(dest + "\\assets\\indexes\\" + index + ".json",
dest + "\\assets\\virtual\\legacy\\", dest + "\\assets\\objects\\");
} catch (IOException e) {
onErr.run(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy