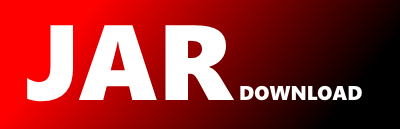
io.github.kamilszewc.tabulator.ObjectProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tabulator Show documentation
Show all versions of tabulator Show documentation
Simple tables/lists output formater
The newest version!
package io.github.kamilszewc.tabulator;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
import java.util.stream.Collectors;
class ObjectProcessor {
public static List getAllGetMethods(Class> aClass) {
return Arrays.stream(aClass.getDeclaredMethods())
.filter(c -> c.getName().startsWith("get"))
.sorted(Comparator.comparing(Method::getName))
.collect(Collectors.toList());
}
public static List getListOfKeys(Object object) {
return getAllGetMethods(object.getClass()).stream()
.map(ObjectProcessor::extractKeyFormMethod)
.collect(Collectors.toList());
}
public static List getListOfValues(Object object) {
return getAllGetMethods(object.getClass()).stream()
.map(method -> {
try {
Object result = method.invoke(object);
if (result != null) {
return method.invoke(object).toString();
} else {
return "";
}
} catch (Exception ex) {
return "";
}
})
.collect(Collectors.toList());
}
public static String extractKeyFormMethod(Method method) {
String name = method.getName().replaceFirst("get", "");
StringBuilder sb = new StringBuilder(name);
sb.setCharAt(0, Character.toLowerCase(sb.charAt(0)));
return sb.toString();
}
public static Map getMapOfMethodNameAndValue(Object object, List selectedKeys) {
if (selectedKeys == null) return getMapOfMethodNameAndValue(object);
Map map = new LinkedHashMap<>();
List methods = getAllGetMethods(object.getClass());
selectedKeys.stream().forEach(key -> {
Method method;
try {
method = methods.stream()
.filter(m -> {
String name = extractKeyFormMethod(m);
return name.equals(key);
})
.findFirst()
.get();
} catch (NoSuchElementException ex) {
return;
}
try {
String name = extractKeyFormMethod(method);
Object result = method.invoke(object);
if (result != null) {
map.put(name, method.invoke(object).toString());
} else {
map.put(name, "");
}
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
}
});
return map;
}
public static Map getMapOfMethodNameAndValue(Object object) {
Map map = new LinkedHashMap<>();
List methods = getAllGetMethods(object.getClass());
methods.stream().forEach(method -> {
try {
String name = extractKeyFormMethod(method);
Object result = method.invoke(object);
if (result != null) {
map.put(name, method.invoke(object).toString());
} else {
map.put(name, "");
}
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
}
});
return map;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy