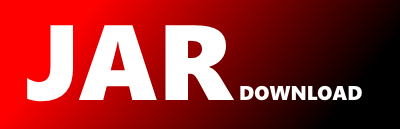
io.github.karlatemp.mxlib.selenium.MxSelenium Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2018-2021 Karlatemp. All rights reserved.
* @author Karlatemp
*
* MXLib/MXLib.mxlib-selenium.main/MxSelenium.java
*
* Use of this source code is governed by the MIT license that can be found via the following link.
*
* https://github.com/Karlatemp/MxLib/blob/master/LICENSE
*/
package io.github.karlatemp.mxlib.selenium;
import com.google.common.base.Splitter;
import io.github.karlatemp.mxlib.MxLib;
import io.github.karlatemp.mxlib.common.utils.IOUtils;
import io.github.karlatemp.mxlib.logger.MLogger;
import io.github.karlatemp.mxlib.utils.Toolkit;
import org.apache.http.client.HttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.io.*;
import java.nio.charset.Charset;
import java.time.Duration;
import java.util.*;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.zip.ZipFile;
import static org.openqa.selenium.support.ui.ExpectedConditions.presenceOfElementLocated;
@SuppressWarnings("ResultOfMethodCallIgnored")
public class MxSelenium {
static final Charset STD_CHARSET;
static final File JAVA_EXECUTABLE;
static final File data = new File(MxLib.getDataStorage(), "selenium");
static final HttpClient client = HttpClientBuilder.create().build();
static boolean IS_SUPPORT, DEBUG = System.getProperty("mxlib.selenium.debug") != null;
public static boolean isSupported() {
return IS_SUPPORT;
}
static MLogger getLogger() {
return MxLib.getLoggerOrStd("MxLib Selenium");
}
static {
{
File je;
String sbt = System.getProperty("sun.boot.library.path");
File exec_windows = new File(sbt, "java.exe");
File exec = new File(sbt, "java");
if (exec_windows.isFile()) {
je = exec_windows;
} else if (exec.exists() && exec.canExecute()) {
je = exec;
} else {
String jhome = System.getProperty("java.home");
exec_windows = new File(jhome, "bin/java.exe");
exec = new File(jhome, "bin/java");
if (exec_windows.isFile()) {
je = exec_windows;
} else if (exec.exists() && exec.canExecute()) {
je = exec;
} else {
je = null;
}
}
JAVA_EXECUTABLE = je;
}
{
Charset c;
try {
String property = System.getProperty("sun.stdout.encoding");
if (property == null) {
if (JAVA_EXECUTABLE == null) {
throw new UnsupportedOperationException("Java Executable not found.");
}
try {
String result = commandProcessResult(true, JAVA_EXECUTABLE.getPath(), "-XshowSettings:properties", "-version");
Optional fileEncoding = Splitter.on('\n').splitToList(result).stream()
.filter(it -> it.trim().startsWith("file.encoding"))
.findFirst();
String rex = fileEncoding.orElse(null);
if (rex != null) {
property = Splitter.on('=').limit(2)
.splitToList(rex)
.get(1)
.trim();
}
} catch (Throwable ignore) {
}
}
//noinspection ConstantConditions
c = Charset.forName(property);
} catch (Throwable ignored) {
try {
c = Charset.forName(System.getProperty("file.encoding"));
} catch (Throwable ignored0) {
c = Charset.defaultCharset();
}
}
STD_CHARSET = c;
}
data.mkdirs();
}
static String commandProcessResult(String... cmd) throws IOException {
return commandProcessResult(false, cmd);
}
static String commandProcessResult(boolean errorStream, String... cmd) throws IOException {
ProcessBuilder builder = new ProcessBuilder(cmd);
if (errorStream) {
builder.redirectOutput(Toolkit.IO.REDIRECT_DISCARD);
} else {
builder.redirectError(Toolkit.IO.REDIRECT_DISCARD);
}
Process process = builder.start();
return new String(IOUtils.readAllBytes(
errorStream ? process.getErrorStream() : process.getInputStream()
), STD_CHARSET == null ? Charset.defaultCharset() : STD_CHARSET);
}
private static boolean initialized;
private static BiFunction, RemoteWebDriver> driverSupplier;
private static Class extends RemoteWebDriver> driverClass;
static Collection setOf(String... urls) {
ArrayList result = new ArrayList<>(urls.length);
for (String u : urls) {
if (!result.contains(u))
result.add(u);
}
return result;
}
public static void initialize() throws Exception {
if (initialized) return;
synchronized (MxSelenium.class) {
if (initialized) return;
try {
initialize0();
} catch (Throwable throwable) {
driverSupplier = (a, b) -> {
throw new RuntimeException(throwable);
};
IS_SUPPORT = false;
throw throwable;
}
}
}
@SuppressWarnings("StatementWithEmptyBody")
private static void initialize0() throws Exception {
if (initialized) return;
synchronized (MxSelenium.class) {
if (initialized) return;
initialized = true;
String os = System.getProperty("os.name");
if (os.toLowerCase().startsWith("windows ")) {
String browser = WindowsKit.queryBrowserUsing();
if (browser.startsWith("Chrome")) {
// region chrome
File bat = new File(data, "chromever.bat");
if (!bat.isFile()) {
data.mkdirs();
try (OutputStream out = new BufferedOutputStream(new FileOutputStream(bat));
InputStream res = MxSelenium.class.getResourceAsStream("resources/chromever.bat")) {
assert res != null;
Toolkit.IO.writeTo(res, out);
}
}
String result = commandProcessResult("cmd", "/c", bat.getPath());
if (result.trim().isEmpty()) {
StringBuilder builder = new StringBuilder();
for (String[] cmd : ChromeKit.windowsVerCommands) {
builder.append(commandProcessResult(cmd));
builder.append('\n').append('\n').append('\n');
}
result = builder.toString();
}
String chromeverx = System.getProperty("mxlib.selenium.chrome.version");
if (chromeverx == null) {
Map> chromever = WindowsKit.parseRegResult(result);
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy