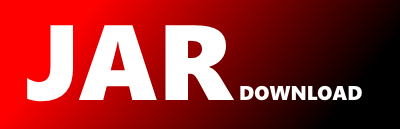
koji.developerkit.runnable.KRunnable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of developerkit Show documentation
Show all versions of developerkit Show documentation
Koji's Developer kit is a dev kit I made for Minecraft that can make creating things and doing things
within the Minecraft Spigot API easier
package koji.developerkit.runnable;
import koji.developerkit.utils.KStatic;
import org.bukkit.Bukkit;
import org.bukkit.plugin.java.JavaPlugin;
import org.bukkit.scheduler.BukkitRunnable;
import org.bukkit.scheduler.BukkitTask;
import org.jetbrains.annotations.NotNull;
import java.util.HashMap;
import java.util.function.Consumer;
public class KRunnable extends BukkitRunnable {
static {
plugin = KStatic.getPlugin();
}
private static final JavaPlugin plugin;
public KRunnable(Consumer task) {
this.task = task;
}
public KRunnable(Consumer task, long period) {
this.task = task;
this.period = period;
cancelAfter(period);
}
long period = -Integer.MAX_VALUE;
Consumer task;
HashMap> tasksOnCancel = new HashMap<>();
private boolean cancelled = false;
public boolean isCancelled() {
return cancelled;
}
@Override
public void run() {
task.accept(this);
}
public KRunnable cancelAfter(long period) {
Bukkit.getScheduler().runTaskLater(plugin, () -> {
if (!cancelled) {
cancel(CancellationActivationType.TIME);
}
}, period);
return this;
}
public void cancel(CancellationActivationType type) {
cancelled = true;
super.cancel();
CancellationActivationType[] typesList = new CancellationActivationType[]{
type, CancellationActivationType.BOTH
};
for (CancellationActivationType types : typesList) {
if (tasksOnCancel.getOrDefault(types, null) != null) {
tasksOnCancel.get(types).accept(this);
}
}
}
@Override
public void cancel() {
cancel(CancellationActivationType.PREMATURE);
}
public KRunnable cancelTask(Consumer taskAfter, CancellationActivationType type) {
tasksOnCancel.put(type, taskAfter);
return this;
}
public enum CancellationActivationType {
PREMATURE,
TIME,
BOTH
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy