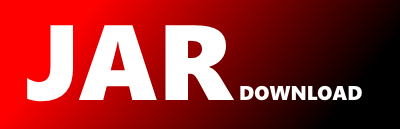
koji.developerkit.utils.duplet.Triplet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of developerkit Show documentation
Show all versions of developerkit Show documentation
Koji's Developer kit is a dev kit I made for Minecraft that can make creating things and doing things
within the Minecraft Spigot API easier
package koji.developerkit.utils.duplet;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Stream;
public class Triplet extends Tuple {
private A first;
private B second;
private C third;
private Triplet(A first, B second, C third) {
this.first = first;
this.second = second;
this.third = third;
}
public static Function, Stream> flat(
Function super A, ? extends D> mapFirst,
Function super B, ? extends D> mapSecond,
Function super C, ? extends D> mapThird) {
return triplet -> triplet.stream(mapFirst, mapSecond, mapThird);
}
public Stream stream(Function super A, ? extends D> mapFirst,
Function super B, ? extends D> mapSecond,
Function super C, ? extends D> mapThird) {
return Stream.of(mapFirst.apply(first), mapSecond.apply(second), mapThird.apply(third));
}
public static Function, Quartet> mapToQuartet(
TriFunction super A, ? super B, ? super C, ? extends D> fun) {
return triplet -> triplet.add(fun.apply(triplet.first, triplet.second, triplet.third));
}
public Quartet add(D fourth) {
return Quartet.of(first, second, third, fourth);
}
public static Triplet of(A first, B second, C third) {
return new Triplet<>(first, second, third);
}
public A getFirst() {
return first;
}
public B getSecond() {
return second;
}
public C getThird() {
return third;
}
public void setFirst(A first) {
this.first = first;
}
public void setSecond(B second) {
this.second = second;
}
public void setThird(C third) {
this.third = third;
}
public boolean contains(T object) {
return getFirst().equals(object) || getSecond().equals(object) || getThird().equals(object);
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("Triplet{");
sb.append("first=").append(first);
sb.append(", second=").append(second);
sb.append(", third=").append(third);
sb.append('}');
return sb.toString();
}
public boolean equals(Triplet other) {
return first.equals(other.getFirst())
&& second.equals(other.getSecond())
&& third.equals(other.getThird());
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Triplet, ?, ?> triplet = (Triplet, ?, ?>) o;
return Objects.equals(first, triplet.first) &&
Objects.equals(second, triplet.second) &&
Objects.equals(third, triplet.third);
}
@Override
public int hashCode() {
return Objects.hash(first, second, third);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy