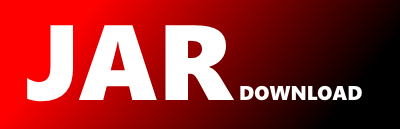
io.github.kosmx.bendylib.mixin.CuboidMutator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bendy-lib Show documentation
Show all versions of bendy-lib Show documentation
Minecraft FabricMC model render library
The newest version!
package io.github.kosmx.bendylib.mixin;
import io.github.kosmx.bendylib.ICuboidBuilder;
import io.github.kosmx.bendylib.MutableCuboid;
import io.github.kosmx.bendylib.impl.accessors.CuboidSideAccessor;
import io.github.kosmx.bendylib.impl.ICuboid;
import org.spongepowered.asm.mixin.Final;
import org.spongepowered.asm.mixin.Mixin;
import org.spongepowered.asm.mixin.Mutable;
import org.spongepowered.asm.mixin.Shadow;
import org.spongepowered.asm.mixin.injection.At;
import org.spongepowered.asm.mixin.injection.Inject;
import org.spongepowered.asm.mixin.injection.callback.CallbackInfo;
import javax.annotation.Nullable;
import net.minecraft.class_3545;
import net.minecraft.class_4587;
import net.minecraft.class_4588;
import net.minecraft.class_630;
import java.util.HashMap;
import java.util.List;
@Mixin(class_630.class_628.class)
public class CuboidMutator implements MutableCuboid, CuboidSideAccessor {
@Shadow @Final public float minX;
@Shadow @Final public float minY;
@Shadow @Final public float minZ;
@Mutable
@Shadow @Final private class_630.class_593[] sides;
//Store the mutators and the mutator builders.
HashMap mutators = new HashMap<>();
HashMap mutatorBuilders = new HashMap<>();
private class_630.class_593[] originalQuads;
private boolean isSidesSwapped = false;
ICuboidBuilder.Data partData;
@Nullable
ICuboid activeMutator;
@Nullable
String activeMutatorID;
@Inject(method = "", at = @At(value = "RETURN"))
private void constructor(int u, int v, float x, float y, float z, float sizeX, float sizeY, float sizeZ, float extraX, float extraY, float extraZ, boolean mirror, float textureWidth, float textureHeight, CallbackInfo ci){
partData = new ICuboidBuilder.Data(u, v, minX, minY, minZ, sizeX, sizeY, sizeZ, extraX, extraY, extraZ, mirror, textureWidth, textureHeight);
originalQuads = this.sides;
}
@Override
public boolean registerMutator(String name, ICuboidBuilder builder) {
if(mutatorBuilders.containsKey(name)) return false;
if(builder == null) throw new NullPointerException("builder can not be null");
mutatorBuilders.put(name, builder);
return true;
}
@Override
public boolean unregisterMutator(String name) {
if(mutatorBuilders.remove(name) != null){
if(name.equals(activeMutatorID)){
activeMutator = null;
activeMutatorID = null;
}
mutators.remove(name);
return true;
}
return false;
}
@Nullable
@Override
public class_3545 getActiveMutator() {
return activeMutator == null ? null : new class_3545<>(activeMutatorID, activeMutator);
}
@Nullable
@Override
public ICuboid getMutator(String name) {
return mutators.get(name);
}
@Nullable
@Override
public ICuboid getAndActivateMutator(@Nullable String name) {
if(name == null){
activeMutatorID = null;
activeMutator = null;
return null;
}
if(mutatorBuilders.containsKey(name)){
if(!mutators.containsKey(name)){
mutators.put(name, mutatorBuilders.get(name).build(partData));
}
activeMutatorID = name;
return activeMutator = mutators.get(name);
}
return null;
}
@Override
public void copyStateFrom(MutableCuboid other) {
if(other.getActiveMutator() == null){
activeMutator = null;
activeMutatorID = null;
}
else {
if(this.getAndActivateMutator(other.getActiveMutator().method_15442()) != null){
activeMutator.copyState(other.getActiveMutator().method_15441());
}
}
}
@Inject(method = "renderCuboid", at = @At(value = "HEAD"), cancellable = true)
private void renderRedirect(class_4587.class_4665 entry, class_4588 vertexConsumer, int light, int overlay, float red, float green, float blue, float alpha, CallbackInfo ci){
if(getActiveMutator() != null){
getActiveMutator().method_15441().render(entry, vertexConsumer, red, green, blue, alpha, light, overlay);
if(getActiveMutator().method_15441().disableAfterDraw()) {
activeMutator = null; //mutator lives only for one render cycle
activeMutatorID = null;
}
ci.cancel();
}
}
@Override
public void doSideSwapping(){
if(this.getActiveMutator() != null){
List sides = this.getActiveMutator().method_15441().getQuads();
if(sides != null){
this.isSidesSwapped = true;
this.sides = sides.toArray(new class_630.class_593[4]);
}
}
}
@Override
public class_630.class_593[] getSides() {
return this.sides;
}
@Override
public void setSides(class_630.class_593[] sides) {
isSidesSwapped = true;
this.sides = sides;
}
@Override
public void resetSides() {
this.sides = this.originalQuads;
isSidesSwapped = false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy