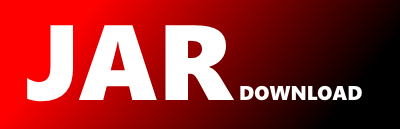
dsh.messages.Envelope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of platform-sdk-java Show documentation
Show all versions of platform-sdk-java Show documentation
The Data Serviceshub (DSH) Platform SDK facilitates easier development of applications on the DSH. It abstracts over the authentication and configuration of your Kafka clients against the policies of the DSH.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: envelope.proto
package dsh.messages;
public final class Envelope {
private Envelope() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* System QOS identifiers
*
*
* Protobuf enum {@code dsh.messages.common.QoS}
*/
public enum QoS
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* might be dropped in case of resources running low (~ highest throughput)
*
*
* BEST_EFFORT = 0;
*/
BEST_EFFORT(0),
/**
*
* will *never* be dropped and retried until success (~ lowest throughput)
*
*
* RELIABLE = 1;
*/
RELIABLE(1),
UNRECOGNIZED(-1),
;
/**
*
* might be dropped in case of resources running low (~ highest throughput)
*
*
* BEST_EFFORT = 0;
*/
public static final int BEST_EFFORT_VALUE = 0;
/**
*
* will *never* be dropped and retried until success (~ lowest throughput)
*
*
* RELIABLE = 1;
*/
public static final int RELIABLE_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static QoS valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static QoS forNumber(int value) {
switch (value) {
case 0: return BEST_EFFORT;
case 1: return RELIABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
QoS> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public QoS findValueByNumber(int number) {
return QoS.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return dsh.messages.Envelope.getDescriptor().getEnumTypes().get(0);
}
private static final QoS[] VALUES = values();
public static QoS valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private QoS(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:dsh.messages.common.QoS)
}
public interface KeyEnvelopeOrBuilder extends
// @@protoc_insertion_point(interface_extends:dsh.messages.common.KeyEnvelope)
com.google.protobuf.MessageOrBuilder {
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return Whether the header field is set.
*/
boolean hasHeader();
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return The header.
*/
dsh.messages.Envelope.KeyHeader getHeader();
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
dsh.messages.Envelope.KeyHeaderOrBuilder getHeaderOrBuilder();
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The key.
*/
java.lang.String getKey();
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The bytes for key.
*/
com.google.protobuf.ByteString
getKeyBytes();
}
/**
* Protobuf type {@code dsh.messages.common.KeyEnvelope}
*/
public static final class KeyEnvelope extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:dsh.messages.common.KeyEnvelope)
KeyEnvelopeOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyEnvelope.newBuilder() to construct.
private KeyEnvelope(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyEnvelope() {
key_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeyEnvelope();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyEnvelope(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
dsh.messages.Envelope.KeyHeader.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(dsh.messages.Envelope.KeyHeader.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyEnvelope_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.KeyEnvelope.class, dsh.messages.Envelope.KeyEnvelope.Builder.class);
}
public static final int HEADER_FIELD_NUMBER = 1;
private dsh.messages.Envelope.KeyHeader header_;
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return Whether the header field is set.
*/
@java.lang.Override
public boolean hasHeader() {
return header_ != null;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return The header.
*/
@java.lang.Override
public dsh.messages.Envelope.KeyHeader getHeader() {
return header_ == null ? dsh.messages.Envelope.KeyHeader.getDefaultInstance() : header_;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
@java.lang.Override
public dsh.messages.Envelope.KeyHeaderOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object key_;
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The key.
*/
@java.lang.Override
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The bytes for key.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (header_ != null) {
output.writeMessage(1, getHeader());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(key_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, key_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getHeader());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(key_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, key_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dsh.messages.Envelope.KeyEnvelope)) {
return super.equals(obj);
}
dsh.messages.Envelope.KeyEnvelope other = (dsh.messages.Envelope.KeyEnvelope) obj;
if (hasHeader() != other.hasHeader()) return false;
if (hasHeader()) {
if (!getHeader()
.equals(other.getHeader())) return false;
}
if (!getKey()
.equals(other.getKey())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.KeyEnvelope parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyEnvelope parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyEnvelope parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dsh.messages.Envelope.KeyEnvelope prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code dsh.messages.common.KeyEnvelope}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:dsh.messages.common.KeyEnvelope)
dsh.messages.Envelope.KeyEnvelopeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyEnvelope_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.KeyEnvelope.class, dsh.messages.Envelope.KeyEnvelope.Builder.class);
}
// Construct using dsh.messages.Envelope.KeyEnvelope.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
key_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyEnvelope_descriptor;
}
@java.lang.Override
public dsh.messages.Envelope.KeyEnvelope getDefaultInstanceForType() {
return dsh.messages.Envelope.KeyEnvelope.getDefaultInstance();
}
@java.lang.Override
public dsh.messages.Envelope.KeyEnvelope build() {
dsh.messages.Envelope.KeyEnvelope result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dsh.messages.Envelope.KeyEnvelope buildPartial() {
dsh.messages.Envelope.KeyEnvelope result = new dsh.messages.Envelope.KeyEnvelope(this);
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
result.key_ = key_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dsh.messages.Envelope.KeyEnvelope) {
return mergeFrom((dsh.messages.Envelope.KeyEnvelope)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dsh.messages.Envelope.KeyEnvelope other) {
if (other == dsh.messages.Envelope.KeyEnvelope.getDefaultInstance()) return this;
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
dsh.messages.Envelope.KeyEnvelope parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (dsh.messages.Envelope.KeyEnvelope) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private dsh.messages.Envelope.KeyHeader header_;
private com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.KeyHeader, dsh.messages.Envelope.KeyHeader.Builder, dsh.messages.Envelope.KeyHeaderOrBuilder> headerBuilder_;
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return Whether the header field is set.
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
* @return The header.
*/
public dsh.messages.Envelope.KeyHeader getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? dsh.messages.Envelope.KeyHeader.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public Builder setHeader(dsh.messages.Envelope.KeyHeader value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public Builder setHeader(
dsh.messages.Envelope.KeyHeader.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public Builder mergeHeader(dsh.messages.Envelope.KeyHeader value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
dsh.messages.Envelope.KeyHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public dsh.messages.Envelope.KeyHeader.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
public dsh.messages.Envelope.KeyHeaderOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
dsh.messages.Envelope.KeyHeader.getDefaultInstance() : header_;
}
}
/**
*
* header is mandatory on stream topics
*
*
* .dsh.messages.common.KeyHeader header = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.KeyHeader, dsh.messages.Envelope.KeyHeader.Builder, dsh.messages.Envelope.KeyHeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.KeyHeader, dsh.messages.Envelope.KeyHeader.Builder, dsh.messages.Envelope.KeyHeaderOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private java.lang.Object key_ = "";
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The key.
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return The bytes for key.
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
*
* MQTT topic (minus prefixes) on stream topics
*
*
* string key = 2;
* @param value The bytes for key to set.
* @return This builder for chaining.
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:dsh.messages.common.KeyEnvelope)
}
// @@protoc_insertion_point(class_scope:dsh.messages.common.KeyEnvelope)
private static final dsh.messages.Envelope.KeyEnvelope DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dsh.messages.Envelope.KeyEnvelope();
}
public static dsh.messages.Envelope.KeyEnvelope getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeyEnvelope parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyEnvelope(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dsh.messages.Envelope.KeyEnvelope getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KeyHeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:dsh.messages.common.KeyHeader)
com.google.protobuf.MessageOrBuilder {
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return The identifier.
*/
dsh.messages.Envelope.Identity getIdentifier();
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
dsh.messages.Envelope.IdentityOrBuilder getIdentifierOrBuilder();
/**
*
* marks the message as 'retained'
* this makes the Latest Value Store keep a copy of the message in memory for later retrieval
*
*
* bool retained = 2;
* @return The retained.
*/
boolean getRetained();
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The enum numeric value on the wire for qos.
*/
int getQosValue();
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The qos.
*/
dsh.messages.Envelope.QoS getQos();
}
/**
* Protobuf type {@code dsh.messages.common.KeyHeader}
*/
public static final class KeyHeader extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:dsh.messages.common.KeyHeader)
KeyHeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyHeader.newBuilder() to construct.
private KeyHeader(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyHeader() {
qos_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeyHeader();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyHeader(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
dsh.messages.Envelope.Identity.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(dsh.messages.Envelope.Identity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 16: {
retained_ = input.readBool();
break;
}
case 24: {
int rawValue = input.readEnum();
qos_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.KeyHeader.class, dsh.messages.Envelope.KeyHeader.Builder.class);
}
public static final int IDENTIFIER_FIELD_NUMBER = 1;
private dsh.messages.Envelope.Identity identifier_;
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return The identifier.
*/
@java.lang.Override
public dsh.messages.Envelope.Identity getIdentifier() {
return identifier_ == null ? dsh.messages.Envelope.Identity.getDefaultInstance() : identifier_;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
@java.lang.Override
public dsh.messages.Envelope.IdentityOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int RETAINED_FIELD_NUMBER = 2;
private boolean retained_;
/**
*
* marks the message as 'retained'
* this makes the Latest Value Store keep a copy of the message in memory for later retrieval
*
*
* bool retained = 2;
* @return The retained.
*/
@java.lang.Override
public boolean getRetained() {
return retained_;
}
public static final int QOS_FIELD_NUMBER = 3;
private int qos_;
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The enum numeric value on the wire for qos.
*/
@java.lang.Override public int getQosValue() {
return qos_;
}
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The qos.
*/
@java.lang.Override public dsh.messages.Envelope.QoS getQos() {
@SuppressWarnings("deprecation")
dsh.messages.Envelope.QoS result = dsh.messages.Envelope.QoS.valueOf(qos_);
return result == null ? dsh.messages.Envelope.QoS.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (identifier_ != null) {
output.writeMessage(1, getIdentifier());
}
if (retained_ != false) {
output.writeBool(2, retained_);
}
if (qos_ != dsh.messages.Envelope.QoS.BEST_EFFORT.getNumber()) {
output.writeEnum(3, qos_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getIdentifier());
}
if (retained_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, retained_);
}
if (qos_ != dsh.messages.Envelope.QoS.BEST_EFFORT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, qos_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dsh.messages.Envelope.KeyHeader)) {
return super.equals(obj);
}
dsh.messages.Envelope.KeyHeader other = (dsh.messages.Envelope.KeyHeader) obj;
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (getRetained()
!= other.getRetained()) return false;
if (qos_ != other.qos_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + RETAINED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRetained());
hash = (37 * hash) + QOS_FIELD_NUMBER;
hash = (53 * hash) + qos_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.KeyHeader parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyHeader parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.KeyHeader parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dsh.messages.Envelope.KeyHeader prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code dsh.messages.common.KeyHeader}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:dsh.messages.common.KeyHeader)
dsh.messages.Envelope.KeyHeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.KeyHeader.class, dsh.messages.Envelope.KeyHeader.Builder.class);
}
// Construct using dsh.messages.Envelope.KeyHeader.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
retained_ = false;
qos_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_KeyHeader_descriptor;
}
@java.lang.Override
public dsh.messages.Envelope.KeyHeader getDefaultInstanceForType() {
return dsh.messages.Envelope.KeyHeader.getDefaultInstance();
}
@java.lang.Override
public dsh.messages.Envelope.KeyHeader build() {
dsh.messages.Envelope.KeyHeader result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dsh.messages.Envelope.KeyHeader buildPartial() {
dsh.messages.Envelope.KeyHeader result = new dsh.messages.Envelope.KeyHeader(this);
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.retained_ = retained_;
result.qos_ = qos_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dsh.messages.Envelope.KeyHeader) {
return mergeFrom((dsh.messages.Envelope.KeyHeader)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dsh.messages.Envelope.KeyHeader other) {
if (other == dsh.messages.Envelope.KeyHeader.getDefaultInstance()) return this;
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (other.getRetained() != false) {
setRetained(other.getRetained());
}
if (other.qos_ != 0) {
setQosValue(other.getQosValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
dsh.messages.Envelope.KeyHeader parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (dsh.messages.Envelope.KeyHeader) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private dsh.messages.Envelope.Identity identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.Identity, dsh.messages.Envelope.Identity.Builder, dsh.messages.Envelope.IdentityOrBuilder> identifierBuilder_;
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
* @return The identifier.
*/
public dsh.messages.Envelope.Identity getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? dsh.messages.Envelope.Identity.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public Builder setIdentifier(dsh.messages.Envelope.Identity value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public Builder setIdentifier(
dsh.messages.Envelope.Identity.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public Builder mergeIdentifier(dsh.messages.Envelope.Identity value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
dsh.messages.Envelope.Identity.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public dsh.messages.Envelope.Identity.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
public dsh.messages.Envelope.IdentityOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
dsh.messages.Envelope.Identity.getDefaultInstance() : identifier_;
}
}
/**
*
* identifies the message origin
*
*
* .dsh.messages.common.Identity identifier = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.Identity, dsh.messages.Envelope.Identity.Builder, dsh.messages.Envelope.IdentityOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
dsh.messages.Envelope.Identity, dsh.messages.Envelope.Identity.Builder, dsh.messages.Envelope.IdentityOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private boolean retained_ ;
/**
*
* marks the message as 'retained'
* this makes the Latest Value Store keep a copy of the message in memory for later retrieval
*
*
* bool retained = 2;
* @return The retained.
*/
@java.lang.Override
public boolean getRetained() {
return retained_;
}
/**
*
* marks the message as 'retained'
* this makes the Latest Value Store keep a copy of the message in memory for later retrieval
*
*
* bool retained = 2;
* @param value The retained to set.
* @return This builder for chaining.
*/
public Builder setRetained(boolean value) {
retained_ = value;
onChanged();
return this;
}
/**
*
* marks the message as 'retained'
* this makes the Latest Value Store keep a copy of the message in memory for later retrieval
*
*
* bool retained = 2;
* @return This builder for chaining.
*/
public Builder clearRetained() {
retained_ = false;
onChanged();
return this;
}
private int qos_ = 0;
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The enum numeric value on the wire for qos.
*/
@java.lang.Override public int getQosValue() {
return qos_;
}
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @param value The enum numeric value on the wire for qos to set.
* @return This builder for chaining.
*/
public Builder setQosValue(int value) {
qos_ = value;
onChanged();
return this;
}
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return The qos.
*/
@java.lang.Override
public dsh.messages.Envelope.QoS getQos() {
@SuppressWarnings("deprecation")
dsh.messages.Envelope.QoS result = dsh.messages.Envelope.QoS.valueOf(qos_);
return result == null ? dsh.messages.Envelope.QoS.UNRECOGNIZED : result;
}
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @param value The qos to set.
* @return This builder for chaining.
*/
public Builder setQos(dsh.messages.Envelope.QoS value) {
if (value == null) {
throw new NullPointerException();
}
qos_ = value.getNumber();
onChanged();
return this;
}
/**
*
* the QOS with wich the message is handled within the system
*
*
* .dsh.messages.common.QoS qos = 3;
* @return This builder for chaining.
*/
public Builder clearQos() {
qos_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:dsh.messages.common.KeyHeader)
}
// @@protoc_insertion_point(class_scope:dsh.messages.common.KeyHeader)
private static final dsh.messages.Envelope.KeyHeader DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dsh.messages.Envelope.KeyHeader();
}
public static dsh.messages.Envelope.KeyHeader getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeyHeader parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyHeader(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dsh.messages.Envelope.KeyHeader getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IdentityOrBuilder extends
// @@protoc_insertion_point(interface_extends:dsh.messages.common.Identity)
com.google.protobuf.MessageOrBuilder {
/**
* string tenant = 1;
* @return The tenant.
*/
java.lang.String getTenant();
/**
* string tenant = 1;
* @return The bytes for tenant.
*/
com.google.protobuf.ByteString
getTenantBytes();
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return Whether the freeForm field is set.
*/
boolean hasFreeForm();
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The freeForm.
*/
java.lang.String getFreeForm();
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The bytes for freeForm.
*/
com.google.protobuf.ByteString
getFreeFormBytes();
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return Whether the user field is set.
*/
boolean hasUser();
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The user.
*/
java.lang.String getUser();
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The bytes for user.
*/
com.google.protobuf.ByteString
getUserBytes();
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return Whether the client field is set.
*/
boolean hasClient();
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The client.
*/
java.lang.String getClient();
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The bytes for client.
*/
com.google.protobuf.ByteString
getClientBytes();
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return Whether the application field is set.
*/
boolean hasApplication();
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The application.
*/
java.lang.String getApplication();
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The bytes for application.
*/
com.google.protobuf.ByteString
getApplicationBytes();
public dsh.messages.Envelope.Identity.PublisherCase getPublisherCase();
}
/**
*
* identifies the data origin
*
*
* Protobuf type {@code dsh.messages.common.Identity}
*/
public static final class Identity extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:dsh.messages.common.Identity)
IdentityOrBuilder {
private static final long serialVersionUID = 0L;
// Use Identity.newBuilder() to construct.
private Identity(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Identity() {
tenant_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Identity();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Identity(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
tenant_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
publisherCase_ = 2;
publisher_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
publisherCase_ = 3;
publisher_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
publisherCase_ = 4;
publisher_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
publisherCase_ = 5;
publisher_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_Identity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_Identity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.Identity.class, dsh.messages.Envelope.Identity.Builder.class);
}
private int publisherCase_ = 0;
private java.lang.Object publisher_;
public enum PublisherCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
FREE_FORM(2),
USER(3),
CLIENT(4),
APPLICATION(5),
PUBLISHER_NOT_SET(0);
private final int value;
private PublisherCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PublisherCase valueOf(int value) {
return forNumber(value);
}
public static PublisherCase forNumber(int value) {
switch (value) {
case 2: return FREE_FORM;
case 3: return USER;
case 4: return CLIENT;
case 5: return APPLICATION;
case 0: return PUBLISHER_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PublisherCase
getPublisherCase() {
return PublisherCase.forNumber(
publisherCase_);
}
public static final int TENANT_FIELD_NUMBER = 1;
private volatile java.lang.Object tenant_;
/**
* string tenant = 1;
* @return The tenant.
*/
@java.lang.Override
public java.lang.String getTenant() {
java.lang.Object ref = tenant_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tenant_ = s;
return s;
}
}
/**
* string tenant = 1;
* @return The bytes for tenant.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTenantBytes() {
java.lang.Object ref = tenant_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tenant_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FREE_FORM_FIELD_NUMBER = 2;
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return Whether the freeForm field is set.
*/
public boolean hasFreeForm() {
return publisherCase_ == 2;
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The freeForm.
*/
public java.lang.String getFreeForm() {
java.lang.Object ref = "";
if (publisherCase_ == 2) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 2) {
publisher_ = s;
}
return s;
}
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The bytes for freeForm.
*/
public com.google.protobuf.ByteString
getFreeFormBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 2) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 2) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_FIELD_NUMBER = 3;
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return Whether the user field is set.
*/
public boolean hasUser() {
return publisherCase_ == 3;
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The user.
*/
public java.lang.String getUser() {
java.lang.Object ref = "";
if (publisherCase_ == 3) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 3) {
publisher_ = s;
}
return s;
}
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The bytes for user.
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 3) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 3) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_FIELD_NUMBER = 4;
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return Whether the client field is set.
*/
public boolean hasClient() {
return publisherCase_ == 4;
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The client.
*/
public java.lang.String getClient() {
java.lang.Object ref = "";
if (publisherCase_ == 4) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 4) {
publisher_ = s;
}
return s;
}
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The bytes for client.
*/
public com.google.protobuf.ByteString
getClientBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 4) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 4) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPLICATION_FIELD_NUMBER = 5;
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return Whether the application field is set.
*/
public boolean hasApplication() {
return publisherCase_ == 5;
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The application.
*/
public java.lang.String getApplication() {
java.lang.Object ref = "";
if (publisherCase_ == 5) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 5) {
publisher_ = s;
}
return s;
}
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The bytes for application.
*/
public com.google.protobuf.ByteString
getApplicationBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 5) {
ref = publisher_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 5) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tenant_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, tenant_);
}
if (publisherCase_ == 2) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, publisher_);
}
if (publisherCase_ == 3) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, publisher_);
}
if (publisherCase_ == 4) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, publisher_);
}
if (publisherCase_ == 5) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, publisher_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tenant_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, tenant_);
}
if (publisherCase_ == 2) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, publisher_);
}
if (publisherCase_ == 3) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, publisher_);
}
if (publisherCase_ == 4) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, publisher_);
}
if (publisherCase_ == 5) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, publisher_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dsh.messages.Envelope.Identity)) {
return super.equals(obj);
}
dsh.messages.Envelope.Identity other = (dsh.messages.Envelope.Identity) obj;
if (!getTenant()
.equals(other.getTenant())) return false;
if (!getPublisherCase().equals(other.getPublisherCase())) return false;
switch (publisherCase_) {
case 2:
if (!getFreeForm()
.equals(other.getFreeForm())) return false;
break;
case 3:
if (!getUser()
.equals(other.getUser())) return false;
break;
case 4:
if (!getClient()
.equals(other.getClient())) return false;
break;
case 5:
if (!getApplication()
.equals(other.getApplication())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TENANT_FIELD_NUMBER;
hash = (53 * hash) + getTenant().hashCode();
switch (publisherCase_) {
case 2:
hash = (37 * hash) + FREE_FORM_FIELD_NUMBER;
hash = (53 * hash) + getFreeForm().hashCode();
break;
case 3:
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
break;
case 4:
hash = (37 * hash) + CLIENT_FIELD_NUMBER;
hash = (53 * hash) + getClient().hashCode();
break;
case 5:
hash = (37 * hash) + APPLICATION_FIELD_NUMBER;
hash = (53 * hash) + getApplication().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static dsh.messages.Envelope.Identity parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.Identity parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.Identity parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.Identity parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.Identity parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.Identity parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.Identity parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.Identity parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.Identity parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.Identity parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.Identity parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.Identity parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dsh.messages.Envelope.Identity prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* identifies the data origin
*
*
* Protobuf type {@code dsh.messages.common.Identity}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:dsh.messages.common.Identity)
dsh.messages.Envelope.IdentityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_Identity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_Identity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.Identity.class, dsh.messages.Envelope.Identity.Builder.class);
}
// Construct using dsh.messages.Envelope.Identity.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tenant_ = "";
publisherCase_ = 0;
publisher_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_Identity_descriptor;
}
@java.lang.Override
public dsh.messages.Envelope.Identity getDefaultInstanceForType() {
return dsh.messages.Envelope.Identity.getDefaultInstance();
}
@java.lang.Override
public dsh.messages.Envelope.Identity build() {
dsh.messages.Envelope.Identity result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dsh.messages.Envelope.Identity buildPartial() {
dsh.messages.Envelope.Identity result = new dsh.messages.Envelope.Identity(this);
result.tenant_ = tenant_;
if (publisherCase_ == 2) {
result.publisher_ = publisher_;
}
if (publisherCase_ == 3) {
result.publisher_ = publisher_;
}
if (publisherCase_ == 4) {
result.publisher_ = publisher_;
}
if (publisherCase_ == 5) {
result.publisher_ = publisher_;
}
result.publisherCase_ = publisherCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dsh.messages.Envelope.Identity) {
return mergeFrom((dsh.messages.Envelope.Identity)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dsh.messages.Envelope.Identity other) {
if (other == dsh.messages.Envelope.Identity.getDefaultInstance()) return this;
if (!other.getTenant().isEmpty()) {
tenant_ = other.tenant_;
onChanged();
}
switch (other.getPublisherCase()) {
case FREE_FORM: {
publisherCase_ = 2;
publisher_ = other.publisher_;
onChanged();
break;
}
case USER: {
publisherCase_ = 3;
publisher_ = other.publisher_;
onChanged();
break;
}
case CLIENT: {
publisherCase_ = 4;
publisher_ = other.publisher_;
onChanged();
break;
}
case APPLICATION: {
publisherCase_ = 5;
publisher_ = other.publisher_;
onChanged();
break;
}
case PUBLISHER_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
dsh.messages.Envelope.Identity parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (dsh.messages.Envelope.Identity) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int publisherCase_ = 0;
private java.lang.Object publisher_;
public PublisherCase
getPublisherCase() {
return PublisherCase.forNumber(
publisherCase_);
}
public Builder clearPublisher() {
publisherCase_ = 0;
publisher_ = null;
onChanged();
return this;
}
private java.lang.Object tenant_ = "";
/**
* string tenant = 1;
* @return The tenant.
*/
public java.lang.String getTenant() {
java.lang.Object ref = tenant_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tenant_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string tenant = 1;
* @return The bytes for tenant.
*/
public com.google.protobuf.ByteString
getTenantBytes() {
java.lang.Object ref = tenant_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tenant_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string tenant = 1;
* @param value The tenant to set.
* @return This builder for chaining.
*/
public Builder setTenant(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tenant_ = value;
onChanged();
return this;
}
/**
* string tenant = 1;
* @return This builder for chaining.
*/
public Builder clearTenant() {
tenant_ = getDefaultInstance().getTenant();
onChanged();
return this;
}
/**
* string tenant = 1;
* @param value The bytes for tenant to set.
* @return This builder for chaining.
*/
public Builder setTenantBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tenant_ = value;
onChanged();
return this;
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return Whether the freeForm field is set.
*/
@java.lang.Override
public boolean hasFreeForm() {
return publisherCase_ == 2;
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The freeForm.
*/
@java.lang.Override
public java.lang.String getFreeForm() {
java.lang.Object ref = "";
if (publisherCase_ == 2) {
ref = publisher_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 2) {
publisher_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return The bytes for freeForm.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFreeFormBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 2) {
ref = publisher_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 2) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @param value The freeForm to set.
* @return This builder for chaining.
*/
public Builder setFreeForm(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
publisherCase_ = 2;
publisher_ = value;
onChanged();
return this;
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @return This builder for chaining.
*/
public Builder clearFreeForm() {
if (publisherCase_ == 2) {
publisherCase_ = 0;
publisher_ = null;
onChanged();
}
return this;
}
/**
*
* for 'backwards' compatibility or produces not matching the publishers below
*
*
* string free_form = 2;
* @param value The bytes for freeForm to set.
* @return This builder for chaining.
*/
public Builder setFreeFormBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
publisherCase_ = 2;
publisher_ = value;
onChanged();
return this;
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return Whether the user field is set.
*/
@java.lang.Override
public boolean hasUser() {
return publisherCase_ == 3;
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The user.
*/
@java.lang.Override
public java.lang.String getUser() {
java.lang.Object ref = "";
if (publisherCase_ == 3) {
ref = publisher_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 3) {
publisher_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return The bytes for user.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 3) {
ref = publisher_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 3) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @param value The user to set.
* @return This builder for chaining.
*/
public Builder setUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
publisherCase_ = 3;
publisher_ = value;
onChanged();
return this;
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @return This builder for chaining.
*/
public Builder clearUser() {
if (publisherCase_ == 3) {
publisherCase_ = 0;
publisher_ = null;
onChanged();
}
return this;
}
/**
*
* reserved for MQTT side publishes ('humans')
*
*
* string user = 3;
* @param value The bytes for user to set.
* @return This builder for chaining.
*/
public Builder setUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
publisherCase_ = 3;
publisher_ = value;
onChanged();
return this;
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return Whether the client field is set.
*/
@java.lang.Override
public boolean hasClient() {
return publisherCase_ == 4;
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The client.
*/
@java.lang.Override
public java.lang.String getClient() {
java.lang.Object ref = "";
if (publisherCase_ == 4) {
ref = publisher_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 4) {
publisher_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return The bytes for client.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 4) {
ref = publisher_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 4) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @param value The client to set.
* @return This builder for chaining.
*/
public Builder setClient(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
publisherCase_ = 4;
publisher_ = value;
onChanged();
return this;
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @return This builder for chaining.
*/
public Builder clearClient() {
if (publisherCase_ == 4) {
publisherCase_ = 0;
publisher_ = null;
onChanged();
}
return this;
}
/**
*
* reserved for MQTT side publishes ('devices')
*
*
* string client = 4;
* @param value The bytes for client to set.
* @return This builder for chaining.
*/
public Builder setClientBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
publisherCase_ = 4;
publisher_ = value;
onChanged();
return this;
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return Whether the application field is set.
*/
@java.lang.Override
public boolean hasApplication() {
return publisherCase_ == 5;
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The application.
*/
@java.lang.Override
public java.lang.String getApplication() {
java.lang.Object ref = "";
if (publisherCase_ == 5) {
ref = publisher_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (publisherCase_ == 5) {
publisher_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return The bytes for application.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApplicationBytes() {
java.lang.Object ref = "";
if (publisherCase_ == 5) {
ref = publisher_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (publisherCase_ == 5) {
publisher_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @param value The application to set.
* @return This builder for chaining.
*/
public Builder setApplication(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
publisherCase_ = 5;
publisher_ = value;
onChanged();
return this;
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @return This builder for chaining.
*/
public Builder clearApplication() {
if (publisherCase_ == 5) {
publisherCase_ = 0;
publisher_ = null;
onChanged();
}
return this;
}
/**
*
* to be used when producing data from containers
*
*
* string application = 5;
* @param value The bytes for application to set.
* @return This builder for chaining.
*/
public Builder setApplicationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
publisherCase_ = 5;
publisher_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:dsh.messages.common.Identity)
}
// @@protoc_insertion_point(class_scope:dsh.messages.common.Identity)
private static final dsh.messages.Envelope.Identity DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dsh.messages.Envelope.Identity();
}
public static dsh.messages.Envelope.Identity getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Identity parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Identity(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dsh.messages.Envelope.Identity getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataEnvelopeOrBuilder extends
// @@protoc_insertion_point(interface_extends:dsh.messages.common.DataEnvelope)
com.google.protobuf.MessageOrBuilder {
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return Whether the payload field is set.
*/
boolean hasPayload();
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return The payload.
*/
com.google.protobuf.ByteString getPayload();
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
int getTracingCount();
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
boolean containsTracing(
java.lang.String key);
/**
* Use {@link #getTracingMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getTracing();
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
java.util.Map
getTracingMap();
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
java.lang.String getTracingOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
java.lang.String getTracingOrThrow(
java.lang.String key);
public dsh.messages.Envelope.DataEnvelope.KindCase getKindCase();
}
/**
* Protobuf type {@code dsh.messages.common.DataEnvelope}
*/
public static final class DataEnvelope extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:dsh.messages.common.DataEnvelope)
DataEnvelopeOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataEnvelope.newBuilder() to construct.
private DataEnvelope(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataEnvelope() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DataEnvelope();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataEnvelope(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
kind_ = input.readBytes();
kindCase_ = 1;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tracing_ = com.google.protobuf.MapField.newMapField(
TracingDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
tracing__ = input.readMessage(
TracingDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
tracing_.getMutableMap().put(
tracing__.getKey(), tracing__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTracing();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.DataEnvelope.class, dsh.messages.Envelope.DataEnvelope.Builder.class);
}
private int kindCase_ = 0;
private java.lang.Object kind_;
public enum KindCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PAYLOAD(1),
KIND_NOT_SET(0);
private final int value;
private KindCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static KindCase valueOf(int value) {
return forNumber(value);
}
public static KindCase forNumber(int value) {
switch (value) {
case 1: return PAYLOAD;
case 0: return KIND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public KindCase
getKindCase() {
return KindCase.forNumber(
kindCase_);
}
public static final int PAYLOAD_FIELD_NUMBER = 1;
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return Whether the payload field is set.
*/
@java.lang.Override
public boolean hasPayload() {
return kindCase_ == 1;
}
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
if (kindCase_ == 1) {
return (com.google.protobuf.ByteString) kind_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int TRACING_FIELD_NUMBER = 2;
private static final class TracingDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_TracingEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> tracing_;
private com.google.protobuf.MapField
internalGetTracing() {
if (tracing_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TracingDefaultEntryHolder.defaultEntry);
}
return tracing_;
}
public int getTracingCount() {
return internalGetTracing().getMap().size();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public boolean containsTracing(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTracing().getMap().containsKey(key);
}
/**
* Use {@link #getTracingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTracing() {
return getTracingMap();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.util.Map getTracingMap() {
return internalGetTracing().getMap();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.lang.String getTracingOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTracing().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.lang.String getTracingOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTracing().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (kindCase_ == 1) {
output.writeBytes(
1, (com.google.protobuf.ByteString) kind_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetTracing(),
TracingDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (kindCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
1, (com.google.protobuf.ByteString) kind_);
}
for (java.util.Map.Entry entry
: internalGetTracing().getMap().entrySet()) {
com.google.protobuf.MapEntry
tracing__ = TracingDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, tracing__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dsh.messages.Envelope.DataEnvelope)) {
return super.equals(obj);
}
dsh.messages.Envelope.DataEnvelope other = (dsh.messages.Envelope.DataEnvelope) obj;
if (!internalGetTracing().equals(
other.internalGetTracing())) return false;
if (!getKindCase().equals(other.getKindCase())) return false;
switch (kindCase_) {
case 1:
if (!getPayload()
.equals(other.getPayload())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetTracing().getMap().isEmpty()) {
hash = (37 * hash) + TRACING_FIELD_NUMBER;
hash = (53 * hash) + internalGetTracing().hashCode();
}
switch (kindCase_) {
case 1:
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.DataEnvelope parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.DataEnvelope parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static dsh.messages.Envelope.DataEnvelope parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dsh.messages.Envelope.DataEnvelope prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code dsh.messages.common.DataEnvelope}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:dsh.messages.common.DataEnvelope)
dsh.messages.Envelope.DataEnvelopeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTracing();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableTracing();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dsh.messages.Envelope.DataEnvelope.class, dsh.messages.Envelope.DataEnvelope.Builder.class);
}
// Construct using dsh.messages.Envelope.DataEnvelope.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
internalGetMutableTracing().clear();
kindCase_ = 0;
kind_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dsh.messages.Envelope.internal_static_dsh_messages_common_DataEnvelope_descriptor;
}
@java.lang.Override
public dsh.messages.Envelope.DataEnvelope getDefaultInstanceForType() {
return dsh.messages.Envelope.DataEnvelope.getDefaultInstance();
}
@java.lang.Override
public dsh.messages.Envelope.DataEnvelope build() {
dsh.messages.Envelope.DataEnvelope result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dsh.messages.Envelope.DataEnvelope buildPartial() {
dsh.messages.Envelope.DataEnvelope result = new dsh.messages.Envelope.DataEnvelope(this);
int from_bitField0_ = bitField0_;
if (kindCase_ == 1) {
result.kind_ = kind_;
}
result.tracing_ = internalGetTracing();
result.tracing_.makeImmutable();
result.kindCase_ = kindCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dsh.messages.Envelope.DataEnvelope) {
return mergeFrom((dsh.messages.Envelope.DataEnvelope)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dsh.messages.Envelope.DataEnvelope other) {
if (other == dsh.messages.Envelope.DataEnvelope.getDefaultInstance()) return this;
internalGetMutableTracing().mergeFrom(
other.internalGetTracing());
switch (other.getKindCase()) {
case PAYLOAD: {
setPayload(other.getPayload());
break;
}
case KIND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
dsh.messages.Envelope.DataEnvelope parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (dsh.messages.Envelope.DataEnvelope) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int kindCase_ = 0;
private java.lang.Object kind_;
public KindCase
getKindCase() {
return KindCase.forNumber(
kindCase_);
}
public Builder clearKind() {
kindCase_ = 0;
kind_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return Whether the payload field is set.
*/
public boolean hasPayload() {
return kindCase_ == 1;
}
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return The payload.
*/
public com.google.protobuf.ByteString getPayload() {
if (kindCase_ == 1) {
return (com.google.protobuf.ByteString) kind_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @param value The payload to set.
* @return This builder for chaining.
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
kindCase_ = 1;
kind_ = value;
onChanged();
return this;
}
/**
*
* leave empty for DELETE semantics in Latest Value Store
*
*
* bytes payload = 1;
* @return This builder for chaining.
*/
public Builder clearPayload() {
if (kindCase_ == 1) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> tracing_;
private com.google.protobuf.MapField
internalGetTracing() {
if (tracing_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TracingDefaultEntryHolder.defaultEntry);
}
return tracing_;
}
private com.google.protobuf.MapField
internalGetMutableTracing() {
onChanged();;
if (tracing_ == null) {
tracing_ = com.google.protobuf.MapField.newMapField(
TracingDefaultEntryHolder.defaultEntry);
}
if (!tracing_.isMutable()) {
tracing_ = tracing_.copy();
}
return tracing_;
}
public int getTracingCount() {
return internalGetTracing().getMap().size();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public boolean containsTracing(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTracing().getMap().containsKey(key);
}
/**
* Use {@link #getTracingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTracing() {
return getTracingMap();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.util.Map getTracingMap() {
return internalGetTracing().getMap();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.lang.String getTracingOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTracing().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
@java.lang.Override
public java.lang.String getTracingOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTracing().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearTracing() {
internalGetMutableTracing().getMutableMap()
.clear();
return this;
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
public Builder removeTracing(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableTracing().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableTracing() {
return internalGetMutableTracing().getMutableMap();
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
public Builder putTracing(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableTracing().getMutableMap()
.put(key, value);
return this;
}
/**
*
* tracing data: used for passing span contexts between applications
*
*
* map<string, string> tracing = 2;
*/
public Builder putAllTracing(
java.util.Map values) {
internalGetMutableTracing().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:dsh.messages.common.DataEnvelope)
}
// @@protoc_insertion_point(class_scope:dsh.messages.common.DataEnvelope)
private static final dsh.messages.Envelope.DataEnvelope DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dsh.messages.Envelope.DataEnvelope();
}
public static dsh.messages.Envelope.DataEnvelope getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DataEnvelope parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataEnvelope(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dsh.messages.Envelope.DataEnvelope getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_dsh_messages_common_KeyEnvelope_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_dsh_messages_common_KeyEnvelope_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_dsh_messages_common_KeyHeader_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_dsh_messages_common_KeyHeader_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_dsh_messages_common_Identity_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_dsh_messages_common_Identity_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_dsh_messages_common_DataEnvelope_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_dsh_messages_common_DataEnvelope_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_dsh_messages_common_DataEnvelope_TracingEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_dsh_messages_common_DataEnvelope_TracingEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016envelope.proto\022\023dsh.messages.common\"P\n" +
"\013KeyEnvelope\022.\n\006header\030\001 \001(\0132\036.dsh.messa" +
"ges.common.KeyHeader\022\013\n\003key\030\002 \001(\tJ\004\010\003\020\004\"" +
"w\n\tKeyHeader\0221\n\nidentifier\030\001 \001(\0132\035.dsh.m" +
"essages.common.Identity\022\020\n\010retained\030\002 \001(" +
"\010\022%\n\003qos\030\003 \001(\0162\030.dsh.messages.common.QoS" +
"\"u\n\010Identity\022\016\n\006tenant\030\001 \001(\t\022\023\n\tfree_for" +
"m\030\002 \001(\tH\000\022\016\n\004user\030\003 \001(\tH\000\022\020\n\006client\030\004 \001(" +
"\tH\000\022\025\n\013application\030\005 \001(\tH\000B\013\n\tpublisher\"" +
"\232\001\n\014DataEnvelope\022\021\n\007payload\030\001 \001(\014H\000\022?\n\007t" +
"racing\030\002 \003(\0132..dsh.messages.common.DataE" +
"nvelope.TracingEntry\032.\n\014TracingEntry\022\013\n\003" +
"key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001B\006\n\004kind*$\n\003" +
"QoS\022\017\n\013BEST_EFFORT\020\000\022\014\n\010RELIABLE\020\001B\016\n\014ds" +
"h.messagesb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_dsh_messages_common_KeyEnvelope_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_dsh_messages_common_KeyEnvelope_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_dsh_messages_common_KeyEnvelope_descriptor,
new java.lang.String[] { "Header", "Key", });
internal_static_dsh_messages_common_KeyHeader_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_dsh_messages_common_KeyHeader_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_dsh_messages_common_KeyHeader_descriptor,
new java.lang.String[] { "Identifier", "Retained", "Qos", });
internal_static_dsh_messages_common_Identity_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_dsh_messages_common_Identity_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_dsh_messages_common_Identity_descriptor,
new java.lang.String[] { "Tenant", "FreeForm", "User", "Client", "Application", "Publisher", });
internal_static_dsh_messages_common_DataEnvelope_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_dsh_messages_common_DataEnvelope_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_dsh_messages_common_DataEnvelope_descriptor,
new java.lang.String[] { "Payload", "Tracing", "Kind", });
internal_static_dsh_messages_common_DataEnvelope_TracingEntry_descriptor =
internal_static_dsh_messages_common_DataEnvelope_descriptor.getNestedTypes().get(0);
internal_static_dsh_messages_common_DataEnvelope_TracingEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_dsh_messages_common_DataEnvelope_TracingEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy