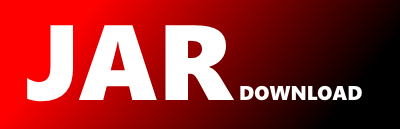
dsh.rest.SimpleRestServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of platform-sdk-java Show documentation
Show all versions of platform-sdk-java Show documentation
The Data Serviceshub (DSH) Platform SDK facilitates easier development of applications on the DSH. It abstracts over the authentication and configuration of your Kafka clients against the policies of the DSH.
package dsh.rest;
import com.sun.net.httpserver.HttpServer;
import dsh.sdk.internal.HttpUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.Closeable;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetSocketAddress;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.Executors;
import java.util.function.Consumer;
import java.util.function.Supplier;
/**
* A convenience class to easily create a HTTP REST service from within your application.
*
* This REST service is tuned for usage as metrics scraper and health reporter.
* In order to fulfill the requirements of returning a body in the response, it is possible to hook up
* a function to the service to supply a {@code Boolean} answer (used for health reporting),
* or an answer with a {@code String} body (use for metric reporting).
*
* By default the service can handle functions (@see Supplier) that return a {@code String} or a {@code Boolean}.
*
* {@code
*
* boolean isHealth() { ... }
* String scrapeMetrics() { ... }
*
* Service myServer = new SimpleRestServer.Builder()
* .setListener("/health", () -> this.isHealthy())
* .setListener("/metrics", () -> this.scrapeMetrics())
*
* myServer.start();
*
* }
*/
public class SimpleRestServer extends Service {
private static Logger logger = LoggerFactory.getLogger(SimpleRestServer.class);
/**
* Builder class to configure thr REST Server
*/
public static class Builder extends Service.Builder{
private Integer port;
private Map> services = new HashMap<>();
/**
* Assign a specific port the server will listen on.
*
* @param port port to listen on
* @return Service Builder
*/
public Builder setPort(Integer port) {
this.port = port;
return this;
}
/**
* Assign a result supplier for a given path for the HTTP Service.
*
* @param path the path for which this supplier will provide the result
* @param provider the provider to calculate the result
* @param result type for the result supplier
* Boolean for 200OK, 500ServiceUnavailable results
* String for 200OK with result body
* @return Service Builder
*/
public Builder setListener(String path, Supplier provider) {
services.putIfAbsent(path, provider);
return this;
}
/**
* Creates the HTTP REST server with the specified configuration options.
*
* @return a server that can be started and stopped
* @exception ServiceConfigException when no available free listening ports can be found
*/
@Override public Service build() throws ServiceConfigException {
SimpleRestServer rest = new SimpleRestServer();
try {
rest.server = HttpServer.create(new InetSocketAddress(this.port == null ? HttpUtils.freePort() : this.port), 0);
rest.server.setExecutor(Executors.newSingleThreadExecutor());
}
catch (IOException e) {
throw new Service.ServiceConfigException(e);
}
logger.info("build REST server @ {}, listeners: {}", this.port, String.join(",", this.services.keySet()));
services.forEach((path, supp) ->
rest.server.createContext(path, http -> {
Consumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy