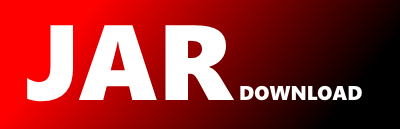
io.github.leheyue.magicapi.nebula.response.NebulaJsonBody Maven / Gradle / Ivy
package io.github.leheyue.magicapi.nebula.response;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.HashMap;
import java.util.List;
public class NebulaJsonBody {
private List errors;
private List results;
public List getErrors() {
return errors;
}
public void setErrors(List errors) {
this.errors = errors;
}
public int getErrorCode() {
return this.errors.get(0).getCode();
}
public String getErrorMsg() {
return this.errors.get(0).getMessage();
}
public List getResults() {
return results;
}
public void setResults(List results) {
this.results = results;
}
public static class NebulaError {
private int code;
private String message;
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
public static class Result {
@JsonProperty("spaceName")
private String spaceName;
private List data;
private List columns;
private NebulaError errors;
@JsonProperty("latencyInUs")
private long latencyInUs;
public String getSpaceName() {
return spaceName;
}
public void setSpaceName(String spaceName) {
this.spaceName = spaceName;
}
public List getData() {
return data;
}
public void setData(List data) {
this.data = data;
}
public List getColumns() {
return columns;
}
public void setColumns(List columns) {
this.columns = columns;
}
public NebulaError getErrors() {
return errors;
}
public void setErrors(NebulaError errors) {
this.errors = errors;
}
public long getLatencyInUs() {
return latencyInUs;
}
public void setLatencyInUs(long latencyInUs) {
this.latencyInUs = latencyInUs;
}
}
public static class Data {
private List> meta;
private List>> row;
public List> getMeta() {
return meta;
}
public void setMeta(List> meta) {
this.meta = meta;
}
public List>> getRow() {
return row;
}
public void setRow(List>> row) {
this.row = row;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy