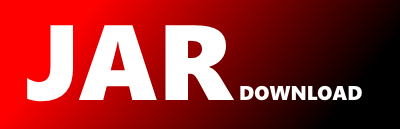
com.xiaopy.python.PyIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chaquopy_java Show documentation
Show all versions of chaquopy_java Show documentation
The Python SDK for Android
package com.xiaopy.python;
import java.util.*;
abstract class PyIterator implements Iterator {
private PyObject iter;
private boolean hasNextElem = true;
private PyObject nextElem;
public PyIterator(MethodCache methods) {
iter = methods.get("__iter__").call();
updateNext();
}
protected void updateNext() {
try {
nextElem = iter.callAttr("__next__");
} catch (PyException e) {
if (e.getMessage().startsWith("StopIteration:")) {
hasNextElem = false;
nextElem = null;
} else {
throw e;
}
}
}
@Override public boolean hasNext() {
return hasNextElem;
}
@Override public T next() {
if (!hasNext()) throw new NoSuchElementException();
T result = makeNext(nextElem);
updateNext();
return result;
}
protected abstract T makeNext(PyObject element);
@Override public void remove() {
throw new UnsupportedOperationException(
"Python does not support removing from a container while iterating over it");
// The removal would succeed, but the iterator would refuse to continue.
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy