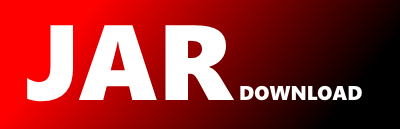
com.orion.office.csv.reader.CsvLambdaReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orion-office Show documentation
Show all versions of orion-office Show documentation
orion office (excel csv and more...)
package com.orion.office.csv.reader;
import com.orion.lang.define.wrapper.Pair;
import com.orion.lang.utils.Valid;
import com.orion.office.csv.core.CsvReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Map;
import java.util.TreeMap;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* csv lambda 读取器
*
* @author Jiahang Li
* @version 1.0.0
* @since 2021/9/8 18:47
*/
public class CsvLambdaReader extends BaseCsvReader {
/**
* 映射
* key: column
* value: convert consumer
*/
protected final Map, BiConsumer>> mapping;
/**
* supplier
*/
protected final Supplier supplier;
/**
* 为null是否调用
*/
protected boolean nullInvoke;
public CsvLambdaReader(CsvReader reader, Supplier supplier) {
this(reader, new ArrayList<>(), null, supplier);
}
public CsvLambdaReader(CsvReader reader, Collection rows, Supplier supplier) {
this(reader, rows, null, supplier);
}
public CsvLambdaReader(CsvReader reader, Consumer consumer, Supplier supplier) {
this(reader, null, consumer, supplier);
}
protected CsvLambdaReader(CsvReader reader, Collection rows, Consumer consumer, Supplier supplier) {
super(reader, rows, consumer);
this.supplier = Valid.notNull(supplier, "supplier is null");
this.mapping = new TreeMap<>();
}
/**
* 如果列为null是否调用function consumer
*
* @return this
*/
public CsvLambdaReader nullInvoke() {
this.nullInvoke = true;
return this;
}
public CsvLambdaReader mapping(int column, BiConsumer consumer) {
mapping.put(column, Pair.of(Function.identity(), consumer));
return this;
}
/**
* 映射
*
* @param column column
* @param convert 转换器
* @param consumer 消费者
* @return this
*/
public CsvLambdaReader mapping(int column, Function convert, BiConsumer consumer) {
mapping.put(column, Pair.of(convert, consumer));
return this;
}
@Override
@SuppressWarnings("unchecked")
protected T parserRow(String[] row) {
T current = supplier.get();
mapping.forEach((k, v) -> {
String value = this.get(row, k);
if (value != null || nullInvoke) {
Function convert = v.getKey();
BiConsumer consumer = (BiConsumer) v.getValue();
Object val = convert.apply(value);
consumer.accept(current, val);
}
});
return current;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy