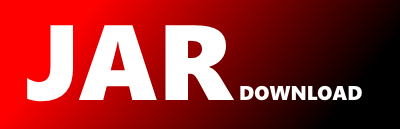
com.orion.office.csv.reader.CsvReaderIterator Maven / Gradle / Ivy
Show all versions of orion-office Show documentation
package com.orion.office.csv.reader;
import com.orion.lang.able.SafeCloseable;
import com.orion.lang.utils.Exceptions;
import java.util.Iterator;
/**
* csv 迭代器
*
* 不会存储也不会消费
*
* @author Jiahang Li
* @version 1.0.0
* @since 2021/2/2 18:31
*/
public class CsvReaderIterator implements SafeCloseable, Iterator, Iterable {
private final BaseCsvReader reader;
/**
* 是否为第一次
*/
private boolean first;
/**
* 是否还有下一个元素
*/
private boolean hasNext;
/**
* 下一个元素
*/
private T next;
public CsvReaderIterator(BaseCsvReader reader) {
this.reader = reader;
this.first = true;
}
@Override
public Iterator iterator() {
return this;
}
@Override
public boolean hasNext() {
if (first) {
this.first = false;
this.hasNext = this.getNext();
}
return hasNext;
}
@Override
public T next() {
if (first) {
boolean next = this.getNext();
if (!next) {
this.throwNoSuch();
}
}
if (!hasNext && !first) {
this.throwNoSuch();
}
if (first) {
this.first = false;
}
T next = this.next;
this.hasNext = this.getNext();
reader.rowNum++;
return next;
}
/**
* 获取下一个元素
*
* @return element
*/
private boolean getNext() {
if (reader.end) {
return false;
}
this.next = reader.nextRow();
return !reader.end;
}
/**
* 抛出异常
*/
private void throwNoSuch() {
throw Exceptions.noSuchElement("there are no more elements");
}
@Override
public void close() {
reader.close();
}
}