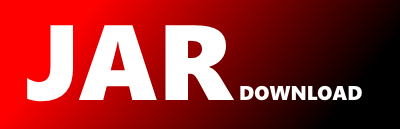
com.orion.office.excel.ExcelExt Maven / Gradle / Ivy
Show all versions of orion-office Show documentation
package com.orion.office.excel;
import com.monitorjbl.xlsx.impl.StreamingWorkbook;
import com.orion.lang.able.SafeCloseable;
import com.orion.lang.define.collect.MutableMap;
import com.orion.lang.utils.Valid;
import com.orion.lang.utils.io.Files1;
import com.orion.lang.utils.io.Streams;
import com.orion.office.excel.reader.ExcelArrayReader;
import com.orion.office.excel.reader.ExcelBeanReader;
import com.orion.office.excel.reader.ExcelLambdaReader;
import com.orion.office.excel.reader.ExcelMapReader;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import java.io.File;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Supplier;
/**
* excel 提取器
*
* 使用流式读取文件必须是 xlsx 文件
*
* @author Jiahang Li
* @version 1.0.0
* @since 2020/4/6 12:51
*/
public class ExcelExt implements SafeCloseable {
private final Workbook workbook;
public ExcelExt(File file) {
this(Files1.openInputStreamSafe(file), null, false, true);
}
public ExcelExt(File file, boolean streaming) {
this(Files1.openInputStreamSafe(file), null, streaming, true);
}
public ExcelExt(File file, String password) {
this(Files1.openInputStreamSafe(file), password, false, true);
}
public ExcelExt(File file, String password, boolean streaming) {
this(Files1.openInputStreamSafe(file), password, streaming, true);
}
public ExcelExt(String file) {
this(Files1.openInputStreamSafe(file), null, false, true);
}
public ExcelExt(String file, boolean streaming) {
this(Files1.openInputStreamSafe(file), null, streaming, true);
}
public ExcelExt(String file, String password) {
this(Files1.openInputStreamSafe(file), password, false, true);
}
public ExcelExt(String file, String password, boolean streaming) {
this(Files1.openInputStreamSafe(file), password, streaming, true);
}
public ExcelExt(InputStream in) {
this(in, null, false, false);
}
public ExcelExt(InputStream in, boolean streaming) {
this(in, null, streaming, false);
}
public ExcelExt(InputStream in, String password) {
this(in, password, false, false);
}
public ExcelExt(InputStream in, String password, boolean streaming) {
this(in, password, streaming, false);
}
public ExcelExt(InputStream in, String password, boolean streaming, boolean close) {
if (streaming) {
// 使用流式读取文件必须是 xlsx 文件
this.workbook = Excels.openStreamingWorkbook(in, password);
} else {
this.workbook = Excels.openWorkbook(in, password);
}
Valid.notNull(workbook, "workbook is null");
if (close) {
Streams.close(in);
}
}
public ExcelExt(Workbook workbook) {
Valid.notNull(workbook, "workbook is null");
this.workbook = workbook;
}
// -------------------- array reader --------------------
public ExcelArrayReader arrayReader(int sheetIndex) {
return this.arrayReader(workbook.getSheetAt(sheetIndex), new ArrayList<>(), null);
}
public ExcelArrayReader arrayReader(String sheetName) {
return this.arrayReader(workbook.getSheet(sheetName), new ArrayList<>(), null);
}
public ExcelArrayReader arrayReader(int sheetIndex, List rows) {
return this.arrayReader(workbook.getSheetAt(sheetIndex), rows, null);
}
public ExcelArrayReader arrayReader(String sheetName, List rows) {
return this.arrayReader(workbook.getSheet(sheetName), rows, null);
}
public ExcelArrayReader arrayReader(int sheetIndex, Consumer consumer) {
return this.arrayReader(workbook.getSheetAt(sheetIndex), null, consumer);
}
public ExcelArrayReader arrayReader(String sheetName, Consumer consumer) {
return this.arrayReader(workbook.getSheet(sheetName), null, consumer);
}
/**
* 获取表格的 array 读取器
*
* @param sheet sheet
* @param rows rows
* @param consumer consumer
* @return ExcelArrayReader
*/
private ExcelArrayReader arrayReader(Sheet sheet, List rows, Consumer consumer) {
if (rows != null) {
return new ExcelArrayReader(workbook, sheet, rows);
} else {
return new ExcelArrayReader(workbook, sheet, consumer);
}
}
// -------------------- map reader --------------------
public ExcelMapReader mapReader(int sheetIndex) {
return this.mapReader(workbook.getSheetAt(sheetIndex), new ArrayList<>(), null);
}
public ExcelMapReader mapReader(String sheetName) {
return this.mapReader(workbook.getSheet(sheetName), new ArrayList<>(), null);
}
public ExcelMapReader mapReader(int sheetIndex, List> rows) {
return this.mapReader(workbook.getSheetAt(sheetIndex), rows, null);
}
public ExcelMapReader mapReader(String sheetName, List> rows) {
return this.mapReader(workbook.getSheet(sheetName), rows, null);
}
public ExcelMapReader mapReader(int sheetIndex, Consumer> consumer) {
return this.mapReader(workbook.getSheetAt(sheetIndex), null, consumer);
}
public ExcelMapReader mapReader(String sheetName, Consumer> consumer) {
return this.mapReader(workbook.getSheet(sheetName), null, consumer);
}
/**
* 获取表格的 map 读取器
*
* @param sheet sheet
* @param rows rows
* @param consumer consumer
* @param K
* @param V
* @return ExcelMapReader
*/
private ExcelMapReader mapReader(Sheet sheet, List> rows, Consumer> consumer) {
if (rows != null) {
return new ExcelMapReader<>(workbook, sheet, rows);
} else {
return new ExcelMapReader<>(workbook, sheet, consumer);
}
}
// -------------------- bean reader --------------------
public ExcelBeanReader beanReader(int sheetIndex, Class targetClass) {
return this.beanReader(workbook.getSheetAt(sheetIndex), targetClass, new ArrayList<>(), null);
}
public ExcelBeanReader beanReader(String sheetName, Class targetClass) {
return this.beanReader(workbook.getSheet(sheetName), targetClass, new ArrayList<>(), null);
}
public ExcelBeanReader beanReader(int sheetIndex, Class targetClass, List rows) {
return this.beanReader(workbook.getSheetAt(sheetIndex), targetClass, rows, null);
}
public ExcelBeanReader beanReader(String sheetName, Class targetClass, List rows) {
return this.beanReader(workbook.getSheet(sheetName), targetClass, rows, null);
}
public ExcelBeanReader beanReader(int sheetIndex, Class targetClass, Consumer consumer) {
return this.beanReader(workbook.getSheetAt(sheetIndex), targetClass, null, consumer);
}
public ExcelBeanReader beanReader(String sheetName, Class targetClass, Consumer consumer) {
return this.beanReader(workbook.getSheet(sheetName), targetClass, null, consumer);
}
/**
* 获取表格的 bean 读取器
*
* @param sheet sheet
* @param targetClass bean class
* @param rows rows
* @param consumer consumer
* @param T
* @return ExcelBeanReader
*/
private ExcelBeanReader beanReader(Sheet sheet, Class targetClass, List rows, Consumer consumer) {
if (rows != null) {
return new ExcelBeanReader<>(workbook, sheet, targetClass, rows);
} else {
return new ExcelBeanReader<>(workbook, sheet, targetClass, consumer);
}
}
// -------------------- lambda reader --------------------
public ExcelLambdaReader lambdaReader(int sheetIndex, Supplier supplier) {
return this.lambdaReader(workbook.getSheetAt(sheetIndex), new ArrayList<>(), null, supplier);
}
public ExcelLambdaReader lambdaReader(String sheetName, Supplier supplier) {
return this.lambdaReader(workbook.getSheet(sheetName), new ArrayList<>(), null, supplier);
}
public ExcelLambdaReader lambdaReader(int sheetIndex, List rows, Supplier supplier) {
return this.lambdaReader(workbook.getSheetAt(sheetIndex), rows, null, supplier);
}
public ExcelLambdaReader lambdaReader(String sheetName, List rows, Supplier supplier) {
return this.lambdaReader(workbook.getSheet(sheetName), rows, null, supplier);
}
public ExcelLambdaReader lambdaReader(int sheetIndex, Consumer consumer, Supplier supplier) {
return this.lambdaReader(workbook.getSheetAt(sheetIndex), null, consumer, supplier);
}
public ExcelLambdaReader lambdaReader(String sheetName, Consumer consumer, Supplier supplier) {
return this.lambdaReader(workbook.getSheet(sheetName), null, consumer, supplier);
}
/**
* 获取表格的 lambda 读取器
*
* @param sheet sheet
* @param rows rows
* @param consumer consumer
* @param supplier supplier
* @param T
* @return ExcelLambdaReader
*/
private ExcelLambdaReader lambdaReader(Sheet sheet, List rows, Consumer consumer, Supplier supplier) {
if (rows != null) {
return new ExcelLambdaReader<>(workbook, sheet, rows, supplier);
} else {
return new ExcelLambdaReader<>(workbook, sheet, consumer, supplier);
}
}
public Workbook getWorkbook() {
return workbook;
}
public boolean isStreaming() {
return workbook instanceof StreamingWorkbook;
}
@Override
public void close() {
Streams.close(workbook);
}
}