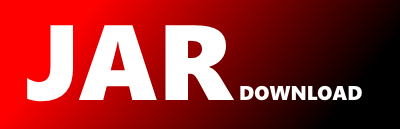
com.orion.office.excel.writer.ExcelWriterBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orion-office Show documentation
Show all versions of orion-office Show documentation
orion office (excel csv and more...)
package com.orion.office.excel.writer;
import com.orion.office.excel.Excels;
import com.orion.office.excel.option.PropertiesOption;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
/**
* excel 构建器
*
* @author Jiahang Li
* @version 1.0.0
* @since 2020/4/6 21:59
*/
public class ExcelWriterBuilder extends BaseExcelWriteable {
public ExcelWriterBuilder() {
this(new SXSSFWorkbook());
}
public ExcelWriterBuilder(Workbook workbook) {
super(workbook);
}
public ExcelArrayWriter getArrayWriter(String name) {
return new ExcelArrayWriter<>(workbook, workbook.getSheet(name));
}
/**
* 获取 array writer
*
* @param T
* @param index index
* @return ExcelArrayWriter
*/
public ExcelArrayWriter getArrayWriter(int index) {
return new ExcelArrayWriter<>(workbook, workbook.getSheetAt(index));
}
public ExcelMapWriter getMapWriter(String name) {
return new ExcelMapWriter<>(workbook, workbook.getSheet(name));
}
/**
* 获取 map writer
*
* @param K
* @param V
* @param index index
* @return ExcelMapWriter
*/
public ExcelMapWriter getMapWriter(int index) {
return new ExcelMapWriter<>(workbook, workbook.getSheetAt(index));
}
public ExcelBeanWriter getBeanWriter(String name, Class targetClass) {
return new ExcelBeanWriter<>(workbook, workbook.getSheet(name), targetClass);
}
/**
* 获取 bean writer
*
* @param T
* @param index index
* @param targetClass targetClass
* @return ExcelBeanWriter
*/
public ExcelBeanWriter getBeanWriter(int index, Class targetClass) {
return new ExcelBeanWriter<>(workbook, workbook.getSheetAt(index), targetClass);
}
public ExcelLambdaWriter getLambdaWriter(String name) {
return new ExcelLambdaWriter<>(workbook, workbook.getSheet(name));
}
/**
* 获取 lambda writer
*
* @param T
* @param index index
* @return ExcelLambdaWriter
*/
public ExcelLambdaWriter getLambdaWriter(int index) {
return new ExcelLambdaWriter<>(workbook, workbook.getSheetAt(index));
}
public ExcelArrayWriter createArrayWriter() {
return new ExcelArrayWriter<>(workbook, workbook.createSheet());
}
/**
* 创建 array writer
*
* @param T
* @param name name
* @return ExcelArrayWriter
*/
public ExcelArrayWriter createArrayWriter(String name) {
return new ExcelArrayWriter<>(workbook, workbook.createSheet(name));
}
public ExcelMapWriter createMapWriter() {
return new ExcelMapWriter<>(workbook, workbook.createSheet());
}
/**
* 创建 map writer
*
* @param K
* @param V
* @param name name
* @return ExcelMapWriter
*/
public ExcelMapWriter createMapWriter(String name) {
return new ExcelMapWriter<>(workbook, workbook.createSheet(name));
}
public ExcelBeanWriter createBeanWriter(Class targetClass) {
return new ExcelBeanWriter<>(workbook, workbook.createSheet(), targetClass);
}
/**
* 创建 bean writer
*
* @param T
* @param name name
* @param targetClass targetClass
* @return ExcelBeanWriter
*/
public ExcelBeanWriter createBeanWriter(String name, Class targetClass) {
return new ExcelBeanWriter<>(workbook, workbook.createSheet(name), targetClass);
}
public ExcelLambdaWriter createLambdaWriter() {
return new ExcelLambdaWriter<>(workbook, workbook.createSheet());
}
/**
* 创建 lambda writer
*
* @param T
* @param name name
* @return ExcelLambdaWriter
*/
public ExcelLambdaWriter createLambdaWriter(String name) {
return new ExcelLambdaWriter<>(workbook, workbook.createSheet(name));
}
/**
* 设置表格属性
*
* @param option option
* @return this
*/
public ExcelWriterBuilder properties(PropertiesOption option) {
Excels.setProperties(workbook, option);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy