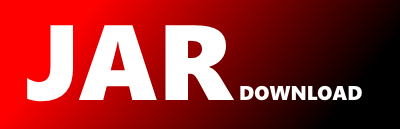
io.github.linmoure.utils.DateUtil Maven / Gradle / Ivy
package io.github.linmoure.utils;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import io.github.linmoure.constants.Constants;
import io.github.linmoure.constants.enumConstants.UrlEnum;
import lombok.extern.slf4j.Slf4j;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.util.Calendar;
import java.util.Date;
import java.util.Map;
import java.util.Objects;
@Slf4j
public class DateUtil {
/**
* 一天 86400L 秒
*/
public static final Long DAY_SECONDS = 86400L;
public static final DateTimeFormatter DFY_MD_HMS = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
public static final DateTimeFormatter DFY_MD = DateTimeFormatter.ofPattern("yyyy-MM-dd");
/**
* LocalDateTime 转时间戳
*
* @param localDateTime /
* @return /
*/
public static Long getTimeStamp(LocalDateTime localDateTime) {
return localDateTime.atZone(ZoneId.systemDefault()).toEpochSecond();
}
public static String getNow() {
return LocalDateTime.now().format(DFY_MD_HMS);
}
public static String getNow(DateTimeFormatter dtf) {
return LocalDateTime.now().format(dtf);
}
public static String getYesterday() {
return LocalDateTime.now().plusDays(-Constants.INTEGER_1).format(DFY_MD_HMS);
}
public static String getYesterday(DateTimeFormatter dtf) {
return LocalDateTime.now().plusDays(-Constants.INTEGER_1).format(dtf == null ? DFY_MD : dtf);
}
public static String localDateTimeToTimeString(LocalDateTime localDateTime) {
return localDateTime.format(DFY_MD_HMS);
}
public static String localDateToDateString(LocalDate localDate) {
return localDate.format(DFY_MD);
}
/**
* 时间戳转LocalDateTime
*
* @param timeStamp /
* @return /
*/
public static LocalDateTime fromTimeStamp(Long timeStamp) {
return LocalDateTime.ofEpochSecond(timeStamp, 0, OffsetDateTime.now().getOffset());
}
/**
* LocalDateTime 转 Date
* Jdk8 后 不推荐使用 {@link Date} Date
*
* @param localDateTime /
* @return /
*/
public static Date toDate(LocalDateTime localDateTime) {
return Date.from(localDateTime.atZone(ZoneId.systemDefault()).toInstant());
}
/**
* LocalDate 转 Date
* Jdk8 后 不推荐使用 {@link Date} Date
*
* @param localDate /
* @return /
*/
public static Date toDate(LocalDate localDate) {
return toDate(localDate.atTime(LocalTime.now(ZoneId.systemDefault())));
}
/**
* Date转 LocalDateTime
* Jdk8 后 不推荐使用 {@link Date} Date
*
* @param date /
* @return /
*/
public static LocalDateTime toLocalDateTime(Date date) {
return LocalDateTime.ofInstant(date.toInstant(), ZoneId.systemDefault());
}
/**
* 日期 格式化
*
* @param localDateTime /
* @param patten /
* @return /
*/
public static String localDateTimeFormat(LocalDateTime localDateTime, String patten) {
DateTimeFormatter df = DateTimeFormatter.ofPattern(patten);
return df.format(localDateTime);
}
/**
* 日期 格式化
*
* @param localDateTime /
* @param df /
* @return /
*/
public static String localDateTimeFormat(LocalDateTime localDateTime, DateTimeFormatter df) {
return df.format(localDateTime);
}
/**
* 日期格式化 yyyy-MM-dd HH:mm:ss
*
* @param localDateTime /
* @return /
*/
public static String localDateTimeFormatyMdHms(LocalDateTime localDateTime) {
return DFY_MD_HMS.format(localDateTime);
}
/**
* 日期格式化 yyyy-MM-dd
*
* @param localDateTime /
* @return /
*/
public String localDateTimeFormatyMd(LocalDateTime localDateTime) {
return DFY_MD.format(localDateTime);
}
/**
* 字符串转 LocalDateTime ,字符串格式 yyyy-MM-dd
*
* @param localDateTime /
* @param pattern /
* @return /
*/
public static LocalDateTime parseLocalDateTimeFormat(String localDateTime, String pattern) {
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern(pattern);
return LocalDateTime.from(dateTimeFormatter.parse(localDateTime));
}
/**
* 字符串转 LocalDateTime ,字符串格式 yyyy-MM-dd
*
* @param localDateTime /
* @param dateTimeFormatter /
* @return /
*/
public static LocalDateTime parseLocalDateTimeFormat(String localDateTime, DateTimeFormatter dateTimeFormatter) {
return LocalDateTime.from(dateTimeFormatter.parse(localDateTime));
}
/**
* 字符串转 LocalDateTime ,字符串格式 yyyy-MM-dd HH:mm:ss
*
* @param localDateTime /
* @return /
*/
public static LocalDateTime parseLocalDateTimeFormatyMdHms(String localDateTime) {
return LocalDateTime.from(DFY_MD_HMS.parse(localDateTime));
}
/**
* 获得当天是周几
*
* @return day
*/
public static String getWeekDay() {
String[] weekDays = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
Calendar cal = Calendar.getInstance();
cal.setTime(new Date());
int w = cal.get(Calendar.DAY_OF_WEEK) - 1;
if (w < 0) {
w = 0;
}
return weekDays[w];
}
public static LocalDateTime getBeforeMonth(long x) {
return LocalDateTime.now().minusMonths(x);
}
public static boolean isHoliday() {
return isHoliday(DateUtil.getNow(DateUtil.DFY_MD));
}
public static boolean isHoliday(String date) {
try {
String result = HttpClientPool.getResult(UrlEnum.HOLIDAY_URL.get() + date);
JSONObject holiday = JSON.parseObject(result);
log.info("holiday:{}", result);
if (Objects.equals(Constants.INTEGER_0, holiday.getInteger("code"))) {
Map map = holiday.getObject("holiday", Map.class);
if (map == null || map.isEmpty()) {
return false;
} else {
log.info("今天是:{}", map.get("date") + map.get("name"));
return true;
}
} else {
return false;
}
} catch (Exception e) {
log.error(e.getMessage(), e);
return false;
}
}
public static boolean isWeekEnd() {
// 创建Calendar类实例
Calendar instance = Calendar.getInstance();
// 获取今天星期几
int today = instance.get(Calendar.DAY_OF_WEEK);
return (today == Calendar.SATURDAY || today == Calendar.SUNDAY);
}
/**
* 计算俩个时间相差几天
*
* @param after 时间戳
* @param before 时间戳
* @return 相差天数
*/
public static long getTimeDiff(Long after, Long before) {
long diff = before - after;
// 获取日
long day = diff / (1000 * 60 * 60 * 24);
diff = diff % (1000 * 60 * 60 * 24);
// 获取时
long hour = diff / (1000 * 60 * 60);
diff = diff % (1000 * 60 * 60);
// 获取分
long min = diff / (1000 * 60);
diff = diff % (1000 * 60);
// 获取秒
long sec = diff / 1000;
if (hour > 0 || min > 0 || sec > 0) {
day = day + 1;
}
return day;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy