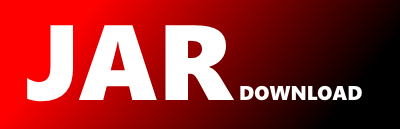
io.github.linmoure.utils.EncryptUtils Maven / Gradle / Ivy
package io.github.linmoure.utils;
import com.alibaba.fastjson.JSON;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.lang3.StringUtils;
import java.nio.charset.StandardCharsets;
import java.util.Arrays;
import java.util.Map;
@Slf4j
public class EncryptUtils extends CodingUtil {
public static final String SECRET_KEY = "1d136603b8134384b2fd31a04fabf0e9";
public static final String IV = "9581491399778464";
public static final String CLIENT_CODE = "client_code";
public static final String CLIENT_SECRET = "client_secret";
public static final String REQ_TIME = "req_time";
public static final String AUTH_CODE = "auth_code";
/**
* AES 加密
*
* @param str 加密字符串
* @return 解密结果
*/
public static Object aesEncrypt(String str) {
return aesEncrypt(str, SECRET_KEY, IV);
}
/**
* AES 解密
*
* @param str 需要解密的字符串
* @return 解密结果
*/
public static Object aesDecrypt(String str) {
return aesDecrypt(str, SECRET_KEY, IV);
}
/**
* 生成参数排序
*
* @param params 参数Map
* @param clientCode clientCode
* @param clientSecret clientSecret
* @return auth_code
*/
public static String getSign(Map params, String clientCode, String clientSecret) {
params.put(CLIENT_CODE, clientCode);
params.put(CLIENT_SECRET, clientSecret);
params.put(REQ_TIME, System.currentTimeMillis() / 1000);
String[] array = params.keySet().toArray(new String[0]);
Arrays.sort(array, String.CASE_INSENSITIVE_ORDER);
StringBuilder builder = new StringBuilder();
for (String arr : array) {
builder.append(params.get(arr));
}
params.remove(CLIENT_SECRET);
String code = DigestUtils.md5Hex(builder.toString().getBytes(StandardCharsets.UTF_8));
log.info("参数排序:::{},auth_code:::{}", builder, code);
return code;
}
/**
* 校验¬参数排序
*
* @param params params
* @param authCode authCode
* @param clientSecret clientSecret
*/
public static void checkSign(Map params, String authCode, String clientSecret) {
log.info("传入参数:::{}", JSON.toJSONString(params));
if (StringUtils.isEmpty(authCode)) {
throw new RuntimeException("鉴权失败");
}
if (params.get(REQ_TIME) == null || (System.currentTimeMillis() / 1000 - ((Long) params.get(REQ_TIME))) > 5) {
throw new RuntimeException("请求超时");
}
if (params.keySet().contains(AUTH_CODE) || params.keySet().contains(CLIENT_SECRET)) {
throw new RuntimeException("鉴权失败");
}
params.put(CLIENT_SECRET, clientSecret);
String[] array = params.keySet().toArray(new String[0]);
Arrays.sort(array, String.CASE_INSENSITIVE_ORDER);
StringBuilder builder = new StringBuilder();
for (String arr : array) {
builder.append(params.get(arr));
}
log.info("参数排序:::{}", builder);
if (!DigestUtils.md5Hex(builder.toString()).equals(authCode)) {
throw new RuntimeException("鉴权失败");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy