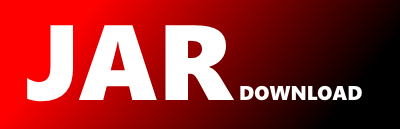
com.ebay.sdk.call.ReviseMyMessagesCall Maven / Gradle / Ivy
/*
Copyright (c) 2013 eBay, Inc.
This program is licensed under the terms of the eBay Common Development and
Distribution License (CDDL) Version 1.0 (the "License") and any subsequent version
thereof released by eBay. The then-current version of the License can be found
at http://www.opensource.org/licenses/cddl1.php and in the eBaySDKLicense file that
is under the eBay SDK ../docs directory.
*/
package com.ebay.sdk.call;
import java.lang.Boolean;
import java.lang.Long;
import com.ebay.sdk.*;
import com.ebay.soap.eBLBaseComponents.*;
/**
* Wrapper class of the ReviseMyMessages call of eBay SOAP API.
*
* Title: SOAP API wrapper library.
* Description: Contains wrapper classes for eBay SOAP APIs.
* Copyright: Copyright (c) 2009
* Company: eBay Inc.
*
Input property: MessageIDs
- This container is used to specify up to 10 messages (specified with their MessageID values) on which to perform on or more actions. At least one MessageID value must be included in the request. MessageID values can be retrieved with the GetMyMessages call with the DetailLevel value set to ReturnHeaders
.
*
*
* Note: Messages in the Sent folder of My Messages cannot be moved, marked as read, or flagged.
*
Input property: AlertIDs
- This field is deprecated.
*
Input property: Read
- This boolean field is used to change the 'Read' status of the message(s) in the MessageIDs container. Including this field and setting its value to true
will mark all messages in the MessageIDs container as 'Read'. Conversely, including this field and setting its value to false
will mark all messages in the MessageIDs container as 'Unread'. The 'Read' status of a message can be retrieved by looking at the Message.Read boolean field of the GetMyMessages call response.
*
*
* In each ReviseMyMessages call, at least one of the following fields must be specified in the request: Read, Flagged, and FolderID.
*
*
* Note: Messages in the Sent folder of My Messages cannot be moved, marked as read, or flagged.
*
Input property: Flagged
- This boolean field is used to change the 'Flagged' status of the message(s) in the MessageIDs container. Including this field and setting its value to true
will flag all messages in the MessageIDs container. Conversely, including this field and setting its value to false
will unflag all messages in the MessageIDs container. The 'Flagged' status of a message can be retrieved by looking at the Message.Flagged boolean field of the GetMyMessages call response.
*
*
* In each ReviseMyMessages call, at least one of the following fields must be specified in the request: Read, Flagged, and FolderID.
*
*
* Note: Messages in the Sent folder of My Messages cannot be moved, marked as read, or flagged.
*
Input property: FolderID
- A unique identifier of My Messages folder. A FolderID value is supplied if the user want to move the message(s) in the MessageIDs container to a different folder. FolderID values can be retrieved with the GetMyMessages call with the DetailLevel value set to ReturnSummary
.
*
* In each ReviseMyMessages call, at least one of the following fields must be specified in the request: Read, Flagged, and FolderID.
*
*
* Note: Messages in the Sent folder of My Messages cannot be moved, marked as read, or flagged.
*
* @author Ron Murphy
* @version 1.0
*/
public class ReviseMyMessagesCall extends com.ebay.sdk.ApiCall
{
private String[] messageIDs = null;
private String[] alertIDs = null;
private Boolean read = null;
private Boolean flagged = null;
private Long folderID = null;
/**
* Constructor.
*/
public ReviseMyMessagesCall() {
}
/**
* Constructor.
* @param apiContext The ApiContext object to be used to make the call.
*/
public ReviseMyMessagesCall(ApiContext apiContext) {
super(apiContext);
}
/**
* This call can be used to mark one or more messages as 'Read', to flag one or more messages, and/or to move one or more messages to another My Messages folder. Any of these actions can be applied on up to 10 messages with one call.
*
*
* @throws ApiException
* @throws SdkException
* @throws Exception
* @return The void object.
*/
public void reviseMyMessages()
throws com.ebay.sdk.ApiException, com.ebay.sdk.SdkException, java.lang.Exception
{
ReviseMyMessagesRequestType req;
req = new ReviseMyMessagesRequestType();
if (this.messageIDs != null)
{
MyMessagesMessageIDArrayType ary = new MyMessagesMessageIDArrayType();
ary.setMessageID(this.messageIDs);
req.setMessageIDs(ary);
}
if (this.alertIDs != null)
{
MyMessagesAlertIDArrayType ary = new MyMessagesAlertIDArrayType();
ary.setAlertID(this.alertIDs);
req.setAlertIDs(ary);
}
if (this.read != null)
req.setRead(this.read);
if (this.flagged != null)
req.setFlagged(this.flagged);
if (this.folderID != null)
req.setFolderID(this.folderID);
ReviseMyMessagesResponseType resp = (ReviseMyMessagesResponseType) execute(req);
}
/**
* Gets the ReviseMyMessagesRequestType.alertIDs.
* @return String[]
*/
public String[] getAlertIDs()
{
return this.alertIDs;
}
/**
* Sets the ReviseMyMessagesRequestType.alertIDs.
* @param alertIDs String[]
*/
public void setAlertIDs(String[] alertIDs)
{
this.alertIDs = alertIDs;
}
/**
* Gets the ReviseMyMessagesRequestType.flagged.
* @return Boolean
*/
public Boolean getFlagged()
{
return this.flagged;
}
/**
* Sets the ReviseMyMessagesRequestType.flagged.
* @param flagged Boolean
*/
public void setFlagged(Boolean flagged)
{
this.flagged = flagged;
}
/**
* Gets the ReviseMyMessagesRequestType.folderID.
* @return Long
*/
public Long getFolderID()
{
return this.folderID;
}
/**
* Sets the ReviseMyMessagesRequestType.folderID.
* @param folderID Long
*/
public void setFolderID(Long folderID)
{
this.folderID = folderID;
}
/**
* Gets the ReviseMyMessagesRequestType.messageIDs.
* @return String[]
*/
public String[] getMessageIDs()
{
return this.messageIDs;
}
/**
* Sets the ReviseMyMessagesRequestType.messageIDs.
* @param messageIDs String[]
*/
public void setMessageIDs(String[] messageIDs)
{
this.messageIDs = messageIDs;
}
/**
* Gets the ReviseMyMessagesRequestType.read.
* @return Boolean
*/
public Boolean getRead()
{
return this.read;
}
/**
* Sets the ReviseMyMessagesRequestType.read.
* @param read Boolean
*/
public void setRead(Boolean read)
{
this.read = read;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy