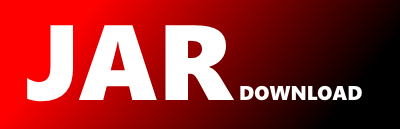
io.github.linuxforhealth.hl7.expression.SimpleExpression Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hl7v2-fhir-converter Show documentation
Show all versions of hl7v2-fhir-converter Show documentation
FHIR converter is a Java based library that enables converting Hl7v2 messages to FHIR resources
/*
* (C) Copyright IBM Corp. 2020
*
* SPDX-License-Identifier: Apache-2.0
*/
package io.github.linuxforhealth.hl7.expression;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
import io.github.linuxforhealth.api.EvaluationResult;
import io.github.linuxforhealth.api.InputDataExtractor;
import io.github.linuxforhealth.core.Constants;
import io.github.linuxforhealth.core.expression.EvaluationResultFactory;
import io.github.linuxforhealth.core.expression.VariableUtils;
import io.github.linuxforhealth.hl7.data.SimpleDataTypeMapper;
import io.github.linuxforhealth.hl7.data.ValueExtractor;
import io.github.linuxforhealth.hl7.resource.deserializer.TemplateFieldNames;
@JsonIgnoreProperties(ignoreUnknown = true)
public class SimpleExpression extends AbstractExpression {
private static final Logger LOGGER = LoggerFactory.getLogger(SimpleExpression.class);
private String value;
@JsonCreator
public SimpleExpression(String var) {
this("String", var, new HashMap<>(), null, false);
}
/**
*
* @param type
* @param value
* @param variables
* @param condition
*/
@JsonCreator
public SimpleExpression(@JsonProperty(TemplateFieldNames.TYPE) String type,
@JsonProperty(TemplateFieldNames.VALUE) String value,
@JsonProperty(TemplateFieldNames.VARIABLES) Map variables,
@JsonProperty(TemplateFieldNames.CONDITION) String condition,
@JsonProperty(TemplateFieldNames.USE_GROUP) boolean useGroup) {
super(type, null, false, "", variables, condition, null, useGroup);
this.value = value;
}
public String getValue() {
return value;
}
@Override
public EvaluationResult evaluateExpression(InputDataExtractor dataSource,
Map contextValues, EvaluationResult baseValue) {
Preconditions.checkArgument(contextValues != null, "contextValues cannot be null");
Map localContextValues = new HashMap<>(contextValues);
if (baseValue != null && !baseValue.isEmpty()) {
localContextValues.put(baseValue.getIdentifier(), baseValue);
localContextValues.put(Constants.BASE_VALUE_NAME, baseValue);
}
if (VariableUtils.isVar(value)) {
EvaluationResult obj =
getVariableValueFromVariableContextMap(value, ImmutableMap.copyOf(localContextValues));
LOGGER.debug("Evaluated value {} to {} ", this.value, obj);
if (obj != null) {
return getValueOfSpecifiedType(obj.getValue());
} else {
LOGGER.debug("Evaluated {} returning null", this.value);
return null;
}
} else {
LOGGER.debug("Evaluated {} returning value enclosed as GenericResult.", this.value);
return getValueOfSpecifiedType(this.value);
}
}
private EvaluationResult getValueOfSpecifiedType(Object obj) {
if (obj != null) {
LOGGER.debug("Evaluated value {} to {} type {} ", this.value, obj, obj.getClass());
ValueExtractor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy