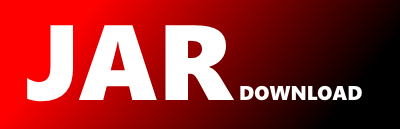
com.liubs.shadowrpcfly.server.handler.MessageHandler Maven / Gradle / Ivy
package com.liubs.shadowrpcfly.server.handler;
import com.liubs.shadowrpcfly.logging.Logger;
import com.liubs.shadowrpcfly.server.module.ModulePool;
import com.liubs.shadowrpcfly.server.module.SerializeModule;
import com.liubs.shadowrpcfly.protocol.HeartBeatMessage;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ByteToMessageCodec;
import java.util.List;
/**
* 消息的压缩和解压缩
* @author Liubsyy
* @date 2023/12/15 10:01 PM
**/
public class MessageHandler extends ByteToMessageCodec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy