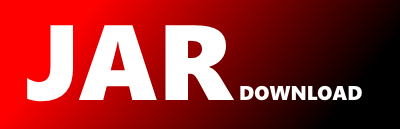
org.lognet.springboot.grpc.validation.ValidatingInterceptor Maven / Gradle / Ivy
// Generated by delombok at Wed Sep 27 05:27:18 UTC 2023
package org.lognet.springboot.grpc.validation;
import io.grpc.ForwardingServerCall;
import io.grpc.Metadata;
import io.grpc.ServerCall;
import io.grpc.ServerCallHandler;
import io.grpc.ServerInterceptor;
import io.grpc.Status;
import jakarta.validation.ConstraintViolation;
import jakarta.validation.ConstraintViolationException;
import jakarta.validation.Validator;
import java.util.Optional;
import java.util.Set;
import org.lognet.springboot.grpc.FailureHandlingSupport;
import org.lognet.springboot.grpc.MessageBlockingServerCallListener;
import org.lognet.springboot.grpc.recovery.GRpcRuntimeExceptionWrapper;
import org.lognet.springboot.grpc.validation.group.RequestMessage;
import org.lognet.springboot.grpc.validation.group.ResponseMessage;
import org.springframework.core.Ordered;
public class ValidatingInterceptor implements ServerInterceptor, Ordered {
private final Validator validator;
private Integer order;
private final FailureHandlingSupport failureHandlingSupport;
public ValidatingInterceptor(Validator validator, FailureHandlingSupport failureHandlingSupport) {
this.validator = validator;
this.failureHandlingSupport = failureHandlingSupport;
}
@Override
public ServerCall.Listener interceptCall(
ServerCall call, Metadata headers, ServerCallHandler next) {
final ForwardingServerCall.SimpleForwardingServerCall validationServerCall =
new ForwardingServerCall.SimpleForwardingServerCall(call) {
@Override
public void sendMessage(RespT message) {
final Set> violations =
validator.validate(message, ResponseMessage.class);
if (!violations.isEmpty()) {
GRpcRuntimeExceptionWrapper exception =
new GRpcRuntimeExceptionWrapper(
new ConstraintViolationException(violations), Status.FAILED_PRECONDITION);
failureHandlingSupport.closeCall(exception, this, headers, b -> b.response(message));
} else {
super.sendMessage(message);
}
}
};
ServerCall.Listener listener = next.startCall(validationServerCall, headers);
return new MessageBlockingServerCallListener(listener) {
@Override
public void onMessage(ReqT message) {
final Set> violations =
validator.validate(message, RequestMessage.class);
if (!violations.isEmpty()) {
blockMessage();
final GRpcRuntimeExceptionWrapper exception =
new GRpcRuntimeExceptionWrapper(
new ConstraintViolationException(violations), Status.INVALID_ARGUMENT);
failureHandlingSupport.closeCall(
exception, validationServerCall, headers, b -> b.request(message));
} else {
super.onMessage(message);
}
}
};
}
@Override
public int getOrder() {
return Optional.ofNullable(order).orElse(HIGHEST_PRECEDENCE + 10);
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ValidatingInterceptor order(final Integer order) {
this.order = order;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy