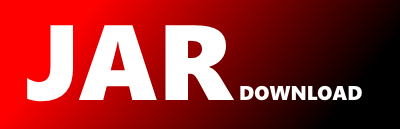
com.longport.Config Maven / Gradle / Ivy
Show all versions of openapi-sdk Show documentation
package com.longport;
import java.time.OffsetDateTime;
import java.util.concurrent.CompletableFuture;
/**
* Configuration options for LongPort sdk
*/
public class Config implements AutoCloseable {
private long raw;
/**
* @hidden
*/
Config(long config) {
this.raw = config;
}
/**
* Create a new `Config` from the given environment variables
*
* It first gets the environment variables from the .env file in the current
* directory.
*
* @return Config object
* @throws OpenApiException If an error occurs
*/
public static Config fromEnv() throws OpenApiException {
return new Config(SdkNative.newConfigFromEnv());
}
/**
* @hidden
* @return Context pointer
*/
public long getRaw() {
return this.raw;
}
@Override
public void close() throws Exception {
SdkNative.freeConfig(this.raw);
}
public CompletableFuture refreshAccessToken(OffsetDateTime expiredAt) throws OpenApiException {
return AsyncCallback.executeTask((callback) -> {
SdkNative.configRefreshAccessToken(this.raw, expiredAt, callback);
});
}
}