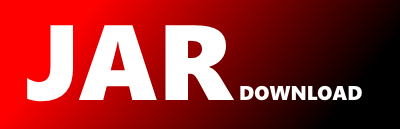
org.docx4j.openpackaging.packages.ProtectDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docx4j-core Show documentation
Show all versions of docx4j-core Show documentation
docx4j is a library which helps you to work with the Office Open
XML file format as used in docx
documents, pptx presentations, and xlsx spreadsheets.
/**
*
*/
package org.docx4j.openpackaging.packages;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import org.docx4j.openpackaging.exceptions.Docx4JException;
import org.docx4j.openpackaging.exceptions.InvalidFormatException;
import org.docx4j.openpackaging.parts.Part;
import org.docx4j.openpackaging.parts.WordprocessingML.DocumentSettingsPart;
import org.docx4j.openpackaging.parts.WordprocessingML.StyleDefinitionsPart;
import org.docx4j.org.apache.poi.poifs.crypt.HashAlgorithm;
import org.docx4j.utils.CompoundTraversalUtilVisitorCallback;
import org.docx4j.utils.TraversalUtilVisitor;
import org.docx4j.wml.ContentAccessor;
import org.docx4j.wml.P;
import org.docx4j.wml.R;
import org.docx4j.wml.STCryptProv;
import org.docx4j.wml.STDocProtect;
import org.docx4j.wml.Tbl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @author jharrop
*
*/
public class ProtectDocument extends ProtectionSettings {
protected static Logger log = LoggerFactory.getLogger(ProtectDocument.class);
public ProtectDocument(WordprocessingMLPackage pkg) {
super(pkg);
}
private WordprocessingMLPackage getPkg() {
return (WordprocessingMLPackage)pkg;
}
/**
* Restrict allowed formatting to specified styles, no password.
*
* @param allowedStyleNames
* @param removedNotAllowedFormatting whether existing usages of styles which aren't allowed are removed
* @param autoFormatOverride
* @param styleLockTheme
* @param styleLockQFSet
* @throws Docx4JException
* @since 3.3.0
*/
public void restrictFormatting(List allowedStyleNames, boolean removedNotAllowedFormatting,
boolean autoFormatOverride, boolean styleLockTheme, boolean styleLockQFSet) throws Docx4JException {
restrictFormatting(allowedStyleNames, removedNotAllowedFormatting,
autoFormatOverride, styleLockTheme, styleLockQFSet,
null, null);
}
/**
* Restrict allowed formatting to specified styles, password protected. Defaults to sha1.
*
* @param allowedStyleNames
* @param removedNotAllowedFormatting whether existing usages of styles which aren't allowed are removed
* @param autoFormatOverride
* @param styleLockTheme
* @param styleLockQFSet
* @param password
* @throws Docx4JException
* @since 3.3.0
*/
public void restrictFormatting(List allowedStyleNames, boolean removedNotAllowedFormatting,
boolean autoFormatOverride, boolean styleLockTheme, boolean styleLockQFSet,
String password) throws Docx4JException {
restrictFormatting(allowedStyleNames, removedNotAllowedFormatting,
autoFormatOverride, styleLockTheme, styleLockQFSet,
password, HashAlgorithm.sha1);
}
/**
* Restrict allowed formatting to specified styles. Specify password and HashAlgorithm
*
* @param allowedStyleNames
* @param removedNotAllowedFormatting whether existing usages of styles which aren't allowed are removed
* @param autoFormatOverride
* @param styleLockTheme
* @param styleLockQFSet
* @param password
* @param hashAlgo
* @throws Docx4JException
* @since 3.3.0
*/
public void restrictFormatting(List allowedStyleNames, boolean removedNotAllowedFormatting,
boolean autoFormatOverride, boolean styleLockTheme, boolean styleLockQFSet,
String password, HashAlgorithm hashAlgo) throws Docx4JException {
if (getPkg().getMainDocumentPart()==null)
throw new Docx4JException("No MainDocumentPart in this WordprocessingMLPackage");
if (getPkg().getMainDocumentPart().getStyleDefinitionsPart()==null)
throw new Docx4JException("No StyleDefinitionsPart in this WordprocessingMLPackage");
Set stylesInUse = getPkg().getMainDocumentPart().getStylesInUse();
// The styles part
StyleDefinitionsPart sdp = getPkg().getMainDocumentPart().getStyleDefinitionsPart();
sdp.protectRestrictFormatting(allowedStyleNames, removedNotAllowedFormatting, stylesInUse);
// TODO: do the same to stylesWithEffects.xml
// The main document part (and other content parts)
if (removedNotAllowedFormatting) {
List visitors = new ArrayList();
visitors.add(new VisitorRemovePFormatting(sdp, allowedStyleNames));
visitors.add(new VisitorRemoveRFormatting(sdp, allowedStyleNames));
visitors.add(new VisitorRemoveTableFormatting(sdp, allowedStyleNames));
CompoundTraversalUtilVisitorCallback compound = new CompoundTraversalUtilVisitorCallback(visitors);
for( Part p : getPkg().getParts().getParts().values()) {
if (p instanceof ContentAccessor) {
compound.walkJAXBElements(((ContentAccessor)p).getContent());
}
}
}
// The document settings part
DocumentSettingsPart documentSettingsPart = getPkg().getMainDocumentPart().getDocumentSettingsPart(true);
documentSettingsPart.protectRestrictFormatting(autoFormatOverride, styleLockTheme, styleLockQFSet, password, hashAlgo);
// app.xml DocSecurity
if (pkg.getDocPropsExtendedPart()==null)
{
pkg.addDocPropsExtendedPart();
}
this.setDocSecurity(0); // same as Word 2013
}
private static class VisitorRemovePFormatting extends TraversalUtilVisitor {
StyleDefinitionsPart sdp;
List allowedStyleNames;
VisitorRemovePFormatting(StyleDefinitionsPart sdp, List allowedStyleNames) {
this.sdp = sdp;
this.allowedStyleNames = allowedStyleNames;
}
@Override
public void apply(P p, Object parent, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy