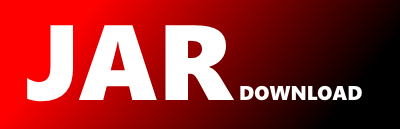
io.lsdconsulting.lsd.distributed.http.repository.InterceptedDocumentHttpRepository.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lsd-distributed-http-access Show documentation
Show all versions of lsd-distributed-http-access Show documentation
This is a HTTP version of the data access for the distributed data storage.
The newest version!
package io.lsdconsulting.lsd.distributed.http.repository
import com.fasterxml.jackson.core.type.TypeReference
import com.fasterxml.jackson.databind.ObjectMapper
import io.lsdconsulting.lsd.distributed.access.model.InterceptedInteraction
import io.lsdconsulting.lsd.distributed.access.repository.InterceptedDocumentRepository
import io.lsdconsulting.lsd.distributed.http.config.log
import org.apache.http.HttpResponse
import org.apache.http.HttpStatus.SC_OK
import org.apache.http.client.fluent.Request
import org.apache.http.entity.ContentType.APPLICATION_JSON
import java.io.BufferedReader
import java.net.SocketTimeoutException
class InterceptedDocumentHttpRepository(
connectionString: String,
private val connectionTimeout: Int,
private val objectMapper: ObjectMapper
) : InterceptedDocumentRepository {
private val uri = "$connectionString/lsds"
override fun save(interceptedInteraction: InterceptedInteraction) {
val response: HttpResponse
try {
response = Request.Post(uri)
.connectTimeout(connectionTimeout)
.socketTimeout(connectionTimeout)
.bodyString(objectMapper.writeValueAsString(interceptedInteraction), APPLICATION_JSON)
.execute()
.returnResponse()
} catch (e: SocketTimeoutException) {
log().warn("Connection to $uri timed out. Dropping interceptedInteraction: $interceptedInteraction")
return
}
val statusCode = response.statusLine.statusCode
if (statusCode != SC_OK) {
log().warn("Unable to call $uri - received: $statusCode. Lost interceptedInteraction: $interceptedInteraction")
}
}
override fun findByTraceIds(vararg traceId: String): List {
val response: HttpResponse
try {
response = Request.Get(uri + "?traceIds=" + traceId.joinToString(separator = "&traceIds="))
.connectTimeout(connectionTimeout)
.socketTimeout(connectionTimeout)
.execute()
.returnResponse()
} catch (e: SocketTimeoutException) {
log().warn("Connection to $uri timed out.")
throw e
}
val statusCode = response.statusLine.statusCode
if (statusCode != SC_OK) {
log().warn("Unable to call $uri - received: $statusCode.")
}
val use = response.entity.content.bufferedReader().use(BufferedReader::readText)
return objectMapper.readValue(use, object: TypeReference>(){})
}
override fun isActive() = true
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy