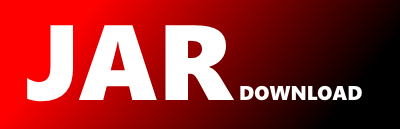
commonMain.dev.icerock.moko.web3.contract.SmartContract.kt Maven / Gradle / Ivy
/*
* Copyright 2020 IceRock MAG Inc. Use of this source code is governed by the Apache 2.0 license.
*/
package dev.icerock.moko.web3.contract
import dev.icerock.moko.web3.BlockHash
import dev.icerock.moko.web3.BlockState
import dev.icerock.moko.web3.ContractAddress
import dev.icerock.moko.web3.Web3Executor
import dev.icerock.moko.web3.contract.ABIEncoder.encodeCallDataForMethod
import dev.icerock.moko.web3.hex.Hex32String
import dev.icerock.moko.web3.hex.HexString
import dev.icerock.moko.web3.requests.CallRpcRequest
import dev.icerock.moko.web3.requests.Web3Requests
import dev.icerock.moko.web3.requests.executeBatch
import dev.icerock.moko.web3.requests.getLogs
import kotlinx.serialization.DeserializationStrategy
import kotlinx.serialization.descriptors.PrimitiveKind
import kotlinx.serialization.descriptors.PrimitiveSerialDescriptor
import kotlinx.serialization.descriptors.SerialDescriptor
import kotlinx.serialization.encoding.Decoder
import kotlinx.serialization.json.JsonArray
class SmartContract(
val executor: Web3Executor,
val contractAddress: ContractAddress,
val abiJson: JsonArray
) {
@Suppress("UNCHECKED_CAST")
private fun makeAbiDeserializer(method: String, mapper: (List) -> T): DeserializationStrategy =
object : DeserializationStrategy {
override val descriptor: SerialDescriptor = PrimitiveSerialDescriptor(
serialName = "StringAbiPrimitiveDeserializer",
kind = PrimitiveKind.STRING
)
override fun deserialize(decoder: Decoder): T {
val abiResult = HexString(decoder.decodeString())
return ABIDecoder.decodeCallDataForOutputs(abiJson, method, abiResult).let(mapper)
}
}
fun readRequest(
method: String,
params: List,
mapper: (List) -> T
): CallRpcRequest {
val data = encodeMethod(method, params)
return Web3Requests.call(contractAddress, data, makeAbiDeserializer(method, mapper))
}
fun encodeMethod(
method: String,
params: List
): HexString = encodeCallDataForMethod(abiJson, method, params)
suspend fun read(
method: String,
params: List,
mapper: (List) -> T
): T = executor.executeBatch(readRequest(method, params, mapper)).first()
fun getLogsRequest(
fromBlock: BlockState? = null,
toBlock: BlockState? = null,
topics: List? = null,
blockHash: BlockHash? = null
) = Web3Requests.getLogs(contractAddress, fromBlock, toBlock, topics, blockHash)
suspend fun getLogs(
fromBlock: BlockState? = null,
toBlock: BlockState? = null,
topics: List? = null,
blockHash: BlockHash? = null
) = executor.getLogs(contractAddress, fromBlock, toBlock, topics, blockHash)
fun hashEventSignature(event: String): Hex32String = ABIEncoder.hashEventSignature(abiJson, event)
// fun writeRequest(
// method: String,
// params: List,
// from: WalletAddress? = null,
// value: BigInteger?
// ): Web3RpcRequest {
// TODO("For future releases")
// }
//
// @Web3Stub
// suspend fun write(
// method: String,
// params: List,
// from: WalletAddress? = null,
// value: BigInteger?
// ): TransactionHash = executor.executeBatch(writeRequest(method, params, from, value)).first()
//
// @Web3Stub
// fun signTransaction(data: JsonElement): String {
// TODO("For future release")
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy