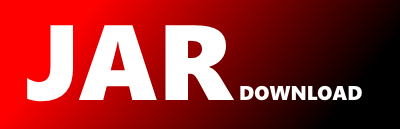
org.infinispan.persistence.redis.compression.LzfCompressor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-cachestore-redis
Show all versions of infinispan-cachestore-redis
Infinispan CacheStore using Redis
package org.infinispan.persistence.redis.compression;
import com.ning.compress.lzf.LZFInputStream;
import com.ning.compress.lzf.LZFOutputStream;
import org.infinispan.marshall.persistence.PersistenceMarshaller;
import org.infinispan.persistence.spi.PersistenceException;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class LzfCompressor implements Compressor {
private final PersistenceMarshaller marshaller;
public LzfCompressor(PersistenceMarshaller marshaller) {
this.marshaller = marshaller;
}
@Override
public byte[] compress(Object entry) {
ByteArrayOutputStream out = new ByteArrayOutputStream();
try (LZFOutputStream lzfStream = new LZFOutputStream(out).setFinishBlockOnFlush(true)) {
marshaller.writeObject(entry, lzfStream);
} catch (IOException e) {
throw new PersistenceException(e);
}
return out.toByteArray();
}
@Override
@SuppressWarnings("unchecked")
public E uncompress(byte[] bytes) {
if (bytes == null) {
return null;
}
try (ByteArrayInputStream byteStream = new ByteArrayInputStream(bytes);
LZFInputStream lzfStream = new LZFInputStream(byteStream)) {
return (E) marshaller.readObject(lzfStream);
} catch (IOException | ClassNotFoundException e) {
throw new PersistenceException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy