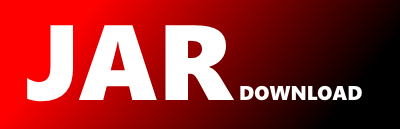
com.ly.invoker.builder.compiler.Context Maven / Gradle / Ivy
package com.ly.invoker.builder.compiler;
import com.ly.invoker.builder.constant.ChainType;
import com.ly.invoker.core.handler.HandlerFunction;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
public class Context {
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy