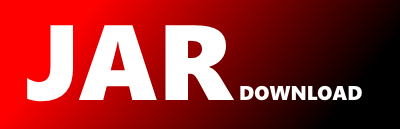
com.ly.invoker.builder.template.Template Maven / Gradle / Ivy
package com.ly.invoker.builder.template;
import com.ly.invoker.builder.constant.ChainType;
public class Template {
protected StringBuilder structure = new StringBuilder();
protected boolean lock = false;
public static Template from(String description) {
Template template = new Template();
template.structure.append(description);
return template;
}
public Template name() {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
structure.append(ChainType.NAME_ROUTER.getValue());
return this;
}
public Template version() {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
structure.append(ChainType.VERSION_ROUTER.getValue());
return this;
}
public Template handler() {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
structure.append(ChainType.HANDLER.getValue());
return this;
}
public Template next(Template template, int n) {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
lock = true;
template = template.clone();
if (n > 0) {
for (int i = 0; i < n; i++) {
structure.append(ChainType.LP.getValue())
.append(template.structure())
.append(ChainType.RP.getValue());
}
} else {
structure.append(ChainType.LP.getValue())
.append(template.structure())
.append(ChainType.RP.getValue())
.append(ChainType.INFINITE.getValue());
}
return this;
}
public Template name(int n) {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
if (n > 0) {
for (int i = 0; i < n; i++) {
structure.append(ChainType.NAME_ROUTER.getValue());
}
} else {
structure.append(ChainType.NAME_ROUTER.getValue()).append(ChainType.INFINITE.getValue());
}
return this;
}
public Template version(int n) {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
if (n > 0) {
for (int i = 0; i < n; i++) {
structure.append(ChainType.VERSION_ROUTER.getValue());
}
} else {
structure.append(ChainType.VERSION_ROUTER.getValue()).append(ChainType.INFINITE.getValue());
}
return this;
}
public Template handler(int n) {
if (lock) {
throw new RuntimeException("已生成的模板不可修改");
}
if (n > 0) {
for (int i = 0; i < n; i++) {
structure.append(ChainType.HANDLER.getValue());
}
} else {
structure.append(ChainType.HANDLER.getValue()).append(ChainType.INFINITE.getValue());
}
return this;
}
public StringBuilder structure() {
return structure;
}
public Template clear() {
structure = new StringBuilder();
lock = false;
return this;
}
public Template clone() {
Template template = new Template();
template.structure.append(structure);
return template;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy