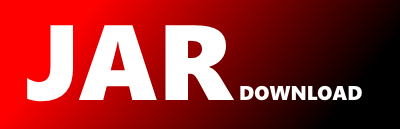
com.ly.mybatis.mapperservice.service.join.inject.Inject Maven / Gradle / Ivy
The newest version!
package com.ly.mybatis.mapperservice.service.join.inject;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.ly.mybatis.mapperservice.model.Result;
import com.ly.mybatis.mapperservice.util.MPSUtil;
import lombok.extern.slf4j.Slf4j;
import java.util.*;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
@Slf4j
public class Inject {
protected Class from;
protected Class join;
protected Class> searchClass;
protected MapStream key;
protected MapStream on;
protected String column;
protected Function extends Collection>, ? extends Collection> search;
protected InjectStream set;
protected Inject sub;
protected List> next = new ArrayList<>();
public Inject from(Class from) {
this.from = from;
return this;
}
public Inject join(Class join) {
this.join = join;
return this;
}
public Inject key(Consumer key) {
if (this.key == null) {
this.key = new MapStream();
}
key.accept(this.key);
return this;
}
public Inject on(Consumer on) {
if (this.on == null) {
this.on = new MapStream();
}
on.accept(this.on);
return this;
}
public Inject column(Class> searchClass, String on) {
this.searchClass = searchClass;
column = on;
return this;
}
public , O extends Collection> Inject search(Function search) {
this.search = search;
return this;
}
public Inject set(Consumer set) {
if (this.set == null) {
this.set = new InjectStream();
}
set.accept(this.set);
return this;
}
public Inject sub() {
Inject sub = new Inject<>();
sub.from(this.join);
this.sub = sub;
return sub;
}
public Inject next() {
Inject sub = new Inject<>();
sub.from(this.join);
this.next.add(sub);
return sub;
}
public List process(List models) {
List ms = key.construct(models);
if (ms.isEmpty()) {
return new ArrayList<>();
}
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy