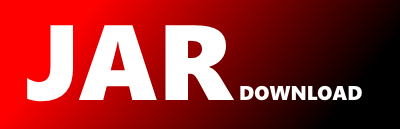
com.ly.mybatis.mapperservice.service.join.inject.InjectJoin Maven / Gradle / Ivy
package com.ly.mybatis.mapperservice.service.join.inject;
import cn.hutool.core.exceptions.ExceptionUtil;
import com.baomidou.mybatisplus.core.toolkit.support.SFunction;
import com.ly.mybatis.mapperservice.service.join.inject.interfaces.*;
import com.ly.mybatis.mapperservice.table.MyBatisTableUtil;
import lombok.extern.slf4j.Slf4j;
import java.util.Collection;
import java.util.List;
import java.util.function.*;
import java.util.stream.Collector;
@SuppressWarnings("all")
@Slf4j
public class InjectJoin implements IProcessInject {
private final Inject root = new Inject<>();
private Inject builder = root;
private Inject last;
private From from = new From();
private Join join = new Join();
private Key key = new Key();
private On on = new On();
private Set set = new Set();
public static IKeyInject create(Class from, Class join) {
InjectJoin injectJoin = new InjectJoin<>();
return injectJoin.from.from(from)
.join(join);
}
class From implements IFromInject {
@Override
public IJoinInject from(Class from) {
builder = ((Inject) builder).from(from);
return join;
}
}
class Join implements IJoinInject {
@Override
public IKeyInject join(Class join) {
builder = ((Inject) builder).join(join);
return (IKeyInject) key;
}
}
class Key implements IKeyInject {
@Override
public IKeyInject filter(Predicate super K> predicate) {
builder = ((Inject) builder).key(mapStream -> mapStream.filter(predicate));
return this;
}
@Override
public IKeyInject map(Function super K, ? extends R> mapper) {
builder = ((Inject) builder).key(mapStream -> mapStream.map(mapper));
return (IKeyInject) this;
}
@Override
public IKeyInject flatMap(Function super K, ? extends Collection extends R>> mapper) {
builder = ((Inject) builder).key(mapStream -> mapStream.flatMap(mapper));
return (IKeyInject) this;
}
@Override
public IOnInject on() {
return (IOnInject) on;
}
}
class On implements IOnInject {
@Override
public IProcessInject build() {
return InjectJoin.this;
}
@Override
public IOnInject filter(Predicate super O> predicate) {
builder = ((Inject) builder).on(mapStream -> mapStream.filter(predicate));
return this;
}
@Override
public IOnInject map(Function super O, ? extends R> mapper) {
builder = ((Inject) builder).on(mapStream -> mapStream.map(mapper));
return (IOnInject) this;
}
@Override
public IOnInject mapColumn(SFunction super O, ? extends R> mapper) {
builder = ((Inject) builder).on(mapStream -> mapStream.map(mapper))
.column(
null,
MyBatisTableUtil.getColumnBySFunction((SFunction, ?>) mapper)
);
return (IOnInject) this;
}
@Override
public IOnInject flatMap(Function super O, ? extends Collection extends R>> mapper) {
builder = ((Inject) builder).on(mapStream -> mapStream.flatMap(mapper));
return (IOnInject) this;
}
@Override
public IOnInject column(Class tClass, SFunction column) {
builder = ((Inject) builder).column(tClass, MyBatisTableUtil.getColumnBySFunction(column));
return this;
}
@Override
public IOnInject column(Class tClass, String column) {
builder = ((Inject) builder).column(tClass, column);
return this;
}
@Override
public ISetInject search(Function, ? extends Collection> search) {
builder = ((Inject) builder).search(search);
return (ISetInject) set;
}
@Override
public ISetInject set() {
return (ISetInject) set;
}
@Override
public IKeyInject subJoin(Class join) {
return InjectJoin.this.subJoin(join);
}
@Override
public IKeyInject join(Class join) {
return InjectJoin.this.join(join);
}
}
class Set implements ISetInject {
@Override
public IProcessInject build() {
return InjectJoin.this;
}
@Override
public IKeyInject join(Class join) {
return InjectJoin.this.join(join);
}
@Override
public ISetInject filter(Predicate super S> predicate) {
builder = ((Inject) builder).set(injectStream -> injectStream.filter(predicate));
return this;
}
@Override
public ISetInject map(Function super S, ? extends R> mapper) {
builder = ((Inject) builder).set(injectStream -> injectStream.map(mapper));
return (ISetInject) this;
}
@Override
public ISetInject flatMap(Function super S, ? extends Collection extends R>> mapper) {
builder = ((Inject) builder).set(injectStream -> injectStream.flatMap(mapper));
return (ISetInject) this;
}
@Override
public ISetInject collect(Collector super S, ?, ? extends R> collector) {
builder = ((Inject) builder).set(injectStream -> injectStream.collect(collector));
return (ISetInject) this;
}
@Override
public ISetInject set(BiConsumer super V, ? extends S> set) {
builder = ((Inject) builder).set(injectStream -> injectStream.set(set));
return this;
}
@Override
public IKeyInject subJoin(Class join) {
return InjectJoin.this.subJoin(join);
}
}
protected IKeyInject subJoin(Class join) {
if (last != null) {
builder = last.sub;
}
last = builder;
builder = ((Inject) builder)
.sub()
.join(join);
return (IKeyInject) key;
}
protected IKeyInject join(Class join) {
builder = ((Inject, F>) last)
.next()
.join(join);
return (IKeyInject) key;
}
@Override
public void process(Collection vs) {
if (vs == null || vs.isEmpty()) {
return;
}
List models = Model.of(vs);
Inject current = root;
if (current != null) {
models = root.process(models);
if (models.isEmpty()) {
log.warn("empty results:{}", ExceptionUtil.getStackElement(3).toString());
return;
}
while (current.sub != null) {
List process = current.sub.process(models);
if (process.isEmpty()) {
log.warn("empty results:{}", ExceptionUtil.getStackElement(3).toString());
break;
}
for (Object o : current.next) {
Inject n = (Inject) o;
n.process(models);
}
models = process;
current = current.sub;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy