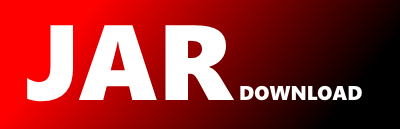
com.ly.mybatis.mapperservice.service.join.inject.MapStream Maven / Gradle / Ivy
package com.ly.mybatis.mapperservice.service.join.inject;
import java.util.*;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class MapStream {
private final Queue queue = new LinkedList<>();
public MapStream filter(Predicate super T> predicate) {
queue.add(Operation.of(1, predicate));
return this;
}
public MapStream map(Function super T, ? extends R> mapper) {
queue.add(Operation.of(2, mapper));
return this;
}
public MapStream flatMap(Function super T, ? extends Collection extends R>> mapper) {
queue.add(Operation.of(3, mapper));
return this;
}
public List construct(Collection models) {
Stream s = models.parallelStream()
.map(Model::copy);
for (Operation operation : queue) {
switch (operation.getType()) {
case 1:
s = s.filter(m -> {
try {
return operation.>getOperation().test(m.join());
} catch (Exception e) {
e.printStackTrace();
return false;
}
});
break;
case 2:
s = s.peek(m -> {
try {
m.join(operation.>getOperation()
.apply(m.join()));
} catch (Exception e) {
e.printStackTrace();
}
});
break;
default:
s = s.flatMap(m -> {
try {
Collection> cs = operation.>>getOperation()
.apply(m.join());
if (cs == null) {
return Stream.empty();
} else {
return cs.parallelStream()
.filter(Objects::nonNull)
.map(j -> {
try {
return m.copy()
.join(j);
} catch (Exception e) {
e.printStackTrace();
return null;
}
})
.filter(Objects::nonNull);
}
} catch (Exception e) {
e.printStackTrace();
return Stream.empty();
}
});
}
}
return s.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy