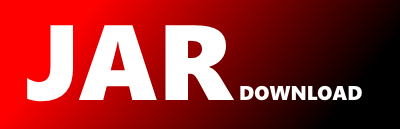
com.ly.mybatis.mapperservice.service.join.inject.Model Maven / Gradle / Ivy
The newest version!
package com.ly.mybatis.mapperservice.service.join.inject;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Model {
private Object source;
private Object from;
private Object join;
private Integer sourceId;
private Integer fromId;
private Integer joinId;
public static List of(Collection ts) {
return ts.parallelStream()
.map(t -> new Model().source(t).from(t).join(t))
.collect(Collectors.toList());
}
public static List merge(Collection merge, Collection by) {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy